First C# RPG game and project as a beginner
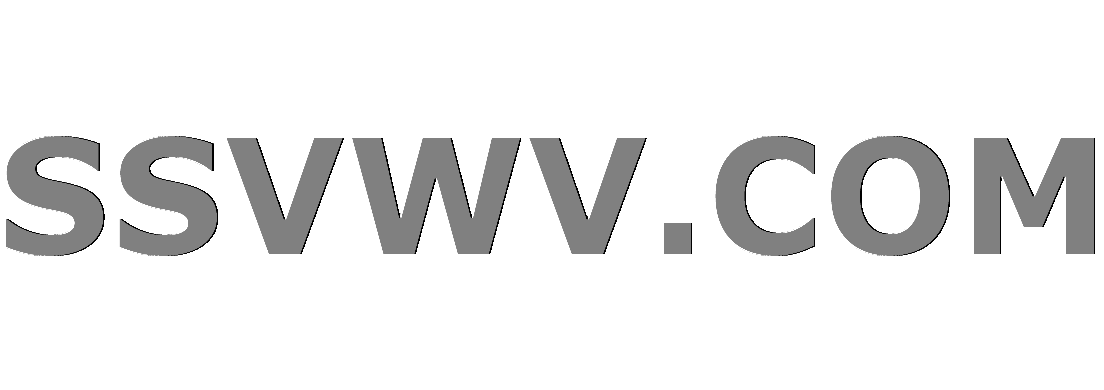
Multi tool use
Guys I wanted your opinion on the first RPG game I made in C#, what things can I improve on with the code and suggestions about more advanced projects that I should work on in the future.
Events:
static class Events
{
public static void SpawnWeapon(string weapon_name)
{
Console.WriteLine("nAfter that fight you looted a weapon from the enemy party: {0}", weapon_name);
Player.EquipWeapon(weapon_name);
}
public static void LootHerbs(Player player)
{
Console.WriteLine("nYou also managed to loot some herbs that you will use to cure you! You gained 3 HP.");
player.HP += 3;
}
public static void LootSpecialHerbs(Player player)
{
Console.WriteLine("nYou also managed to loot some special herbs that you will use to cure you! You gained 5 HP.");
player.HP += 5;
}
public static void LootMedicine(Player player)
{
Console.WriteLine("nYou looted some medicine that you will use to cure you! You gained 5 HP.");
player.HP += 5;
}
public static void LootHighGradeMedicine(Player player)
{
Console.WriteLine("nAnd you also managed to loot some high grade medicine that you will use to cure you! You gained 7 HP.");
player.HP += 7;
}
public static void GodBlessing(Player player)
{
Console.WriteLine("nAs you were walking towards the dragon nest, you suddenly hear a angelical voice behind youn" +
"and your wounds magically heal. You were blessed by a god to finish your journey! You gained 12 HP.");
player.HP += 12;
}
}
Battle:
static class Battle
{
public static int crit_chance = 16; // 1 in 15 attacks is a crit. ( Yes, 15 ).
public static int attack_timer = 1500;
public static bool isCrit()
{
Random rnd = new Random();
int crit = rnd.Next(1, crit_chance);
if (crit == 1)
{
return true;
}
return false;
}
public static bool isPlayerDead(Player player)
{
if (player.HP <= 0)
{
return true;
}
return false;
}
public static bool isMobDead(Mob mob)
{
if (mob.HP <= 0)
{
return true;
}
return false;
}
public static void PlayerAttack(Player player, Mob mob)
{
bool was_crit = false;
int DamageInflicted()
{
int damage = player.Attack() - mob.Defense();
if (damage <= 1)
{
damage = 1;
}
if (isCrit() == true)
{
was_crit = true;
damage += damage;
}
return damage;
}
mob.HP -= DamageInflicted();
if (was_crit == true)
{
Console.WriteLine("It was a critical hit! You dealt {0} damage.", DamageInflicted());
}
else
{
Console.WriteLine("You dealt {0} damage.", DamageInflicted());
}
Console.WriteLine("The enemy has {0} HP.", mob.HP);
}
public static void EnemyAttack(Player player, Mob mob)
{
bool was_crit = false;
int DamageInflicted()
{
int damage = mob.Attack() - player.Defense();
if (damage <= 0)
{
damage = 0;
}
if (isCrit() == true)
{
was_crit = true;
damage += damage;
}
return damage;
}
player.HP -= DamageInflicted();
if (was_crit == true)
{
Console.WriteLine("It was a critical hit! The enemy dealt {0} damage.", DamageInflicted());
}
else
{
Console.WriteLine("The enemy dealt {0} damage.", DamageInflicted());
}
Console.WriteLine("You have {0} HP.", player.HP);
}
public static void MakeBattle(Player player, Mob mob)
{
Console.WriteLine("You, {0}, will now fight the {1} {2}.", player.name, mob.specie, mob.name);
mob.Stats();
Console.WriteLine("Press Enter to begin the fight.");
Console.ReadLine();
Random rnd = new Random();
int move = rnd.Next(1, 3);
if (move == 1)
{
Console.WriteLine("You begin!");
while (true)
{
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
PlayerAttack(player, mob);
if (isMobDead(mob))
{
Console.WriteLine("You killed the {0}!", mob.specie);
Console.ReadLine();
Console.Clear();
break;
}
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
EnemyAttack(player, mob);
if (isPlayerDead(player))
{
Console.WriteLine("You died! Game Over!");
break;
}
}
}
else
{
Console.WriteLine("The enemy will begin!");
while (true)
{
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
EnemyAttack(player, mob);
if (isPlayerDead(player))
{
Console.WriteLine("You died! Game Over!");
break;
}
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
PlayerAttack(player, mob);
if (isMobDead(mob))
{
Console.WriteLine("You killed the {0}!", mob.specie);
Console.ReadLine();
Console.Clear();
break;
}
}
}
}
}
Mob:
class Mob
{
public string specie;
public string name;
public int base_attack = 2;
public int base_defense = 1;
public int HP;
public int mob_defense;
public int mob_attack;
public Mob(string aSpecie, string aName, int aHP, int aAttack, int aDefense)
{
specie = aSpecie;
name = aName;
HP = aHP;
mob_attack = aAttack;
mob_defense = aDefense;
}
public int Attack()
{
return (base_attack + mob_attack);
}
public int Defense()
{
return (base_defense + mob_defense);
}
public void Stats()
{
Console.WriteLine("The {0} {1}'s Stats:", specie, name);
Console.WriteLine("HP: {0}", HP);
Console.WriteLine("Attack: {0}", Attack());
Console.WriteLine("Defense: {0}", Defense());
}
}
Story:
static class Story
{
public static void S_1_Bandits(Player player)
{
Console.WriteLine("You are " + player.name + ", who's on his way to kill the dragons which are destroying the kingdom.");
Console.WriteLine("As you are on your way to the lairs of the dragons, you run into a couple of bandits.");
Console.WriteLine("And they don't seem so friendly...");
Console.ReadLine();
Console.Clear();
}
public static void S_2_Knights()
{
Console.WriteLine("The bandits weren't much of a match for you. Well Done! You continue on to the dragons lair!");
Console.WriteLine("However, a new movement has risen that wants to protect the dragons of the world.");
Console.WriteLine("Many people have joined this movement, including some knights.");
Console.WriteLine("And uh oh, 3 of them have found out about your quest...");
Console.WriteLine("Maybe they're friendly?");
Console.ReadLine();
Console.WriteLine("Nope.");
Console.ReadLine();
Console.Clear();
}
public static void S_3_Dragons()
{
Console.WriteLine("With the knights defeated you continue on your journey!");
Console.WriteLine("After a while you make it to the lair of dragons...");
Console.WriteLine("It's hot and little smokey in there. You put your sight on the pair of dragons in the center of it.");
Console.WriteLine("The time has come to end the dragons rampage!");
Console.ReadLine();
Console.Clear();
}
public static void S_End(Player player)
{
Console.WriteLine("You killed the dragons and saved the kingdom!");
Console.WriteLine("Your name will be remembered forever and legends will be told about the hero {0} that defeated the evil dragons!", player.name);
Console.WriteLine("Congrats!");
}
}
Weapon:
class Weapon
{
public string name;
public int attack;
public int defense;
public static List<Weapon> weapon_list = new List<Weapon>();
public Weapon(string aName, int aAttack, int aDefense)
{
name = aName;
attack = aAttack;
defense = aDefense;
}
public static void CreateWeapon( string name, int attack, int defense)
{
Weapon weapon = new Weapon(name, attack, defense);
weapon_list.Add(weapon);
}
public static void CheckAllAvailableWeaponsStats()
{
Console.WriteLine("nAll weapons in the game:");
foreach (Weapon weapon in Weapon.weapon_list)
{
Console.Write("Name: {0}nAttack: {1}nDefense: {2}n", weapon.name, weapon.attack, weapon.defense);
Console.WriteLine("---------------------------");
}
}
public static void CheckWeaponStats(Weapon weapon)
{
Console.Write("nName: {0}nAttack: {1}nDefense: {2}n", weapon.name, weapon.attack, weapon.defense);
}
public static void CompareWeaponStats(Weapon other_weapon, Weapon your_weapon)
{
if (your_weapon == Player.equipped_weapon)
{
Console.Write("Name: {0} | Equipped Weapon Name: {1}nAttack: {2} | Equipped Weapon Attack: {3} nDefense: {4} |" +
" Equipped Weapon Defense: {5}n", other_weapon.name, your_weapon.name, other_weapon.attack, your_weapon.attack, other_weapon.defense, your_weapon.defense);
}
else
{
Console.Write("Other Weapon Name: {0} | Your Weapon Name: {1}nOther Weapon Attack: {2} | Your Weapon Attack: {3} nOther Weapon Defense: {4} " +
"| Your Weapon Defense: {5}n", other_weapon.name, your_weapon.name, other_weapon.attack, your_weapon.attack, other_weapon.defense, your_weapon.defense);
}
}
}
Player:
class Player
{
public static Weapon initial_sword = new Weapon("Initial Sword", 8, 4);
public string name;
public int HP;
public int level = 0;
public static Weapon equipped_weapon = initial_sword;
public int base_attack = 4;
public int base_defense = 2;
public Player(string aName, int aHP)
{
name = aName;
HP = aHP;
}
public int Attack()
{
return (base_attack + equipped_weapon.attack);
}
public int Defense()
{
return (base_defense + equipped_weapon.defense);
}
public void Stats()
{
Console.WriteLine("n{0}'s Stats:", name);
Console.WriteLine("HP: {0}", HP);
Console.WriteLine("Attack: {0} ({1})", Attack(), base_attack);
Console.WriteLine("Defense: {0} ({1})", Defense(), base_defense);
Console.WriteLine("Equipped Weapon: {0}; AT: {1}; DF: {2}", equipped_weapon.name, equipped_weapon.attack, equipped_weapon.defense);
}
public static bool QuestionPrompt()
{
string yes_list = { "yes", "", "sure", "y", "yeah", "why not", "yes.", "y." };
Console.Write("-->");
string input = Console.ReadLine();
string iinput = input.ToLower();
foreach (string value in yes_list)
{
if (value.Equals(iinput))
{
return true;
}
else
{
continue;
}
}
return false;
}
public static void ChangeWeaponByName(string new_weapon_name)
{
Weapon weapon_to_change = new Weapon("Test Weapon", 0, 0);
bool WeaponExists()
{
foreach (Weapon weapon in Weapon.weapon_list)
{
if (weapon.name.ToLower() == new_weapon_name.ToLower())
{
weapon_to_change = weapon;
return true;
}
else
{
continue;
}
}
return false;
}
if (WeaponExists() == true)
{
equipped_weapon = weapon_to_change;
}
}
public static void ChangeWeapon(Weapon new_weapon)
{
equipped_weapon = new_weapon;
}
public static void EquipWeapon(string weapon_name)
{
Weapon weapon_to_equip = new Weapon("Test Weapon", 0, 0);
bool WeaponExists()
{
foreach (Weapon weapon in Weapon.weapon_list)
{
if (weapon.name.ToLower() == weapon_name.ToLower())
{
weapon_to_equip = weapon;
return true;
}
else
{
continue;
}
}
return false;
}
if (WeaponExists())
{
Console.WriteLine("nComparison of both weapons stats:");
Weapon.CompareWeaponStats(weapon_to_equip, Player.equipped_weapon);
Console.WriteLine("Are you sure you want to equip this weapon?");
if (QuestionPrompt() == true)
{
Console.WriteLine("You equipped the weapon!");
ChangeWeapon(weapon_to_equip);
}
else
{
Console.WriteLine("You will continue with the same weapon, the new one was discarded.");
}
}
else
{
Console.WriteLine("The weapon you want to equip doesn't exist!");
}
}
public static void CheckEquippedWeapon()
{
Console.WriteLine("Equipped Weapon:");
Weapon.CheckWeaponStats(equipped_weapon);
}
}
Main:
static void Main()
{
Player player = new Player("", 30);
bool PlayerDied()
{
if (Battle.isPlayerDead(player) == true)
{
return true;
}
return false;
}
Weapon.CreateWeapon("Rusty Old Sword", 7, 3); // initial weapon
Weapon.CreateWeapon("Iron Sword", 10, 5); // to give the player after he fights the bandits
Weapon.CreateWeapon("Enchanted Silver Sword", 15, 7); // to give the player after he fights the knights
Player.ChangeWeaponByName("rusty old sword");
// the enemies
Mob bandits =
{
new Mob("Bandit", "Rob", 10, 6, 2),
new Mob("Bandit Leader", "Joe", 10, 7, 2)
};
Mob knights =
{
new Mob("Knight", "Rob", 12, 8, 4),
new Mob("Knight", "John", 12, 9, 3),
new Mob("Knight Captain", "Aaron", 14, 10, 4),
};
Mob dragons =
{
new Mob("Blue Dragon", "Jormungandr", 16, 10, 6),
new Mob("Dragon Leader", "Helios", 18, 11, 6),
};
Console.WriteLine("What's your name, adventurer?");
string response = Console.ReadLine();
player.name = response;
player.Stats();
Console.WriteLine("nPress anything to begin the game.");
Console.ReadLine();
Console.Clear();
while (true)
{
Story.S_1_Bandits(player); // first part of the story
foreach (Mob mob in bandits)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Events.SpawnWeapon("Iron Sword");
if (player.HP <= 16)
{
Events.LootSpecialHerbs(player);
}
else
{
Events.LootHerbs(player);
}
player.Stats();
Console.WriteLine("nPress Enter to continue.");
Console.ReadLine();
Console.Clear();
Story.S_2_Knights(); // second part of the story
foreach (Mob mob in knights)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Events.SpawnWeapon("Enchanted Silver Sword");
Events.LootMedicine(player);
if (player.HP >= 15)
{
Events.LootHighGradeMedicine(player);
}
else if (player.HP <= 13)
{
Events.GodBlessing(player);
}
else
{
Events.LootMedicine(player);
}
player.Stats();
Console.WriteLine("nPress Enter to continue.");
Console.ReadLine();
Console.Clear();
Story.S_3_Dragons(); // third part of the story
foreach (Mob mob in dragons)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Console.Clear();
Story.S_End(player);
break;
}
Console.ReadLine();
}
}
c#
New contributor
Cavalex is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
Guys I wanted your opinion on the first RPG game I made in C#, what things can I improve on with the code and suggestions about more advanced projects that I should work on in the future.
Events:
static class Events
{
public static void SpawnWeapon(string weapon_name)
{
Console.WriteLine("nAfter that fight you looted a weapon from the enemy party: {0}", weapon_name);
Player.EquipWeapon(weapon_name);
}
public static void LootHerbs(Player player)
{
Console.WriteLine("nYou also managed to loot some herbs that you will use to cure you! You gained 3 HP.");
player.HP += 3;
}
public static void LootSpecialHerbs(Player player)
{
Console.WriteLine("nYou also managed to loot some special herbs that you will use to cure you! You gained 5 HP.");
player.HP += 5;
}
public static void LootMedicine(Player player)
{
Console.WriteLine("nYou looted some medicine that you will use to cure you! You gained 5 HP.");
player.HP += 5;
}
public static void LootHighGradeMedicine(Player player)
{
Console.WriteLine("nAnd you also managed to loot some high grade medicine that you will use to cure you! You gained 7 HP.");
player.HP += 7;
}
public static void GodBlessing(Player player)
{
Console.WriteLine("nAs you were walking towards the dragon nest, you suddenly hear a angelical voice behind youn" +
"and your wounds magically heal. You were blessed by a god to finish your journey! You gained 12 HP.");
player.HP += 12;
}
}
Battle:
static class Battle
{
public static int crit_chance = 16; // 1 in 15 attacks is a crit. ( Yes, 15 ).
public static int attack_timer = 1500;
public static bool isCrit()
{
Random rnd = new Random();
int crit = rnd.Next(1, crit_chance);
if (crit == 1)
{
return true;
}
return false;
}
public static bool isPlayerDead(Player player)
{
if (player.HP <= 0)
{
return true;
}
return false;
}
public static bool isMobDead(Mob mob)
{
if (mob.HP <= 0)
{
return true;
}
return false;
}
public static void PlayerAttack(Player player, Mob mob)
{
bool was_crit = false;
int DamageInflicted()
{
int damage = player.Attack() - mob.Defense();
if (damage <= 1)
{
damage = 1;
}
if (isCrit() == true)
{
was_crit = true;
damage += damage;
}
return damage;
}
mob.HP -= DamageInflicted();
if (was_crit == true)
{
Console.WriteLine("It was a critical hit! You dealt {0} damage.", DamageInflicted());
}
else
{
Console.WriteLine("You dealt {0} damage.", DamageInflicted());
}
Console.WriteLine("The enemy has {0} HP.", mob.HP);
}
public static void EnemyAttack(Player player, Mob mob)
{
bool was_crit = false;
int DamageInflicted()
{
int damage = mob.Attack() - player.Defense();
if (damage <= 0)
{
damage = 0;
}
if (isCrit() == true)
{
was_crit = true;
damage += damage;
}
return damage;
}
player.HP -= DamageInflicted();
if (was_crit == true)
{
Console.WriteLine("It was a critical hit! The enemy dealt {0} damage.", DamageInflicted());
}
else
{
Console.WriteLine("The enemy dealt {0} damage.", DamageInflicted());
}
Console.WriteLine("You have {0} HP.", player.HP);
}
public static void MakeBattle(Player player, Mob mob)
{
Console.WriteLine("You, {0}, will now fight the {1} {2}.", player.name, mob.specie, mob.name);
mob.Stats();
Console.WriteLine("Press Enter to begin the fight.");
Console.ReadLine();
Random rnd = new Random();
int move = rnd.Next(1, 3);
if (move == 1)
{
Console.WriteLine("You begin!");
while (true)
{
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
PlayerAttack(player, mob);
if (isMobDead(mob))
{
Console.WriteLine("You killed the {0}!", mob.specie);
Console.ReadLine();
Console.Clear();
break;
}
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
EnemyAttack(player, mob);
if (isPlayerDead(player))
{
Console.WriteLine("You died! Game Over!");
break;
}
}
}
else
{
Console.WriteLine("The enemy will begin!");
while (true)
{
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
EnemyAttack(player, mob);
if (isPlayerDead(player))
{
Console.WriteLine("You died! Game Over!");
break;
}
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
PlayerAttack(player, mob);
if (isMobDead(mob))
{
Console.WriteLine("You killed the {0}!", mob.specie);
Console.ReadLine();
Console.Clear();
break;
}
}
}
}
}
Mob:
class Mob
{
public string specie;
public string name;
public int base_attack = 2;
public int base_defense = 1;
public int HP;
public int mob_defense;
public int mob_attack;
public Mob(string aSpecie, string aName, int aHP, int aAttack, int aDefense)
{
specie = aSpecie;
name = aName;
HP = aHP;
mob_attack = aAttack;
mob_defense = aDefense;
}
public int Attack()
{
return (base_attack + mob_attack);
}
public int Defense()
{
return (base_defense + mob_defense);
}
public void Stats()
{
Console.WriteLine("The {0} {1}'s Stats:", specie, name);
Console.WriteLine("HP: {0}", HP);
Console.WriteLine("Attack: {0}", Attack());
Console.WriteLine("Defense: {0}", Defense());
}
}
Story:
static class Story
{
public static void S_1_Bandits(Player player)
{
Console.WriteLine("You are " + player.name + ", who's on his way to kill the dragons which are destroying the kingdom.");
Console.WriteLine("As you are on your way to the lairs of the dragons, you run into a couple of bandits.");
Console.WriteLine("And they don't seem so friendly...");
Console.ReadLine();
Console.Clear();
}
public static void S_2_Knights()
{
Console.WriteLine("The bandits weren't much of a match for you. Well Done! You continue on to the dragons lair!");
Console.WriteLine("However, a new movement has risen that wants to protect the dragons of the world.");
Console.WriteLine("Many people have joined this movement, including some knights.");
Console.WriteLine("And uh oh, 3 of them have found out about your quest...");
Console.WriteLine("Maybe they're friendly?");
Console.ReadLine();
Console.WriteLine("Nope.");
Console.ReadLine();
Console.Clear();
}
public static void S_3_Dragons()
{
Console.WriteLine("With the knights defeated you continue on your journey!");
Console.WriteLine("After a while you make it to the lair of dragons...");
Console.WriteLine("It's hot and little smokey in there. You put your sight on the pair of dragons in the center of it.");
Console.WriteLine("The time has come to end the dragons rampage!");
Console.ReadLine();
Console.Clear();
}
public static void S_End(Player player)
{
Console.WriteLine("You killed the dragons and saved the kingdom!");
Console.WriteLine("Your name will be remembered forever and legends will be told about the hero {0} that defeated the evil dragons!", player.name);
Console.WriteLine("Congrats!");
}
}
Weapon:
class Weapon
{
public string name;
public int attack;
public int defense;
public static List<Weapon> weapon_list = new List<Weapon>();
public Weapon(string aName, int aAttack, int aDefense)
{
name = aName;
attack = aAttack;
defense = aDefense;
}
public static void CreateWeapon( string name, int attack, int defense)
{
Weapon weapon = new Weapon(name, attack, defense);
weapon_list.Add(weapon);
}
public static void CheckAllAvailableWeaponsStats()
{
Console.WriteLine("nAll weapons in the game:");
foreach (Weapon weapon in Weapon.weapon_list)
{
Console.Write("Name: {0}nAttack: {1}nDefense: {2}n", weapon.name, weapon.attack, weapon.defense);
Console.WriteLine("---------------------------");
}
}
public static void CheckWeaponStats(Weapon weapon)
{
Console.Write("nName: {0}nAttack: {1}nDefense: {2}n", weapon.name, weapon.attack, weapon.defense);
}
public static void CompareWeaponStats(Weapon other_weapon, Weapon your_weapon)
{
if (your_weapon == Player.equipped_weapon)
{
Console.Write("Name: {0} | Equipped Weapon Name: {1}nAttack: {2} | Equipped Weapon Attack: {3} nDefense: {4} |" +
" Equipped Weapon Defense: {5}n", other_weapon.name, your_weapon.name, other_weapon.attack, your_weapon.attack, other_weapon.defense, your_weapon.defense);
}
else
{
Console.Write("Other Weapon Name: {0} | Your Weapon Name: {1}nOther Weapon Attack: {2} | Your Weapon Attack: {3} nOther Weapon Defense: {4} " +
"| Your Weapon Defense: {5}n", other_weapon.name, your_weapon.name, other_weapon.attack, your_weapon.attack, other_weapon.defense, your_weapon.defense);
}
}
}
Player:
class Player
{
public static Weapon initial_sword = new Weapon("Initial Sword", 8, 4);
public string name;
public int HP;
public int level = 0;
public static Weapon equipped_weapon = initial_sword;
public int base_attack = 4;
public int base_defense = 2;
public Player(string aName, int aHP)
{
name = aName;
HP = aHP;
}
public int Attack()
{
return (base_attack + equipped_weapon.attack);
}
public int Defense()
{
return (base_defense + equipped_weapon.defense);
}
public void Stats()
{
Console.WriteLine("n{0}'s Stats:", name);
Console.WriteLine("HP: {0}", HP);
Console.WriteLine("Attack: {0} ({1})", Attack(), base_attack);
Console.WriteLine("Defense: {0} ({1})", Defense(), base_defense);
Console.WriteLine("Equipped Weapon: {0}; AT: {1}; DF: {2}", equipped_weapon.name, equipped_weapon.attack, equipped_weapon.defense);
}
public static bool QuestionPrompt()
{
string yes_list = { "yes", "", "sure", "y", "yeah", "why not", "yes.", "y." };
Console.Write("-->");
string input = Console.ReadLine();
string iinput = input.ToLower();
foreach (string value in yes_list)
{
if (value.Equals(iinput))
{
return true;
}
else
{
continue;
}
}
return false;
}
public static void ChangeWeaponByName(string new_weapon_name)
{
Weapon weapon_to_change = new Weapon("Test Weapon", 0, 0);
bool WeaponExists()
{
foreach (Weapon weapon in Weapon.weapon_list)
{
if (weapon.name.ToLower() == new_weapon_name.ToLower())
{
weapon_to_change = weapon;
return true;
}
else
{
continue;
}
}
return false;
}
if (WeaponExists() == true)
{
equipped_weapon = weapon_to_change;
}
}
public static void ChangeWeapon(Weapon new_weapon)
{
equipped_weapon = new_weapon;
}
public static void EquipWeapon(string weapon_name)
{
Weapon weapon_to_equip = new Weapon("Test Weapon", 0, 0);
bool WeaponExists()
{
foreach (Weapon weapon in Weapon.weapon_list)
{
if (weapon.name.ToLower() == weapon_name.ToLower())
{
weapon_to_equip = weapon;
return true;
}
else
{
continue;
}
}
return false;
}
if (WeaponExists())
{
Console.WriteLine("nComparison of both weapons stats:");
Weapon.CompareWeaponStats(weapon_to_equip, Player.equipped_weapon);
Console.WriteLine("Are you sure you want to equip this weapon?");
if (QuestionPrompt() == true)
{
Console.WriteLine("You equipped the weapon!");
ChangeWeapon(weapon_to_equip);
}
else
{
Console.WriteLine("You will continue with the same weapon, the new one was discarded.");
}
}
else
{
Console.WriteLine("The weapon you want to equip doesn't exist!");
}
}
public static void CheckEquippedWeapon()
{
Console.WriteLine("Equipped Weapon:");
Weapon.CheckWeaponStats(equipped_weapon);
}
}
Main:
static void Main()
{
Player player = new Player("", 30);
bool PlayerDied()
{
if (Battle.isPlayerDead(player) == true)
{
return true;
}
return false;
}
Weapon.CreateWeapon("Rusty Old Sword", 7, 3); // initial weapon
Weapon.CreateWeapon("Iron Sword", 10, 5); // to give the player after he fights the bandits
Weapon.CreateWeapon("Enchanted Silver Sword", 15, 7); // to give the player after he fights the knights
Player.ChangeWeaponByName("rusty old sword");
// the enemies
Mob bandits =
{
new Mob("Bandit", "Rob", 10, 6, 2),
new Mob("Bandit Leader", "Joe", 10, 7, 2)
};
Mob knights =
{
new Mob("Knight", "Rob", 12, 8, 4),
new Mob("Knight", "John", 12, 9, 3),
new Mob("Knight Captain", "Aaron", 14, 10, 4),
};
Mob dragons =
{
new Mob("Blue Dragon", "Jormungandr", 16, 10, 6),
new Mob("Dragon Leader", "Helios", 18, 11, 6),
};
Console.WriteLine("What's your name, adventurer?");
string response = Console.ReadLine();
player.name = response;
player.Stats();
Console.WriteLine("nPress anything to begin the game.");
Console.ReadLine();
Console.Clear();
while (true)
{
Story.S_1_Bandits(player); // first part of the story
foreach (Mob mob in bandits)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Events.SpawnWeapon("Iron Sword");
if (player.HP <= 16)
{
Events.LootSpecialHerbs(player);
}
else
{
Events.LootHerbs(player);
}
player.Stats();
Console.WriteLine("nPress Enter to continue.");
Console.ReadLine();
Console.Clear();
Story.S_2_Knights(); // second part of the story
foreach (Mob mob in knights)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Events.SpawnWeapon("Enchanted Silver Sword");
Events.LootMedicine(player);
if (player.HP >= 15)
{
Events.LootHighGradeMedicine(player);
}
else if (player.HP <= 13)
{
Events.GodBlessing(player);
}
else
{
Events.LootMedicine(player);
}
player.Stats();
Console.WriteLine("nPress Enter to continue.");
Console.ReadLine();
Console.Clear();
Story.S_3_Dragons(); // third part of the story
foreach (Mob mob in dragons)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Console.Clear();
Story.S_End(player);
break;
}
Console.ReadLine();
}
}
c#
New contributor
Cavalex is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
Guys I wanted your opinion on the first RPG game I made in C#, what things can I improve on with the code and suggestions about more advanced projects that I should work on in the future.
Events:
static class Events
{
public static void SpawnWeapon(string weapon_name)
{
Console.WriteLine("nAfter that fight you looted a weapon from the enemy party: {0}", weapon_name);
Player.EquipWeapon(weapon_name);
}
public static void LootHerbs(Player player)
{
Console.WriteLine("nYou also managed to loot some herbs that you will use to cure you! You gained 3 HP.");
player.HP += 3;
}
public static void LootSpecialHerbs(Player player)
{
Console.WriteLine("nYou also managed to loot some special herbs that you will use to cure you! You gained 5 HP.");
player.HP += 5;
}
public static void LootMedicine(Player player)
{
Console.WriteLine("nYou looted some medicine that you will use to cure you! You gained 5 HP.");
player.HP += 5;
}
public static void LootHighGradeMedicine(Player player)
{
Console.WriteLine("nAnd you also managed to loot some high grade medicine that you will use to cure you! You gained 7 HP.");
player.HP += 7;
}
public static void GodBlessing(Player player)
{
Console.WriteLine("nAs you were walking towards the dragon nest, you suddenly hear a angelical voice behind youn" +
"and your wounds magically heal. You were blessed by a god to finish your journey! You gained 12 HP.");
player.HP += 12;
}
}
Battle:
static class Battle
{
public static int crit_chance = 16; // 1 in 15 attacks is a crit. ( Yes, 15 ).
public static int attack_timer = 1500;
public static bool isCrit()
{
Random rnd = new Random();
int crit = rnd.Next(1, crit_chance);
if (crit == 1)
{
return true;
}
return false;
}
public static bool isPlayerDead(Player player)
{
if (player.HP <= 0)
{
return true;
}
return false;
}
public static bool isMobDead(Mob mob)
{
if (mob.HP <= 0)
{
return true;
}
return false;
}
public static void PlayerAttack(Player player, Mob mob)
{
bool was_crit = false;
int DamageInflicted()
{
int damage = player.Attack() - mob.Defense();
if (damage <= 1)
{
damage = 1;
}
if (isCrit() == true)
{
was_crit = true;
damage += damage;
}
return damage;
}
mob.HP -= DamageInflicted();
if (was_crit == true)
{
Console.WriteLine("It was a critical hit! You dealt {0} damage.", DamageInflicted());
}
else
{
Console.WriteLine("You dealt {0} damage.", DamageInflicted());
}
Console.WriteLine("The enemy has {0} HP.", mob.HP);
}
public static void EnemyAttack(Player player, Mob mob)
{
bool was_crit = false;
int DamageInflicted()
{
int damage = mob.Attack() - player.Defense();
if (damage <= 0)
{
damage = 0;
}
if (isCrit() == true)
{
was_crit = true;
damage += damage;
}
return damage;
}
player.HP -= DamageInflicted();
if (was_crit == true)
{
Console.WriteLine("It was a critical hit! The enemy dealt {0} damage.", DamageInflicted());
}
else
{
Console.WriteLine("The enemy dealt {0} damage.", DamageInflicted());
}
Console.WriteLine("You have {0} HP.", player.HP);
}
public static void MakeBattle(Player player, Mob mob)
{
Console.WriteLine("You, {0}, will now fight the {1} {2}.", player.name, mob.specie, mob.name);
mob.Stats();
Console.WriteLine("Press Enter to begin the fight.");
Console.ReadLine();
Random rnd = new Random();
int move = rnd.Next(1, 3);
if (move == 1)
{
Console.WriteLine("You begin!");
while (true)
{
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
PlayerAttack(player, mob);
if (isMobDead(mob))
{
Console.WriteLine("You killed the {0}!", mob.specie);
Console.ReadLine();
Console.Clear();
break;
}
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
EnemyAttack(player, mob);
if (isPlayerDead(player))
{
Console.WriteLine("You died! Game Over!");
break;
}
}
}
else
{
Console.WriteLine("The enemy will begin!");
while (true)
{
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
EnemyAttack(player, mob);
if (isPlayerDead(player))
{
Console.WriteLine("You died! Game Over!");
break;
}
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
PlayerAttack(player, mob);
if (isMobDead(mob))
{
Console.WriteLine("You killed the {0}!", mob.specie);
Console.ReadLine();
Console.Clear();
break;
}
}
}
}
}
Mob:
class Mob
{
public string specie;
public string name;
public int base_attack = 2;
public int base_defense = 1;
public int HP;
public int mob_defense;
public int mob_attack;
public Mob(string aSpecie, string aName, int aHP, int aAttack, int aDefense)
{
specie = aSpecie;
name = aName;
HP = aHP;
mob_attack = aAttack;
mob_defense = aDefense;
}
public int Attack()
{
return (base_attack + mob_attack);
}
public int Defense()
{
return (base_defense + mob_defense);
}
public void Stats()
{
Console.WriteLine("The {0} {1}'s Stats:", specie, name);
Console.WriteLine("HP: {0}", HP);
Console.WriteLine("Attack: {0}", Attack());
Console.WriteLine("Defense: {0}", Defense());
}
}
Story:
static class Story
{
public static void S_1_Bandits(Player player)
{
Console.WriteLine("You are " + player.name + ", who's on his way to kill the dragons which are destroying the kingdom.");
Console.WriteLine("As you are on your way to the lairs of the dragons, you run into a couple of bandits.");
Console.WriteLine("And they don't seem so friendly...");
Console.ReadLine();
Console.Clear();
}
public static void S_2_Knights()
{
Console.WriteLine("The bandits weren't much of a match for you. Well Done! You continue on to the dragons lair!");
Console.WriteLine("However, a new movement has risen that wants to protect the dragons of the world.");
Console.WriteLine("Many people have joined this movement, including some knights.");
Console.WriteLine("And uh oh, 3 of them have found out about your quest...");
Console.WriteLine("Maybe they're friendly?");
Console.ReadLine();
Console.WriteLine("Nope.");
Console.ReadLine();
Console.Clear();
}
public static void S_3_Dragons()
{
Console.WriteLine("With the knights defeated you continue on your journey!");
Console.WriteLine("After a while you make it to the lair of dragons...");
Console.WriteLine("It's hot and little smokey in there. You put your sight on the pair of dragons in the center of it.");
Console.WriteLine("The time has come to end the dragons rampage!");
Console.ReadLine();
Console.Clear();
}
public static void S_End(Player player)
{
Console.WriteLine("You killed the dragons and saved the kingdom!");
Console.WriteLine("Your name will be remembered forever and legends will be told about the hero {0} that defeated the evil dragons!", player.name);
Console.WriteLine("Congrats!");
}
}
Weapon:
class Weapon
{
public string name;
public int attack;
public int defense;
public static List<Weapon> weapon_list = new List<Weapon>();
public Weapon(string aName, int aAttack, int aDefense)
{
name = aName;
attack = aAttack;
defense = aDefense;
}
public static void CreateWeapon( string name, int attack, int defense)
{
Weapon weapon = new Weapon(name, attack, defense);
weapon_list.Add(weapon);
}
public static void CheckAllAvailableWeaponsStats()
{
Console.WriteLine("nAll weapons in the game:");
foreach (Weapon weapon in Weapon.weapon_list)
{
Console.Write("Name: {0}nAttack: {1}nDefense: {2}n", weapon.name, weapon.attack, weapon.defense);
Console.WriteLine("---------------------------");
}
}
public static void CheckWeaponStats(Weapon weapon)
{
Console.Write("nName: {0}nAttack: {1}nDefense: {2}n", weapon.name, weapon.attack, weapon.defense);
}
public static void CompareWeaponStats(Weapon other_weapon, Weapon your_weapon)
{
if (your_weapon == Player.equipped_weapon)
{
Console.Write("Name: {0} | Equipped Weapon Name: {1}nAttack: {2} | Equipped Weapon Attack: {3} nDefense: {4} |" +
" Equipped Weapon Defense: {5}n", other_weapon.name, your_weapon.name, other_weapon.attack, your_weapon.attack, other_weapon.defense, your_weapon.defense);
}
else
{
Console.Write("Other Weapon Name: {0} | Your Weapon Name: {1}nOther Weapon Attack: {2} | Your Weapon Attack: {3} nOther Weapon Defense: {4} " +
"| Your Weapon Defense: {5}n", other_weapon.name, your_weapon.name, other_weapon.attack, your_weapon.attack, other_weapon.defense, your_weapon.defense);
}
}
}
Player:
class Player
{
public static Weapon initial_sword = new Weapon("Initial Sword", 8, 4);
public string name;
public int HP;
public int level = 0;
public static Weapon equipped_weapon = initial_sword;
public int base_attack = 4;
public int base_defense = 2;
public Player(string aName, int aHP)
{
name = aName;
HP = aHP;
}
public int Attack()
{
return (base_attack + equipped_weapon.attack);
}
public int Defense()
{
return (base_defense + equipped_weapon.defense);
}
public void Stats()
{
Console.WriteLine("n{0}'s Stats:", name);
Console.WriteLine("HP: {0}", HP);
Console.WriteLine("Attack: {0} ({1})", Attack(), base_attack);
Console.WriteLine("Defense: {0} ({1})", Defense(), base_defense);
Console.WriteLine("Equipped Weapon: {0}; AT: {1}; DF: {2}", equipped_weapon.name, equipped_weapon.attack, equipped_weapon.defense);
}
public static bool QuestionPrompt()
{
string yes_list = { "yes", "", "sure", "y", "yeah", "why not", "yes.", "y." };
Console.Write("-->");
string input = Console.ReadLine();
string iinput = input.ToLower();
foreach (string value in yes_list)
{
if (value.Equals(iinput))
{
return true;
}
else
{
continue;
}
}
return false;
}
public static void ChangeWeaponByName(string new_weapon_name)
{
Weapon weapon_to_change = new Weapon("Test Weapon", 0, 0);
bool WeaponExists()
{
foreach (Weapon weapon in Weapon.weapon_list)
{
if (weapon.name.ToLower() == new_weapon_name.ToLower())
{
weapon_to_change = weapon;
return true;
}
else
{
continue;
}
}
return false;
}
if (WeaponExists() == true)
{
equipped_weapon = weapon_to_change;
}
}
public static void ChangeWeapon(Weapon new_weapon)
{
equipped_weapon = new_weapon;
}
public static void EquipWeapon(string weapon_name)
{
Weapon weapon_to_equip = new Weapon("Test Weapon", 0, 0);
bool WeaponExists()
{
foreach (Weapon weapon in Weapon.weapon_list)
{
if (weapon.name.ToLower() == weapon_name.ToLower())
{
weapon_to_equip = weapon;
return true;
}
else
{
continue;
}
}
return false;
}
if (WeaponExists())
{
Console.WriteLine("nComparison of both weapons stats:");
Weapon.CompareWeaponStats(weapon_to_equip, Player.equipped_weapon);
Console.WriteLine("Are you sure you want to equip this weapon?");
if (QuestionPrompt() == true)
{
Console.WriteLine("You equipped the weapon!");
ChangeWeapon(weapon_to_equip);
}
else
{
Console.WriteLine("You will continue with the same weapon, the new one was discarded.");
}
}
else
{
Console.WriteLine("The weapon you want to equip doesn't exist!");
}
}
public static void CheckEquippedWeapon()
{
Console.WriteLine("Equipped Weapon:");
Weapon.CheckWeaponStats(equipped_weapon);
}
}
Main:
static void Main()
{
Player player = new Player("", 30);
bool PlayerDied()
{
if (Battle.isPlayerDead(player) == true)
{
return true;
}
return false;
}
Weapon.CreateWeapon("Rusty Old Sword", 7, 3); // initial weapon
Weapon.CreateWeapon("Iron Sword", 10, 5); // to give the player after he fights the bandits
Weapon.CreateWeapon("Enchanted Silver Sword", 15, 7); // to give the player after he fights the knights
Player.ChangeWeaponByName("rusty old sword");
// the enemies
Mob bandits =
{
new Mob("Bandit", "Rob", 10, 6, 2),
new Mob("Bandit Leader", "Joe", 10, 7, 2)
};
Mob knights =
{
new Mob("Knight", "Rob", 12, 8, 4),
new Mob("Knight", "John", 12, 9, 3),
new Mob("Knight Captain", "Aaron", 14, 10, 4),
};
Mob dragons =
{
new Mob("Blue Dragon", "Jormungandr", 16, 10, 6),
new Mob("Dragon Leader", "Helios", 18, 11, 6),
};
Console.WriteLine("What's your name, adventurer?");
string response = Console.ReadLine();
player.name = response;
player.Stats();
Console.WriteLine("nPress anything to begin the game.");
Console.ReadLine();
Console.Clear();
while (true)
{
Story.S_1_Bandits(player); // first part of the story
foreach (Mob mob in bandits)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Events.SpawnWeapon("Iron Sword");
if (player.HP <= 16)
{
Events.LootSpecialHerbs(player);
}
else
{
Events.LootHerbs(player);
}
player.Stats();
Console.WriteLine("nPress Enter to continue.");
Console.ReadLine();
Console.Clear();
Story.S_2_Knights(); // second part of the story
foreach (Mob mob in knights)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Events.SpawnWeapon("Enchanted Silver Sword");
Events.LootMedicine(player);
if (player.HP >= 15)
{
Events.LootHighGradeMedicine(player);
}
else if (player.HP <= 13)
{
Events.GodBlessing(player);
}
else
{
Events.LootMedicine(player);
}
player.Stats();
Console.WriteLine("nPress Enter to continue.");
Console.ReadLine();
Console.Clear();
Story.S_3_Dragons(); // third part of the story
foreach (Mob mob in dragons)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Console.Clear();
Story.S_End(player);
break;
}
Console.ReadLine();
}
}
c#
New contributor
Cavalex is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Guys I wanted your opinion on the first RPG game I made in C#, what things can I improve on with the code and suggestions about more advanced projects that I should work on in the future.
Events:
static class Events
{
public static void SpawnWeapon(string weapon_name)
{
Console.WriteLine("nAfter that fight you looted a weapon from the enemy party: {0}", weapon_name);
Player.EquipWeapon(weapon_name);
}
public static void LootHerbs(Player player)
{
Console.WriteLine("nYou also managed to loot some herbs that you will use to cure you! You gained 3 HP.");
player.HP += 3;
}
public static void LootSpecialHerbs(Player player)
{
Console.WriteLine("nYou also managed to loot some special herbs that you will use to cure you! You gained 5 HP.");
player.HP += 5;
}
public static void LootMedicine(Player player)
{
Console.WriteLine("nYou looted some medicine that you will use to cure you! You gained 5 HP.");
player.HP += 5;
}
public static void LootHighGradeMedicine(Player player)
{
Console.WriteLine("nAnd you also managed to loot some high grade medicine that you will use to cure you! You gained 7 HP.");
player.HP += 7;
}
public static void GodBlessing(Player player)
{
Console.WriteLine("nAs you were walking towards the dragon nest, you suddenly hear a angelical voice behind youn" +
"and your wounds magically heal. You were blessed by a god to finish your journey! You gained 12 HP.");
player.HP += 12;
}
}
Battle:
static class Battle
{
public static int crit_chance = 16; // 1 in 15 attacks is a crit. ( Yes, 15 ).
public static int attack_timer = 1500;
public static bool isCrit()
{
Random rnd = new Random();
int crit = rnd.Next(1, crit_chance);
if (crit == 1)
{
return true;
}
return false;
}
public static bool isPlayerDead(Player player)
{
if (player.HP <= 0)
{
return true;
}
return false;
}
public static bool isMobDead(Mob mob)
{
if (mob.HP <= 0)
{
return true;
}
return false;
}
public static void PlayerAttack(Player player, Mob mob)
{
bool was_crit = false;
int DamageInflicted()
{
int damage = player.Attack() - mob.Defense();
if (damage <= 1)
{
damage = 1;
}
if (isCrit() == true)
{
was_crit = true;
damage += damage;
}
return damage;
}
mob.HP -= DamageInflicted();
if (was_crit == true)
{
Console.WriteLine("It was a critical hit! You dealt {0} damage.", DamageInflicted());
}
else
{
Console.WriteLine("You dealt {0} damage.", DamageInflicted());
}
Console.WriteLine("The enemy has {0} HP.", mob.HP);
}
public static void EnemyAttack(Player player, Mob mob)
{
bool was_crit = false;
int DamageInflicted()
{
int damage = mob.Attack() - player.Defense();
if (damage <= 0)
{
damage = 0;
}
if (isCrit() == true)
{
was_crit = true;
damage += damage;
}
return damage;
}
player.HP -= DamageInflicted();
if (was_crit == true)
{
Console.WriteLine("It was a critical hit! The enemy dealt {0} damage.", DamageInflicted());
}
else
{
Console.WriteLine("The enemy dealt {0} damage.", DamageInflicted());
}
Console.WriteLine("You have {0} HP.", player.HP);
}
public static void MakeBattle(Player player, Mob mob)
{
Console.WriteLine("You, {0}, will now fight the {1} {2}.", player.name, mob.specie, mob.name);
mob.Stats();
Console.WriteLine("Press Enter to begin the fight.");
Console.ReadLine();
Random rnd = new Random();
int move = rnd.Next(1, 3);
if (move == 1)
{
Console.WriteLine("You begin!");
while (true)
{
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
PlayerAttack(player, mob);
if (isMobDead(mob))
{
Console.WriteLine("You killed the {0}!", mob.specie);
Console.ReadLine();
Console.Clear();
break;
}
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
EnemyAttack(player, mob);
if (isPlayerDead(player))
{
Console.WriteLine("You died! Game Over!");
break;
}
}
}
else
{
Console.WriteLine("The enemy will begin!");
while (true)
{
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
EnemyAttack(player, mob);
if (isPlayerDead(player))
{
Console.WriteLine("You died! Game Over!");
break;
}
Console.WriteLine();
System.Threading.Thread.Sleep(attack_timer);
PlayerAttack(player, mob);
if (isMobDead(mob))
{
Console.WriteLine("You killed the {0}!", mob.specie);
Console.ReadLine();
Console.Clear();
break;
}
}
}
}
}
Mob:
class Mob
{
public string specie;
public string name;
public int base_attack = 2;
public int base_defense = 1;
public int HP;
public int mob_defense;
public int mob_attack;
public Mob(string aSpecie, string aName, int aHP, int aAttack, int aDefense)
{
specie = aSpecie;
name = aName;
HP = aHP;
mob_attack = aAttack;
mob_defense = aDefense;
}
public int Attack()
{
return (base_attack + mob_attack);
}
public int Defense()
{
return (base_defense + mob_defense);
}
public void Stats()
{
Console.WriteLine("The {0} {1}'s Stats:", specie, name);
Console.WriteLine("HP: {0}", HP);
Console.WriteLine("Attack: {0}", Attack());
Console.WriteLine("Defense: {0}", Defense());
}
}
Story:
static class Story
{
public static void S_1_Bandits(Player player)
{
Console.WriteLine("You are " + player.name + ", who's on his way to kill the dragons which are destroying the kingdom.");
Console.WriteLine("As you are on your way to the lairs of the dragons, you run into a couple of bandits.");
Console.WriteLine("And they don't seem so friendly...");
Console.ReadLine();
Console.Clear();
}
public static void S_2_Knights()
{
Console.WriteLine("The bandits weren't much of a match for you. Well Done! You continue on to the dragons lair!");
Console.WriteLine("However, a new movement has risen that wants to protect the dragons of the world.");
Console.WriteLine("Many people have joined this movement, including some knights.");
Console.WriteLine("And uh oh, 3 of them have found out about your quest...");
Console.WriteLine("Maybe they're friendly?");
Console.ReadLine();
Console.WriteLine("Nope.");
Console.ReadLine();
Console.Clear();
}
public static void S_3_Dragons()
{
Console.WriteLine("With the knights defeated you continue on your journey!");
Console.WriteLine("After a while you make it to the lair of dragons...");
Console.WriteLine("It's hot and little smokey in there. You put your sight on the pair of dragons in the center of it.");
Console.WriteLine("The time has come to end the dragons rampage!");
Console.ReadLine();
Console.Clear();
}
public static void S_End(Player player)
{
Console.WriteLine("You killed the dragons and saved the kingdom!");
Console.WriteLine("Your name will be remembered forever and legends will be told about the hero {0} that defeated the evil dragons!", player.name);
Console.WriteLine("Congrats!");
}
}
Weapon:
class Weapon
{
public string name;
public int attack;
public int defense;
public static List<Weapon> weapon_list = new List<Weapon>();
public Weapon(string aName, int aAttack, int aDefense)
{
name = aName;
attack = aAttack;
defense = aDefense;
}
public static void CreateWeapon( string name, int attack, int defense)
{
Weapon weapon = new Weapon(name, attack, defense);
weapon_list.Add(weapon);
}
public static void CheckAllAvailableWeaponsStats()
{
Console.WriteLine("nAll weapons in the game:");
foreach (Weapon weapon in Weapon.weapon_list)
{
Console.Write("Name: {0}nAttack: {1}nDefense: {2}n", weapon.name, weapon.attack, weapon.defense);
Console.WriteLine("---------------------------");
}
}
public static void CheckWeaponStats(Weapon weapon)
{
Console.Write("nName: {0}nAttack: {1}nDefense: {2}n", weapon.name, weapon.attack, weapon.defense);
}
public static void CompareWeaponStats(Weapon other_weapon, Weapon your_weapon)
{
if (your_weapon == Player.equipped_weapon)
{
Console.Write("Name: {0} | Equipped Weapon Name: {1}nAttack: {2} | Equipped Weapon Attack: {3} nDefense: {4} |" +
" Equipped Weapon Defense: {5}n", other_weapon.name, your_weapon.name, other_weapon.attack, your_weapon.attack, other_weapon.defense, your_weapon.defense);
}
else
{
Console.Write("Other Weapon Name: {0} | Your Weapon Name: {1}nOther Weapon Attack: {2} | Your Weapon Attack: {3} nOther Weapon Defense: {4} " +
"| Your Weapon Defense: {5}n", other_weapon.name, your_weapon.name, other_weapon.attack, your_weapon.attack, other_weapon.defense, your_weapon.defense);
}
}
}
Player:
class Player
{
public static Weapon initial_sword = new Weapon("Initial Sword", 8, 4);
public string name;
public int HP;
public int level = 0;
public static Weapon equipped_weapon = initial_sword;
public int base_attack = 4;
public int base_defense = 2;
public Player(string aName, int aHP)
{
name = aName;
HP = aHP;
}
public int Attack()
{
return (base_attack + equipped_weapon.attack);
}
public int Defense()
{
return (base_defense + equipped_weapon.defense);
}
public void Stats()
{
Console.WriteLine("n{0}'s Stats:", name);
Console.WriteLine("HP: {0}", HP);
Console.WriteLine("Attack: {0} ({1})", Attack(), base_attack);
Console.WriteLine("Defense: {0} ({1})", Defense(), base_defense);
Console.WriteLine("Equipped Weapon: {0}; AT: {1}; DF: {2}", equipped_weapon.name, equipped_weapon.attack, equipped_weapon.defense);
}
public static bool QuestionPrompt()
{
string yes_list = { "yes", "", "sure", "y", "yeah", "why not", "yes.", "y." };
Console.Write("-->");
string input = Console.ReadLine();
string iinput = input.ToLower();
foreach (string value in yes_list)
{
if (value.Equals(iinput))
{
return true;
}
else
{
continue;
}
}
return false;
}
public static void ChangeWeaponByName(string new_weapon_name)
{
Weapon weapon_to_change = new Weapon("Test Weapon", 0, 0);
bool WeaponExists()
{
foreach (Weapon weapon in Weapon.weapon_list)
{
if (weapon.name.ToLower() == new_weapon_name.ToLower())
{
weapon_to_change = weapon;
return true;
}
else
{
continue;
}
}
return false;
}
if (WeaponExists() == true)
{
equipped_weapon = weapon_to_change;
}
}
public static void ChangeWeapon(Weapon new_weapon)
{
equipped_weapon = new_weapon;
}
public static void EquipWeapon(string weapon_name)
{
Weapon weapon_to_equip = new Weapon("Test Weapon", 0, 0);
bool WeaponExists()
{
foreach (Weapon weapon in Weapon.weapon_list)
{
if (weapon.name.ToLower() == weapon_name.ToLower())
{
weapon_to_equip = weapon;
return true;
}
else
{
continue;
}
}
return false;
}
if (WeaponExists())
{
Console.WriteLine("nComparison of both weapons stats:");
Weapon.CompareWeaponStats(weapon_to_equip, Player.equipped_weapon);
Console.WriteLine("Are you sure you want to equip this weapon?");
if (QuestionPrompt() == true)
{
Console.WriteLine("You equipped the weapon!");
ChangeWeapon(weapon_to_equip);
}
else
{
Console.WriteLine("You will continue with the same weapon, the new one was discarded.");
}
}
else
{
Console.WriteLine("The weapon you want to equip doesn't exist!");
}
}
public static void CheckEquippedWeapon()
{
Console.WriteLine("Equipped Weapon:");
Weapon.CheckWeaponStats(equipped_weapon);
}
}
Main:
static void Main()
{
Player player = new Player("", 30);
bool PlayerDied()
{
if (Battle.isPlayerDead(player) == true)
{
return true;
}
return false;
}
Weapon.CreateWeapon("Rusty Old Sword", 7, 3); // initial weapon
Weapon.CreateWeapon("Iron Sword", 10, 5); // to give the player after he fights the bandits
Weapon.CreateWeapon("Enchanted Silver Sword", 15, 7); // to give the player after he fights the knights
Player.ChangeWeaponByName("rusty old sword");
// the enemies
Mob bandits =
{
new Mob("Bandit", "Rob", 10, 6, 2),
new Mob("Bandit Leader", "Joe", 10, 7, 2)
};
Mob knights =
{
new Mob("Knight", "Rob", 12, 8, 4),
new Mob("Knight", "John", 12, 9, 3),
new Mob("Knight Captain", "Aaron", 14, 10, 4),
};
Mob dragons =
{
new Mob("Blue Dragon", "Jormungandr", 16, 10, 6),
new Mob("Dragon Leader", "Helios", 18, 11, 6),
};
Console.WriteLine("What's your name, adventurer?");
string response = Console.ReadLine();
player.name = response;
player.Stats();
Console.WriteLine("nPress anything to begin the game.");
Console.ReadLine();
Console.Clear();
while (true)
{
Story.S_1_Bandits(player); // first part of the story
foreach (Mob mob in bandits)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Events.SpawnWeapon("Iron Sword");
if (player.HP <= 16)
{
Events.LootSpecialHerbs(player);
}
else
{
Events.LootHerbs(player);
}
player.Stats();
Console.WriteLine("nPress Enter to continue.");
Console.ReadLine();
Console.Clear();
Story.S_2_Knights(); // second part of the story
foreach (Mob mob in knights)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Events.SpawnWeapon("Enchanted Silver Sword");
Events.LootMedicine(player);
if (player.HP >= 15)
{
Events.LootHighGradeMedicine(player);
}
else if (player.HP <= 13)
{
Events.GodBlessing(player);
}
else
{
Events.LootMedicine(player);
}
player.Stats();
Console.WriteLine("nPress Enter to continue.");
Console.ReadLine();
Console.Clear();
Story.S_3_Dragons(); // third part of the story
foreach (Mob mob in dragons)
{
Battle.MakeBattle(player, mob);
if (Battle.isPlayerDead(player) == true)
{
break;
}
}
if (PlayerDied() == true)
{
break;
}
Console.Clear();
Story.S_End(player);
break;
}
Console.ReadLine();
}
}
c#
c#
New contributor
Cavalex is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Cavalex is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Cavalex is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 5 mins ago


Cavalex
11
11
New contributor
Cavalex is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Cavalex is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Cavalex is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Cavalex is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f210153%2ffirst-c-rpg-game-and-project-as-a-beginner%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Cavalex is a new contributor. Be nice, and check out our Code of Conduct.
Cavalex is a new contributor. Be nice, and check out our Code of Conduct.
Cavalex is a new contributor. Be nice, and check out our Code of Conduct.
Cavalex is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f210153%2ffirst-c-rpg-game-and-project-as-a-beginner%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hqAVF,7F9DKki