python3 multiprocessing queue deadlock when use thread and process at same time
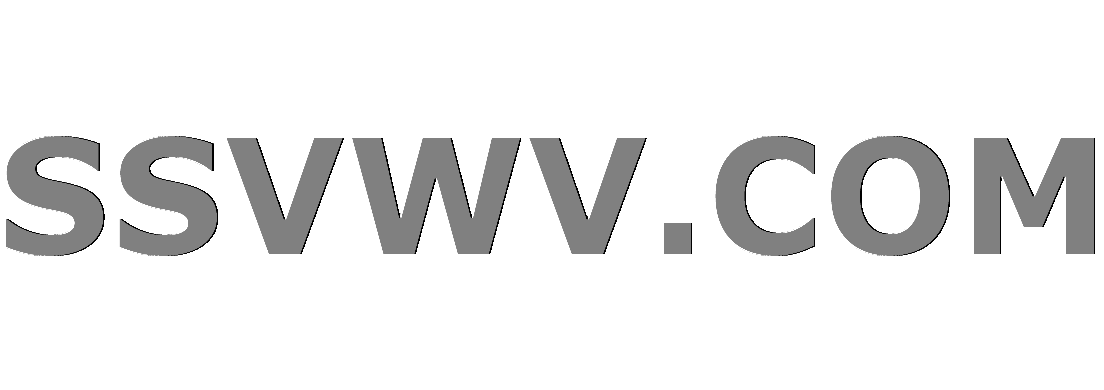
Multi tool use
I used multi-processes to handle cpu intensive task,I have a thread reading data from stdin and put it to a input_queue, a thread get data from output_queue and write it to stdout, multiple processes get data from input queue,then handled the data,and put it to output_queue.But It some times will block forever,I doubt that it was because inappropriate to use the multiprocessing Queue,But I don't know how to solved it,can anyone help me? my code as follows:
import multiprocessing
import sys
import threading
import time
from multiprocessing import Queue
def write_to_stdout(result_queue: Queue):
"""write queue data to stdout"""
while True:
data = result_queue.get()
if data is StopIteration:
break
sys.stdout.write(data)
sys.stdout.flush()
def read_from_stdin(queue):
"""read data from stdin, put it in queue for process handling"""
try:
for line in sys.stdin:
queue.put(line)
finally:
queue.put(StopIteration)
def process_func(input_queue, result_queue):
"""get data from input_queue,handled,put result into result_queue"""
try:
while True:
data = input_queue.get()
if data is StopIteration:
break
# cpu intensive task,use time.sleep instead
# result = compute_something(data)
time.sleep(0.1)
result_queue.put(data)
finally:
# ensure every process end
input_queue.put(StopIteration)
if __name__ == '__main__':
# queue for reading to stdout
input_queue = Queue(1000)
# queue for writing to stdout
result_queue = Queue(1000)
# thread reading data from stdin
input_thread = threading.Thread(target=read_from_stdin, args=(input_queue,))
input_thread.start()
# thread reading data from stdin
output_thread = threading.Thread(target=write_to_stdout, args=(result_queue,))
output_thread.start()
processes =
cpu_count = multiprocessing.cpu_count()
# start multi-process to handle some cpu intensive task
for i in range(cpu_count):
proc = multiprocessing.Process(target=process_func, args=(input_queue, result_queue))
proc.start()
processes.append(proc)
# joined input thread
input_thread.join()
# joined all task processes
for proc in processes:
proc.join()
# ensure output thread end
result_queue.put(StopIteration)
# joined output thread
output_thread.join()
test environment:
python3.6
ubuntu16.04 lts
python multiprocessing
New contributor
白稳平 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I used multi-processes to handle cpu intensive task,I have a thread reading data from stdin and put it to a input_queue, a thread get data from output_queue and write it to stdout, multiple processes get data from input queue,then handled the data,and put it to output_queue.But It some times will block forever,I doubt that it was because inappropriate to use the multiprocessing Queue,But I don't know how to solved it,can anyone help me? my code as follows:
import multiprocessing
import sys
import threading
import time
from multiprocessing import Queue
def write_to_stdout(result_queue: Queue):
"""write queue data to stdout"""
while True:
data = result_queue.get()
if data is StopIteration:
break
sys.stdout.write(data)
sys.stdout.flush()
def read_from_stdin(queue):
"""read data from stdin, put it in queue for process handling"""
try:
for line in sys.stdin:
queue.put(line)
finally:
queue.put(StopIteration)
def process_func(input_queue, result_queue):
"""get data from input_queue,handled,put result into result_queue"""
try:
while True:
data = input_queue.get()
if data is StopIteration:
break
# cpu intensive task,use time.sleep instead
# result = compute_something(data)
time.sleep(0.1)
result_queue.put(data)
finally:
# ensure every process end
input_queue.put(StopIteration)
if __name__ == '__main__':
# queue for reading to stdout
input_queue = Queue(1000)
# queue for writing to stdout
result_queue = Queue(1000)
# thread reading data from stdin
input_thread = threading.Thread(target=read_from_stdin, args=(input_queue,))
input_thread.start()
# thread reading data from stdin
output_thread = threading.Thread(target=write_to_stdout, args=(result_queue,))
output_thread.start()
processes =
cpu_count = multiprocessing.cpu_count()
# start multi-process to handle some cpu intensive task
for i in range(cpu_count):
proc = multiprocessing.Process(target=process_func, args=(input_queue, result_queue))
proc.start()
processes.append(proc)
# joined input thread
input_thread.join()
# joined all task processes
for proc in processes:
proc.join()
# ensure output thread end
result_queue.put(StopIteration)
# joined output thread
output_thread.join()
test environment:
python3.6
ubuntu16.04 lts
python multiprocessing
New contributor
白稳平 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
If your code is not working, it is an offtopic question. Ask how to fix broken code at StackOverflow.
– mickmackusa
4 mins ago
add a comment |
I used multi-processes to handle cpu intensive task,I have a thread reading data from stdin and put it to a input_queue, a thread get data from output_queue and write it to stdout, multiple processes get data from input queue,then handled the data,and put it to output_queue.But It some times will block forever,I doubt that it was because inappropriate to use the multiprocessing Queue,But I don't know how to solved it,can anyone help me? my code as follows:
import multiprocessing
import sys
import threading
import time
from multiprocessing import Queue
def write_to_stdout(result_queue: Queue):
"""write queue data to stdout"""
while True:
data = result_queue.get()
if data is StopIteration:
break
sys.stdout.write(data)
sys.stdout.flush()
def read_from_stdin(queue):
"""read data from stdin, put it in queue for process handling"""
try:
for line in sys.stdin:
queue.put(line)
finally:
queue.put(StopIteration)
def process_func(input_queue, result_queue):
"""get data from input_queue,handled,put result into result_queue"""
try:
while True:
data = input_queue.get()
if data is StopIteration:
break
# cpu intensive task,use time.sleep instead
# result = compute_something(data)
time.sleep(0.1)
result_queue.put(data)
finally:
# ensure every process end
input_queue.put(StopIteration)
if __name__ == '__main__':
# queue for reading to stdout
input_queue = Queue(1000)
# queue for writing to stdout
result_queue = Queue(1000)
# thread reading data from stdin
input_thread = threading.Thread(target=read_from_stdin, args=(input_queue,))
input_thread.start()
# thread reading data from stdin
output_thread = threading.Thread(target=write_to_stdout, args=(result_queue,))
output_thread.start()
processes =
cpu_count = multiprocessing.cpu_count()
# start multi-process to handle some cpu intensive task
for i in range(cpu_count):
proc = multiprocessing.Process(target=process_func, args=(input_queue, result_queue))
proc.start()
processes.append(proc)
# joined input thread
input_thread.join()
# joined all task processes
for proc in processes:
proc.join()
# ensure output thread end
result_queue.put(StopIteration)
# joined output thread
output_thread.join()
test environment:
python3.6
ubuntu16.04 lts
python multiprocessing
New contributor
白稳平 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I used multi-processes to handle cpu intensive task,I have a thread reading data from stdin and put it to a input_queue, a thread get data from output_queue and write it to stdout, multiple processes get data from input queue,then handled the data,and put it to output_queue.But It some times will block forever,I doubt that it was because inappropriate to use the multiprocessing Queue,But I don't know how to solved it,can anyone help me? my code as follows:
import multiprocessing
import sys
import threading
import time
from multiprocessing import Queue
def write_to_stdout(result_queue: Queue):
"""write queue data to stdout"""
while True:
data = result_queue.get()
if data is StopIteration:
break
sys.stdout.write(data)
sys.stdout.flush()
def read_from_stdin(queue):
"""read data from stdin, put it in queue for process handling"""
try:
for line in sys.stdin:
queue.put(line)
finally:
queue.put(StopIteration)
def process_func(input_queue, result_queue):
"""get data from input_queue,handled,put result into result_queue"""
try:
while True:
data = input_queue.get()
if data is StopIteration:
break
# cpu intensive task,use time.sleep instead
# result = compute_something(data)
time.sleep(0.1)
result_queue.put(data)
finally:
# ensure every process end
input_queue.put(StopIteration)
if __name__ == '__main__':
# queue for reading to stdout
input_queue = Queue(1000)
# queue for writing to stdout
result_queue = Queue(1000)
# thread reading data from stdin
input_thread = threading.Thread(target=read_from_stdin, args=(input_queue,))
input_thread.start()
# thread reading data from stdin
output_thread = threading.Thread(target=write_to_stdout, args=(result_queue,))
output_thread.start()
processes =
cpu_count = multiprocessing.cpu_count()
# start multi-process to handle some cpu intensive task
for i in range(cpu_count):
proc = multiprocessing.Process(target=process_func, args=(input_queue, result_queue))
proc.start()
processes.append(proc)
# joined input thread
input_thread.join()
# joined all task processes
for proc in processes:
proc.join()
# ensure output thread end
result_queue.put(StopIteration)
# joined output thread
output_thread.join()
test environment:
python3.6
ubuntu16.04 lts
python multiprocessing
python multiprocessing
New contributor
白稳平 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
白稳平 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
白稳平 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 7 mins ago
白稳平
1
1
New contributor
白稳平 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
白稳平 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
白稳平 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
If your code is not working, it is an offtopic question. Ask how to fix broken code at StackOverflow.
– mickmackusa
4 mins ago
add a comment |
If your code is not working, it is an offtopic question. Ask how to fix broken code at StackOverflow.
– mickmackusa
4 mins ago
If your code is not working, it is an offtopic question. Ask how to fix broken code at StackOverflow.
– mickmackusa
4 mins ago
If your code is not working, it is an offtopic question. Ask how to fix broken code at StackOverflow.
– mickmackusa
4 mins ago
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
白稳平 is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f210536%2fpython3-multiprocessing-queue-deadlock-when-use-thread-and-process-at-same-time%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
白稳平 is a new contributor. Be nice, and check out our Code of Conduct.
白稳平 is a new contributor. Be nice, and check out our Code of Conduct.
白稳平 is a new contributor. Be nice, and check out our Code of Conduct.
白稳平 is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f210536%2fpython3-multiprocessing-queue-deadlock-when-use-thread-and-process-at-same-time%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0,u1eZ9X1dx,B k6Fy7,Zknk8O3aJvYEoC,6,eECH,PU,InbhNxAq Rhdi,9Elg3ubay7C0sNV9g 6o InUUwH,Z1kGK6A,Y8uXY iYIPxf
If your code is not working, it is an offtopic question. Ask how to fix broken code at StackOverflow.
– mickmackusa
4 mins ago