Partial Maven clean install after Git pull
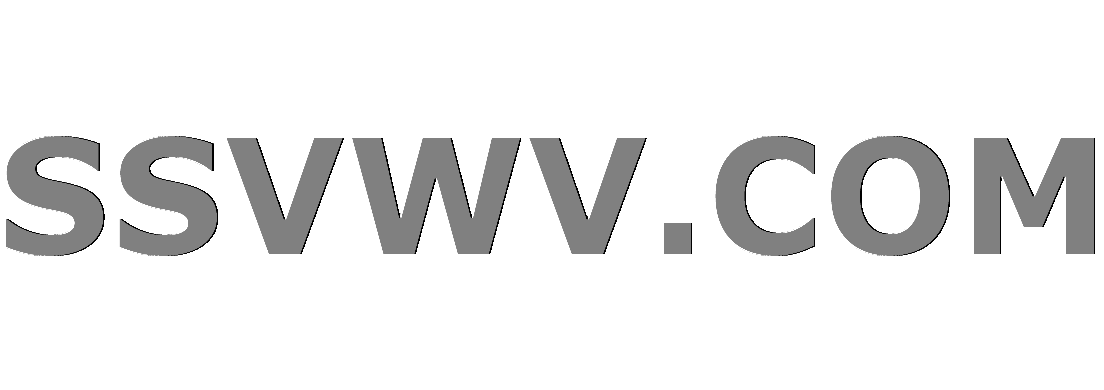
Multi tool use
up vote
2
down vote
favorite
I had searched for a solution to mvn clean install
on my changed projects after a git pull
. I even asked a question over at SO about the same thing. The answer I got pointed me into creating my own script to achieve what I wanted to accomplish. So I went, I saw, and I conquered the script that I wanted. However, it is probably not efficient, and could use refactoring. I am new to bash scripting and learned a bit while doing this.
The structure I was targeting is a Maven POM Project. That project contains several sub-POM Projects, which in turn contain Maven Java Projects.
Example Structure:
-Parent
|-Sub_Parent_A
|-Child_1
|-Child_2
|-Sub_Parent_B
|-Child_3
|-Child_4
I wanted to only call mvn clean install
if a project had files that changed in the child projects. This would reduce time on going to each project by hand and calling the command or by just calling the command on the parent project.
The code can also be found on GitHub
################################################################################
#
# License:.....GNU General Public License v3.0
# Author:......CodeMonkey
# Date:........14 November 2018
# Title:.......GitMavenCleanInstall.sh
# Description: This script is designed to cd to a set Maven POM Project,
# perform a git remote update and pull, and clean install the changed
# files projects.
# Notice:......The project structure this script was originally set to target
# is structured as a Maven POM Project that contains several sub-POM Projects.
# The sub-POM Projects contain Maven Java Application projects. The targets
# should be easy to change, and allow for others to target other structures.
#
################################################################################
#
# Change History: N/A
#
################################################################################
#!/bin/bash
#Function to check if array has element
containsElement () {
local e match="$1"
shift
for e; do [[ "$e" == "$match" ]] && return 0; done
return 1
}
#Navigate to the POM Project
cd PATH/TO/POM/PROJECT
#Remote update
git remote update -p
#Pull
git pull
#Get the current working branch
CURRENT_BRANCH="$(git branch | sed -n -e 's/^* (.*)/1/p')"
#Get the output of the command git diff
GIT_DIFF_OUTPUT="$(git diff --name-status HEAD@{1} ${CURRENT_BRANCH})"
#Split the diff output into an array
read -a GIT_DIFF_OUTPUT_ARY <<< $GIT_DIF_OUTPUT
#Declare empty array for root path
declare -a GIT_DIFF_OUTPUT_ARY_ROOT_PATH=()
FORWARD='/'
#Loop diff output array
for i in "$GIT_DIFF_OUTPUT_ARY[@]}"
do
#Check that the string is not 1 Character
if [[ "$(echo -n $1 | wc -m)" != 1 ]]
then
#Split the file path by /
IFS='/' read -ra SPLIT <<< $i
#Concatenate first path + / + second path
path=${SPLIT[0]}$FORWARD${SPLIT[1]}
#Call function to see if it already exists in the root path array
containsElement "$path" "${GIT_DIFF_OUTPUT_ARY_ROOT_PATH[@]}"
if [[ $? != 0 ]]
then
#Add the path since it was not found
GIT_DIFF_OUTPUT_ARY_ROOT_PATH+=($path)
fi
fi
done
#Loop root path array
for val in ${GIT_DIFF_OUTPUT_ARY_ROOT_PATH[@]}
do
#CD into root path
cd $val
#Maven call to clean install
mvn -DskipTests=true --errors -T 8 -e clean install
#CD back up before next project
cd ../../
done
bash git installer
add a comment |
up vote
2
down vote
favorite
I had searched for a solution to mvn clean install
on my changed projects after a git pull
. I even asked a question over at SO about the same thing. The answer I got pointed me into creating my own script to achieve what I wanted to accomplish. So I went, I saw, and I conquered the script that I wanted. However, it is probably not efficient, and could use refactoring. I am new to bash scripting and learned a bit while doing this.
The structure I was targeting is a Maven POM Project. That project contains several sub-POM Projects, which in turn contain Maven Java Projects.
Example Structure:
-Parent
|-Sub_Parent_A
|-Child_1
|-Child_2
|-Sub_Parent_B
|-Child_3
|-Child_4
I wanted to only call mvn clean install
if a project had files that changed in the child projects. This would reduce time on going to each project by hand and calling the command or by just calling the command on the parent project.
The code can also be found on GitHub
################################################################################
#
# License:.....GNU General Public License v3.0
# Author:......CodeMonkey
# Date:........14 November 2018
# Title:.......GitMavenCleanInstall.sh
# Description: This script is designed to cd to a set Maven POM Project,
# perform a git remote update and pull, and clean install the changed
# files projects.
# Notice:......The project structure this script was originally set to target
# is structured as a Maven POM Project that contains several sub-POM Projects.
# The sub-POM Projects contain Maven Java Application projects. The targets
# should be easy to change, and allow for others to target other structures.
#
################################################################################
#
# Change History: N/A
#
################################################################################
#!/bin/bash
#Function to check if array has element
containsElement () {
local e match="$1"
shift
for e; do [[ "$e" == "$match" ]] && return 0; done
return 1
}
#Navigate to the POM Project
cd PATH/TO/POM/PROJECT
#Remote update
git remote update -p
#Pull
git pull
#Get the current working branch
CURRENT_BRANCH="$(git branch | sed -n -e 's/^* (.*)/1/p')"
#Get the output of the command git diff
GIT_DIFF_OUTPUT="$(git diff --name-status HEAD@{1} ${CURRENT_BRANCH})"
#Split the diff output into an array
read -a GIT_DIFF_OUTPUT_ARY <<< $GIT_DIF_OUTPUT
#Declare empty array for root path
declare -a GIT_DIFF_OUTPUT_ARY_ROOT_PATH=()
FORWARD='/'
#Loop diff output array
for i in "$GIT_DIFF_OUTPUT_ARY[@]}"
do
#Check that the string is not 1 Character
if [[ "$(echo -n $1 | wc -m)" != 1 ]]
then
#Split the file path by /
IFS='/' read -ra SPLIT <<< $i
#Concatenate first path + / + second path
path=${SPLIT[0]}$FORWARD${SPLIT[1]}
#Call function to see if it already exists in the root path array
containsElement "$path" "${GIT_DIFF_OUTPUT_ARY_ROOT_PATH[@]}"
if [[ $? != 0 ]]
then
#Add the path since it was not found
GIT_DIFF_OUTPUT_ARY_ROOT_PATH+=($path)
fi
fi
done
#Loop root path array
for val in ${GIT_DIFF_OUTPUT_ARY_ROOT_PATH[@]}
do
#CD into root path
cd $val
#Maven call to clean install
mvn -DskipTests=true --errors -T 8 -e clean install
#CD back up before next project
cd ../../
done
bash git installer
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I had searched for a solution to mvn clean install
on my changed projects after a git pull
. I even asked a question over at SO about the same thing. The answer I got pointed me into creating my own script to achieve what I wanted to accomplish. So I went, I saw, and I conquered the script that I wanted. However, it is probably not efficient, and could use refactoring. I am new to bash scripting and learned a bit while doing this.
The structure I was targeting is a Maven POM Project. That project contains several sub-POM Projects, which in turn contain Maven Java Projects.
Example Structure:
-Parent
|-Sub_Parent_A
|-Child_1
|-Child_2
|-Sub_Parent_B
|-Child_3
|-Child_4
I wanted to only call mvn clean install
if a project had files that changed in the child projects. This would reduce time on going to each project by hand and calling the command or by just calling the command on the parent project.
The code can also be found on GitHub
################################################################################
#
# License:.....GNU General Public License v3.0
# Author:......CodeMonkey
# Date:........14 November 2018
# Title:.......GitMavenCleanInstall.sh
# Description: This script is designed to cd to a set Maven POM Project,
# perform a git remote update and pull, and clean install the changed
# files projects.
# Notice:......The project structure this script was originally set to target
# is structured as a Maven POM Project that contains several sub-POM Projects.
# The sub-POM Projects contain Maven Java Application projects. The targets
# should be easy to change, and allow for others to target other structures.
#
################################################################################
#
# Change History: N/A
#
################################################################################
#!/bin/bash
#Function to check if array has element
containsElement () {
local e match="$1"
shift
for e; do [[ "$e" == "$match" ]] && return 0; done
return 1
}
#Navigate to the POM Project
cd PATH/TO/POM/PROJECT
#Remote update
git remote update -p
#Pull
git pull
#Get the current working branch
CURRENT_BRANCH="$(git branch | sed -n -e 's/^* (.*)/1/p')"
#Get the output of the command git diff
GIT_DIFF_OUTPUT="$(git diff --name-status HEAD@{1} ${CURRENT_BRANCH})"
#Split the diff output into an array
read -a GIT_DIFF_OUTPUT_ARY <<< $GIT_DIF_OUTPUT
#Declare empty array for root path
declare -a GIT_DIFF_OUTPUT_ARY_ROOT_PATH=()
FORWARD='/'
#Loop diff output array
for i in "$GIT_DIFF_OUTPUT_ARY[@]}"
do
#Check that the string is not 1 Character
if [[ "$(echo -n $1 | wc -m)" != 1 ]]
then
#Split the file path by /
IFS='/' read -ra SPLIT <<< $i
#Concatenate first path + / + second path
path=${SPLIT[0]}$FORWARD${SPLIT[1]}
#Call function to see if it already exists in the root path array
containsElement "$path" "${GIT_DIFF_OUTPUT_ARY_ROOT_PATH[@]}"
if [[ $? != 0 ]]
then
#Add the path since it was not found
GIT_DIFF_OUTPUT_ARY_ROOT_PATH+=($path)
fi
fi
done
#Loop root path array
for val in ${GIT_DIFF_OUTPUT_ARY_ROOT_PATH[@]}
do
#CD into root path
cd $val
#Maven call to clean install
mvn -DskipTests=true --errors -T 8 -e clean install
#CD back up before next project
cd ../../
done
bash git installer
I had searched for a solution to mvn clean install
on my changed projects after a git pull
. I even asked a question over at SO about the same thing. The answer I got pointed me into creating my own script to achieve what I wanted to accomplish. So I went, I saw, and I conquered the script that I wanted. However, it is probably not efficient, and could use refactoring. I am new to bash scripting and learned a bit while doing this.
The structure I was targeting is a Maven POM Project. That project contains several sub-POM Projects, which in turn contain Maven Java Projects.
Example Structure:
-Parent
|-Sub_Parent_A
|-Child_1
|-Child_2
|-Sub_Parent_B
|-Child_3
|-Child_4
I wanted to only call mvn clean install
if a project had files that changed in the child projects. This would reduce time on going to each project by hand and calling the command or by just calling the command on the parent project.
The code can also be found on GitHub
################################################################################
#
# License:.....GNU General Public License v3.0
# Author:......CodeMonkey
# Date:........14 November 2018
# Title:.......GitMavenCleanInstall.sh
# Description: This script is designed to cd to a set Maven POM Project,
# perform a git remote update and pull, and clean install the changed
# files projects.
# Notice:......The project structure this script was originally set to target
# is structured as a Maven POM Project that contains several sub-POM Projects.
# The sub-POM Projects contain Maven Java Application projects. The targets
# should be easy to change, and allow for others to target other structures.
#
################################################################################
#
# Change History: N/A
#
################################################################################
#!/bin/bash
#Function to check if array has element
containsElement () {
local e match="$1"
shift
for e; do [[ "$e" == "$match" ]] && return 0; done
return 1
}
#Navigate to the POM Project
cd PATH/TO/POM/PROJECT
#Remote update
git remote update -p
#Pull
git pull
#Get the current working branch
CURRENT_BRANCH="$(git branch | sed -n -e 's/^* (.*)/1/p')"
#Get the output of the command git diff
GIT_DIFF_OUTPUT="$(git diff --name-status HEAD@{1} ${CURRENT_BRANCH})"
#Split the diff output into an array
read -a GIT_DIFF_OUTPUT_ARY <<< $GIT_DIF_OUTPUT
#Declare empty array for root path
declare -a GIT_DIFF_OUTPUT_ARY_ROOT_PATH=()
FORWARD='/'
#Loop diff output array
for i in "$GIT_DIFF_OUTPUT_ARY[@]}"
do
#Check that the string is not 1 Character
if [[ "$(echo -n $1 | wc -m)" != 1 ]]
then
#Split the file path by /
IFS='/' read -ra SPLIT <<< $i
#Concatenate first path + / + second path
path=${SPLIT[0]}$FORWARD${SPLIT[1]}
#Call function to see if it already exists in the root path array
containsElement "$path" "${GIT_DIFF_OUTPUT_ARY_ROOT_PATH[@]}"
if [[ $? != 0 ]]
then
#Add the path since it was not found
GIT_DIFF_OUTPUT_ARY_ROOT_PATH+=($path)
fi
fi
done
#Loop root path array
for val in ${GIT_DIFF_OUTPUT_ARY_ROOT_PATH[@]}
do
#CD into root path
cd $val
#Maven call to clean install
mvn -DskipTests=true --errors -T 8 -e clean install
#CD back up before next project
cd ../../
done
bash git installer
bash git installer
edited yesterday
asked yesterday


CodeMonkey
264139
264139
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f207685%2fpartial-maven-clean-install-after-git-pull%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WGLd fnYF,PpVFKY8UW3i8tzNpjx37,g3uoVwSgoDAF W5pdM5,P05VwyT5V,osSwqhW3IHN6fd5CtP 2xf04At iETsZk J cVXLG fAfu8