Using try, catch and finally in test class (dreamhouseapp)
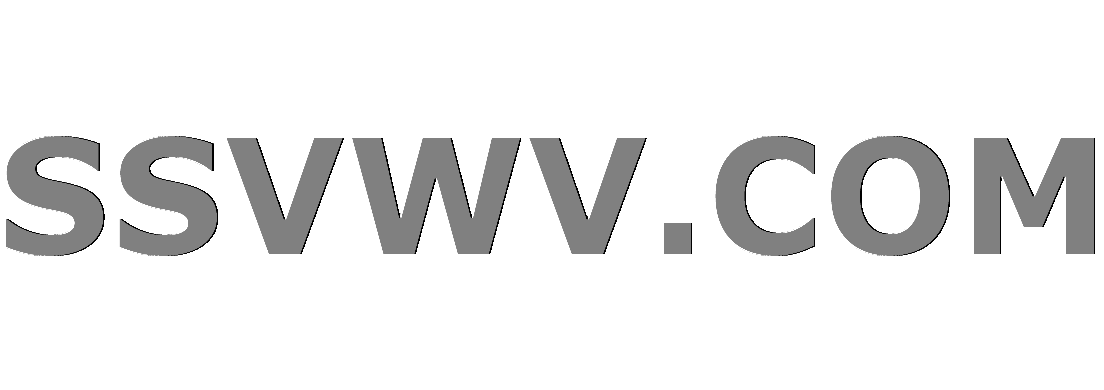
Multi tool use
up vote
5
down vote
favorite
In the test classes of the DreamHouse Sample app all the functions have the following structure:
static testMethod void testSomething() {
Boolean success = true;
try {
...
} catch (Exception e) {
success = false;
} finally {
System.assert(success);
}
}
Source: https://github.com/dreamhouseapp/dreamhouse-sfdx/blob/master/force-app/main/default/classes/PropertyControllerTest.cls
What is the reason for or advantage of this structure?
I am asking, since this app is kind of Salesforce-official (if I got that right) and it says "Get inspired and learn best practices.", so I thought there must be a reason...
Thank you!
unit-test try-catch
add a comment |
up vote
5
down vote
favorite
In the test classes of the DreamHouse Sample app all the functions have the following structure:
static testMethod void testSomething() {
Boolean success = true;
try {
...
} catch (Exception e) {
success = false;
} finally {
System.assert(success);
}
}
Source: https://github.com/dreamhouseapp/dreamhouse-sfdx/blob/master/force-app/main/default/classes/PropertyControllerTest.cls
What is the reason for or advantage of this structure?
I am asking, since this app is kind of Salesforce-official (if I got that right) and it says "Get inspired and learn best practices.", so I thought there must be a reason...
Thank you!
unit-test try-catch
2
I'm curious to see if there's an answer to the contrary, but I don't see the advantage to that structure or think it's best practice. I'd call those smoke tests myself; the assertion proves nothing and actually hides an exception that might have included valuable information.
– David Reed
9 hours ago
add a comment |
up vote
5
down vote
favorite
up vote
5
down vote
favorite
In the test classes of the DreamHouse Sample app all the functions have the following structure:
static testMethod void testSomething() {
Boolean success = true;
try {
...
} catch (Exception e) {
success = false;
} finally {
System.assert(success);
}
}
Source: https://github.com/dreamhouseapp/dreamhouse-sfdx/blob/master/force-app/main/default/classes/PropertyControllerTest.cls
What is the reason for or advantage of this structure?
I am asking, since this app is kind of Salesforce-official (if I got that right) and it says "Get inspired and learn best practices.", so I thought there must be a reason...
Thank you!
unit-test try-catch
In the test classes of the DreamHouse Sample app all the functions have the following structure:
static testMethod void testSomething() {
Boolean success = true;
try {
...
} catch (Exception e) {
success = false;
} finally {
System.assert(success);
}
}
Source: https://github.com/dreamhouseapp/dreamhouse-sfdx/blob/master/force-app/main/default/classes/PropertyControllerTest.cls
What is the reason for or advantage of this structure?
I am asking, since this app is kind of Salesforce-official (if I got that right) and it says "Get inspired and learn best practices.", so I thought there must be a reason...
Thank you!
unit-test try-catch
unit-test try-catch
asked 9 hours ago
martes
304
304
2
I'm curious to see if there's an answer to the contrary, but I don't see the advantage to that structure or think it's best practice. I'd call those smoke tests myself; the assertion proves nothing and actually hides an exception that might have included valuable information.
– David Reed
9 hours ago
add a comment |
2
I'm curious to see if there's an answer to the contrary, but I don't see the advantage to that structure or think it's best practice. I'd call those smoke tests myself; the assertion proves nothing and actually hides an exception that might have included valuable information.
– David Reed
9 hours ago
2
2
I'm curious to see if there's an answer to the contrary, but I don't see the advantage to that structure or think it's best practice. I'd call those smoke tests myself; the assertion proves nothing and actually hides an exception that might have included valuable information.
– David Reed
9 hours ago
I'm curious to see if there's an answer to the contrary, but I don't see the advantage to that structure or think it's best practice. I'd call those smoke tests myself; the assertion proves nothing and actually hides an exception that might have included valuable information.
– David Reed
9 hours ago
add a comment |
3 Answers
3
active
oldest
votes
up vote
3
down vote
finally
-assert
is not your friend. Do not ever do this. Finally executes even if an exception is thrown, so the test will fail, but for the wrong reason. In any situation where it'd make sense to use finally, you can do it shorter without finally.
In fact, you should only use try-catch if you expect a specific exception:
try {
doSomething();
System.assert(false, 'Expected to get an exception');
} catch(SpecificException e) {
// Good, but maybe System.assert for a specific message, etc
}
If no exception is thrown, you get an assertion failure, if you get the wrong exception, you get a failed test from the thrown exception, otherwise the test passes. finally
might look pretty, but it's really just adding more code for no real reason. finally
is useful in some situations, but not in unit tests.
add a comment |
up vote
0
down vote
The test framework reports exceptions as failures so let it do the work for you. Your question example then simplifies to:
@IsTest
static void something() {
...
}
This Simplicity in Software Design: KISS, YAGNI and Occam’s Razor blog post is relevant here and contains this lovely quote:
Perfection is achieved, not when there is nothing more to add, but
when there is nothing left to take away.
I see that not all the DreamHouse tests use that pattern - e.g. BotTest does not. Perhaps the try/catch/finally code was trying to express the idea that the test was there to check for exceptions. A comment would be a good way to communicate that.
add a comment |
up vote
-1
down vote
Personally, I find the below structure clearer and it is the best practice we advise where I work.
Our Best Practice
SpecificException unexpectedException;
Test.startTest();
try
{
// logic here
}
catch (SpecificException e)
{
unexpectedException = e;
}
Test.stopTest();
system.assertEquals(null, unexpectedException, 'Informative message');
The reason we advise this pattern is that you should never put your test assertions within a conditional block, which executes only some of the time. Always make every assertion you intend. Note that if there is an exception, the assertion will show you a good level of detail.
There are variations of anti-pattern which violate this axiom but one typical example is below.
Anti-Pattern
Test.startTest();
try
{
// logic here
}
catch (SpecificException e)
{
system.assert(false, 'Informative message');
}
Test.stopTest();
This anti-pattern violates our axiom that each assertion should be unconditional.
The App
The pattern demonstrated in the app does avoid any violation of the axiom, so the pattern itself would probably pass our code review. However, it would be a marked improvement to cache the specific Exception
instance rather than a Boolean
flag, and in my opinion it is less clear to nest your blocks in this way.
One final note, we consider it firmly not best practice to catch generic Exception
also colloquially called a "pokemon catch"). You should know what type of exception you expect, and handle just that. So while the try
/catch
/finally
pattern would make it through code review (with comment), that aspect of the code would not.
Interesting theory, but why do you advise this over just not using try-catch at all and just let the exception fail the test directly? This gives you an immediate error and stacktrace without the extra assertions, etc. I'd rather focus on writing assertions that prove everything worked successfully rather than checking for specific exceptions that we might get.
– sfdcfox
8 hours ago
Also, "This anti-pattern violates our axiom that each assertion should be unconditional." Don't you mean "conditional"?
– sfdcfox
8 hours ago
We've been over this already in comments on other threads. We contend that tests should only fail via assertion. I know you disagree.
– Adrian Larson♦
7 hours ago
Sorry, I must have forgot. I've had a lot on my mind recently.
– sfdcfox
7 hours ago
1
Haha no offense meant. It's just a bit of a standing dispute between us (though I think we agree to disagree).
– Adrian Larson♦
7 hours ago
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "459"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsalesforce.stackexchange.com%2fquestions%2f243730%2fusing-try-catch-and-finally-in-test-class-dreamhouseapp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
finally
-assert
is not your friend. Do not ever do this. Finally executes even if an exception is thrown, so the test will fail, but for the wrong reason. In any situation where it'd make sense to use finally, you can do it shorter without finally.
In fact, you should only use try-catch if you expect a specific exception:
try {
doSomething();
System.assert(false, 'Expected to get an exception');
} catch(SpecificException e) {
// Good, but maybe System.assert for a specific message, etc
}
If no exception is thrown, you get an assertion failure, if you get the wrong exception, you get a failed test from the thrown exception, otherwise the test passes. finally
might look pretty, but it's really just adding more code for no real reason. finally
is useful in some situations, but not in unit tests.
add a comment |
up vote
3
down vote
finally
-assert
is not your friend. Do not ever do this. Finally executes even if an exception is thrown, so the test will fail, but for the wrong reason. In any situation where it'd make sense to use finally, you can do it shorter without finally.
In fact, you should only use try-catch if you expect a specific exception:
try {
doSomething();
System.assert(false, 'Expected to get an exception');
} catch(SpecificException e) {
// Good, but maybe System.assert for a specific message, etc
}
If no exception is thrown, you get an assertion failure, if you get the wrong exception, you get a failed test from the thrown exception, otherwise the test passes. finally
might look pretty, but it's really just adding more code for no real reason. finally
is useful in some situations, but not in unit tests.
add a comment |
up vote
3
down vote
up vote
3
down vote
finally
-assert
is not your friend. Do not ever do this. Finally executes even if an exception is thrown, so the test will fail, but for the wrong reason. In any situation where it'd make sense to use finally, you can do it shorter without finally.
In fact, you should only use try-catch if you expect a specific exception:
try {
doSomething();
System.assert(false, 'Expected to get an exception');
} catch(SpecificException e) {
// Good, but maybe System.assert for a specific message, etc
}
If no exception is thrown, you get an assertion failure, if you get the wrong exception, you get a failed test from the thrown exception, otherwise the test passes. finally
might look pretty, but it's really just adding more code for no real reason. finally
is useful in some situations, but not in unit tests.
finally
-assert
is not your friend. Do not ever do this. Finally executes even if an exception is thrown, so the test will fail, but for the wrong reason. In any situation where it'd make sense to use finally, you can do it shorter without finally.
In fact, you should only use try-catch if you expect a specific exception:
try {
doSomething();
System.assert(false, 'Expected to get an exception');
} catch(SpecificException e) {
// Good, but maybe System.assert for a specific message, etc
}
If no exception is thrown, you get an assertion failure, if you get the wrong exception, you get a failed test from the thrown exception, otherwise the test passes. finally
might look pretty, but it's really just adding more code for no real reason. finally
is useful in some situations, but not in unit tests.
answered 8 hours ago


sfdcfox
244k10185418
244k10185418
add a comment |
add a comment |
up vote
0
down vote
The test framework reports exceptions as failures so let it do the work for you. Your question example then simplifies to:
@IsTest
static void something() {
...
}
This Simplicity in Software Design: KISS, YAGNI and Occam’s Razor blog post is relevant here and contains this lovely quote:
Perfection is achieved, not when there is nothing more to add, but
when there is nothing left to take away.
I see that not all the DreamHouse tests use that pattern - e.g. BotTest does not. Perhaps the try/catch/finally code was trying to express the idea that the test was there to check for exceptions. A comment would be a good way to communicate that.
add a comment |
up vote
0
down vote
The test framework reports exceptions as failures so let it do the work for you. Your question example then simplifies to:
@IsTest
static void something() {
...
}
This Simplicity in Software Design: KISS, YAGNI and Occam’s Razor blog post is relevant here and contains this lovely quote:
Perfection is achieved, not when there is nothing more to add, but
when there is nothing left to take away.
I see that not all the DreamHouse tests use that pattern - e.g. BotTest does not. Perhaps the try/catch/finally code was trying to express the idea that the test was there to check for exceptions. A comment would be a good way to communicate that.
add a comment |
up vote
0
down vote
up vote
0
down vote
The test framework reports exceptions as failures so let it do the work for you. Your question example then simplifies to:
@IsTest
static void something() {
...
}
This Simplicity in Software Design: KISS, YAGNI and Occam’s Razor blog post is relevant here and contains this lovely quote:
Perfection is achieved, not when there is nothing more to add, but
when there is nothing left to take away.
I see that not all the DreamHouse tests use that pattern - e.g. BotTest does not. Perhaps the try/catch/finally code was trying to express the idea that the test was there to check for exceptions. A comment would be a good way to communicate that.
The test framework reports exceptions as failures so let it do the work for you. Your question example then simplifies to:
@IsTest
static void something() {
...
}
This Simplicity in Software Design: KISS, YAGNI and Occam’s Razor blog post is relevant here and contains this lovely quote:
Perfection is achieved, not when there is nothing more to add, but
when there is nothing left to take away.
I see that not all the DreamHouse tests use that pattern - e.g. BotTest does not. Perhaps the try/catch/finally code was trying to express the idea that the test was there to check for exceptions. A comment would be a good way to communicate that.
edited 35 mins ago
answered 41 mins ago
Keith C
93.9k1088199
93.9k1088199
add a comment |
add a comment |
up vote
-1
down vote
Personally, I find the below structure clearer and it is the best practice we advise where I work.
Our Best Practice
SpecificException unexpectedException;
Test.startTest();
try
{
// logic here
}
catch (SpecificException e)
{
unexpectedException = e;
}
Test.stopTest();
system.assertEquals(null, unexpectedException, 'Informative message');
The reason we advise this pattern is that you should never put your test assertions within a conditional block, which executes only some of the time. Always make every assertion you intend. Note that if there is an exception, the assertion will show you a good level of detail.
There are variations of anti-pattern which violate this axiom but one typical example is below.
Anti-Pattern
Test.startTest();
try
{
// logic here
}
catch (SpecificException e)
{
system.assert(false, 'Informative message');
}
Test.stopTest();
This anti-pattern violates our axiom that each assertion should be unconditional.
The App
The pattern demonstrated in the app does avoid any violation of the axiom, so the pattern itself would probably pass our code review. However, it would be a marked improvement to cache the specific Exception
instance rather than a Boolean
flag, and in my opinion it is less clear to nest your blocks in this way.
One final note, we consider it firmly not best practice to catch generic Exception
also colloquially called a "pokemon catch"). You should know what type of exception you expect, and handle just that. So while the try
/catch
/finally
pattern would make it through code review (with comment), that aspect of the code would not.
Interesting theory, but why do you advise this over just not using try-catch at all and just let the exception fail the test directly? This gives you an immediate error and stacktrace without the extra assertions, etc. I'd rather focus on writing assertions that prove everything worked successfully rather than checking for specific exceptions that we might get.
– sfdcfox
8 hours ago
Also, "This anti-pattern violates our axiom that each assertion should be unconditional." Don't you mean "conditional"?
– sfdcfox
8 hours ago
We've been over this already in comments on other threads. We contend that tests should only fail via assertion. I know you disagree.
– Adrian Larson♦
7 hours ago
Sorry, I must have forgot. I've had a lot on my mind recently.
– sfdcfox
7 hours ago
1
Haha no offense meant. It's just a bit of a standing dispute between us (though I think we agree to disagree).
– Adrian Larson♦
7 hours ago
add a comment |
up vote
-1
down vote
Personally, I find the below structure clearer and it is the best practice we advise where I work.
Our Best Practice
SpecificException unexpectedException;
Test.startTest();
try
{
// logic here
}
catch (SpecificException e)
{
unexpectedException = e;
}
Test.stopTest();
system.assertEquals(null, unexpectedException, 'Informative message');
The reason we advise this pattern is that you should never put your test assertions within a conditional block, which executes only some of the time. Always make every assertion you intend. Note that if there is an exception, the assertion will show you a good level of detail.
There are variations of anti-pattern which violate this axiom but one typical example is below.
Anti-Pattern
Test.startTest();
try
{
// logic here
}
catch (SpecificException e)
{
system.assert(false, 'Informative message');
}
Test.stopTest();
This anti-pattern violates our axiom that each assertion should be unconditional.
The App
The pattern demonstrated in the app does avoid any violation of the axiom, so the pattern itself would probably pass our code review. However, it would be a marked improvement to cache the specific Exception
instance rather than a Boolean
flag, and in my opinion it is less clear to nest your blocks in this way.
One final note, we consider it firmly not best practice to catch generic Exception
also colloquially called a "pokemon catch"). You should know what type of exception you expect, and handle just that. So while the try
/catch
/finally
pattern would make it through code review (with comment), that aspect of the code would not.
Interesting theory, but why do you advise this over just not using try-catch at all and just let the exception fail the test directly? This gives you an immediate error and stacktrace without the extra assertions, etc. I'd rather focus on writing assertions that prove everything worked successfully rather than checking for specific exceptions that we might get.
– sfdcfox
8 hours ago
Also, "This anti-pattern violates our axiom that each assertion should be unconditional." Don't you mean "conditional"?
– sfdcfox
8 hours ago
We've been over this already in comments on other threads. We contend that tests should only fail via assertion. I know you disagree.
– Adrian Larson♦
7 hours ago
Sorry, I must have forgot. I've had a lot on my mind recently.
– sfdcfox
7 hours ago
1
Haha no offense meant. It's just a bit of a standing dispute between us (though I think we agree to disagree).
– Adrian Larson♦
7 hours ago
add a comment |
up vote
-1
down vote
up vote
-1
down vote
Personally, I find the below structure clearer and it is the best practice we advise where I work.
Our Best Practice
SpecificException unexpectedException;
Test.startTest();
try
{
// logic here
}
catch (SpecificException e)
{
unexpectedException = e;
}
Test.stopTest();
system.assertEquals(null, unexpectedException, 'Informative message');
The reason we advise this pattern is that you should never put your test assertions within a conditional block, which executes only some of the time. Always make every assertion you intend. Note that if there is an exception, the assertion will show you a good level of detail.
There are variations of anti-pattern which violate this axiom but one typical example is below.
Anti-Pattern
Test.startTest();
try
{
// logic here
}
catch (SpecificException e)
{
system.assert(false, 'Informative message');
}
Test.stopTest();
This anti-pattern violates our axiom that each assertion should be unconditional.
The App
The pattern demonstrated in the app does avoid any violation of the axiom, so the pattern itself would probably pass our code review. However, it would be a marked improvement to cache the specific Exception
instance rather than a Boolean
flag, and in my opinion it is less clear to nest your blocks in this way.
One final note, we consider it firmly not best practice to catch generic Exception
also colloquially called a "pokemon catch"). You should know what type of exception you expect, and handle just that. So while the try
/catch
/finally
pattern would make it through code review (with comment), that aspect of the code would not.
Personally, I find the below structure clearer and it is the best practice we advise where I work.
Our Best Practice
SpecificException unexpectedException;
Test.startTest();
try
{
// logic here
}
catch (SpecificException e)
{
unexpectedException = e;
}
Test.stopTest();
system.assertEquals(null, unexpectedException, 'Informative message');
The reason we advise this pattern is that you should never put your test assertions within a conditional block, which executes only some of the time. Always make every assertion you intend. Note that if there is an exception, the assertion will show you a good level of detail.
There are variations of anti-pattern which violate this axiom but one typical example is below.
Anti-Pattern
Test.startTest();
try
{
// logic here
}
catch (SpecificException e)
{
system.assert(false, 'Informative message');
}
Test.stopTest();
This anti-pattern violates our axiom that each assertion should be unconditional.
The App
The pattern demonstrated in the app does avoid any violation of the axiom, so the pattern itself would probably pass our code review. However, it would be a marked improvement to cache the specific Exception
instance rather than a Boolean
flag, and in my opinion it is less clear to nest your blocks in this way.
One final note, we consider it firmly not best practice to catch generic Exception
also colloquially called a "pokemon catch"). You should know what type of exception you expect, and handle just that. So while the try
/catch
/finally
pattern would make it through code review (with comment), that aspect of the code would not.
answered 8 hours ago


Adrian Larson♦
104k19112235
104k19112235
Interesting theory, but why do you advise this over just not using try-catch at all and just let the exception fail the test directly? This gives you an immediate error and stacktrace without the extra assertions, etc. I'd rather focus on writing assertions that prove everything worked successfully rather than checking for specific exceptions that we might get.
– sfdcfox
8 hours ago
Also, "This anti-pattern violates our axiom that each assertion should be unconditional." Don't you mean "conditional"?
– sfdcfox
8 hours ago
We've been over this already in comments on other threads. We contend that tests should only fail via assertion. I know you disagree.
– Adrian Larson♦
7 hours ago
Sorry, I must have forgot. I've had a lot on my mind recently.
– sfdcfox
7 hours ago
1
Haha no offense meant. It's just a bit of a standing dispute between us (though I think we agree to disagree).
– Adrian Larson♦
7 hours ago
add a comment |
Interesting theory, but why do you advise this over just not using try-catch at all and just let the exception fail the test directly? This gives you an immediate error and stacktrace without the extra assertions, etc. I'd rather focus on writing assertions that prove everything worked successfully rather than checking for specific exceptions that we might get.
– sfdcfox
8 hours ago
Also, "This anti-pattern violates our axiom that each assertion should be unconditional." Don't you mean "conditional"?
– sfdcfox
8 hours ago
We've been over this already in comments on other threads. We contend that tests should only fail via assertion. I know you disagree.
– Adrian Larson♦
7 hours ago
Sorry, I must have forgot. I've had a lot on my mind recently.
– sfdcfox
7 hours ago
1
Haha no offense meant. It's just a bit of a standing dispute between us (though I think we agree to disagree).
– Adrian Larson♦
7 hours ago
Interesting theory, but why do you advise this over just not using try-catch at all and just let the exception fail the test directly? This gives you an immediate error and stacktrace without the extra assertions, etc. I'd rather focus on writing assertions that prove everything worked successfully rather than checking for specific exceptions that we might get.
– sfdcfox
8 hours ago
Interesting theory, but why do you advise this over just not using try-catch at all and just let the exception fail the test directly? This gives you an immediate error and stacktrace without the extra assertions, etc. I'd rather focus on writing assertions that prove everything worked successfully rather than checking for specific exceptions that we might get.
– sfdcfox
8 hours ago
Also, "This anti-pattern violates our axiom that each assertion should be unconditional." Don't you mean "conditional"?
– sfdcfox
8 hours ago
Also, "This anti-pattern violates our axiom that each assertion should be unconditional." Don't you mean "conditional"?
– sfdcfox
8 hours ago
We've been over this already in comments on other threads. We contend that tests should only fail via assertion. I know you disagree.
– Adrian Larson♦
7 hours ago
We've been over this already in comments on other threads. We contend that tests should only fail via assertion. I know you disagree.
– Adrian Larson♦
7 hours ago
Sorry, I must have forgot. I've had a lot on my mind recently.
– sfdcfox
7 hours ago
Sorry, I must have forgot. I've had a lot on my mind recently.
– sfdcfox
7 hours ago
1
1
Haha no offense meant. It's just a bit of a standing dispute between us (though I think we agree to disagree).
– Adrian Larson♦
7 hours ago
Haha no offense meant. It's just a bit of a standing dispute between us (though I think we agree to disagree).
– Adrian Larson♦
7 hours ago
add a comment |
Thanks for contributing an answer to Salesforce Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsalesforce.stackexchange.com%2fquestions%2f243730%2fusing-try-catch-and-finally-in-test-class-dreamhouseapp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BqZu,A1ES51GCUPIdpdCeaMbUjj,sCl6VPVjVxqCjx8Ug HcJppVF3fRm uywMzB ers 5,EuZw,4zdPQqjaW
2
I'm curious to see if there's an answer to the contrary, but I don't see the advantage to that structure or think it's best practice. I'd call those smoke tests myself; the assertion proves nothing and actually hides an exception that might have included valuable information.
– David Reed
9 hours ago