Azure Blob and Table storage
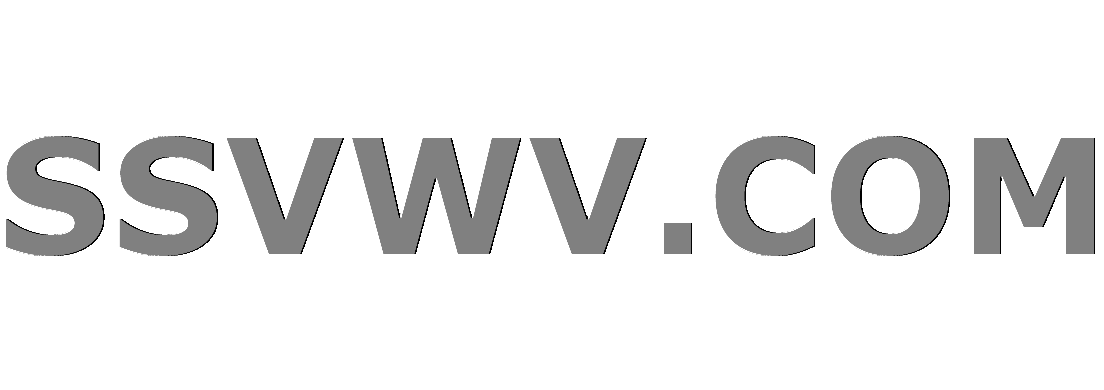
Multi tool use
up vote
0
down vote
favorite
I wrote this code, but is not very practical. I'm still new in this area of coding. Basically, I'm storing an image into a blob container, and I'm saving the URL in a table. I'm doing the same thing for a text file. I would like to use dependency injection for azure connection part to make my code more practical.
Here's my Logo Controller:
[Route("api/manage/logo")]
[ApiController]
public class ManageLogoController : ControllerBase
{
[HttpPost("{version}")]
public async Task<IActionResult> Post(IFormFile image, float version)
{
if (image.Length >= 1048576)
{
return BadRequest("Uploaded image may not exceed 1Mb, please upload a smaller image.");
}
var allowedExtensions = new {
".png", ".jpg", "jpeg" };
string fileExt = Path.GetExtension(image.FileName);
if (allowedExtensions.Contains(fileExt))
{
try
{
await LogoStorage.UploadFileToBlobStorage(version, image.FileName);
return Ok(new
{
lenght = image.Length,
name = image.FileName
});
}
catch (Exception ex)
{
return BadRequest();
}
}
TnC Controller
[Route("api/manage/tnc")]
[ApiController]
public class ManageTermCondController : ControllerBase
{
[HttpPost("{version}")]
public async Task<IActionResult> Post(IFormFile doc, float version)
{
var allowedExtension = ".txt";
string fileExt = Path.GetExtension(doc.FileName);
if (allowedExtension.Contains(fileExt))
{
try
{
await TncStorage.UploadDocToBlobStorage(version, doc.FileName);
return Ok(new
{
lenght = doc.Length,
name = doc.FileName
});
}
catch (Exception ex)
{
return BadRequest();
}
}
else
{
return BadRequest("Only .txt files are allowed!");
}
}
[HttpGet]
public string Geti(float version)
{
var x = TncStorage.GetURL(version);
if (x == null)
{
return "There's no such record";
}
else return TncStorage.GetURL(version);
}
}
I made a new class library named .Service for the following part:
public class VersionURL : TableEntity
{
public VersionURL(string type, string version)
{
PartitionKey = type;
RowKey = version;
}
public VersionURL() { }
public string URL { get; set; }
public string ETag { get; set; }
}
The first class:
public static async Task UploadFileToBlobStorage(float version, string filename)
{
CloudStorageAccount storageAccount = null;
CloudBlobContainer cloudBlobContainer = null;
string storageConnectionString = "";
if (CloudStorageAccount.TryParse(storageConnectionString, out storageAccount))
{
try
{
CloudBlobClient cloudBlobClient = storageAccount.CreateCloudBlobClient();
cloudBlobContainer = cloudBlobClient.GetContainerReference("logodata");
await cloudBlobContainer.CreateIfNotExistsAsync();
BlobContainerPermissions permissions = new BlobContainerPermissions
{
PublicAccess = BlobContainerPublicAccessType.Blob
};
await cloudBlobContainer.SetPermissionsAsync(permissions);
var blobUrl = "";
string file = Guid.NewGuid().ToString() + Path.GetExtension(filename);
CloudBlockBlob cloudBlockBlob = cloudBlobContainer.GetBlockBlobReference(file);
try
{
await cloudBlockBlob.UploadFromFileAsync(filename);
}
catch (Exception ex)
{
}
blobUrl = cloudBlockBlob.Uri.AbsoluteUri;
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
await table.CreateIfNotExistsAsync();
var v = "v" + version;
VersionURL content = new VersionURL("Logo", v);
content.ETag = "*";
content.URL = blobUrl;
var query = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
TableContinuationToken continuationToken = null;
do
{
var result = await table.ExecuteQuerySegmentedAsync(query, continuationToken);
continuationToken = result.ContinuationToken;
if (result.Count() != 0)
{
foreach (VersionURL entity in result)
{
if (entity.RowKey == v)
{
try
{
TableOperation updateOperation = TableOperation.Merge(content);
await table.ExecuteAsync(updateOperation);
}
catch (Exception ex)
{
}
}
}
}
else
{
TableOperation insertOperation = TableOperation.Insert(content);
await table.ExecuteAsync(insertOperation);
}
} while (continuationToken != null);
}
catch (StorageException ex)
{
Console.WriteLine("Error returned from the service: {0}", ex.Message);
}
finally
{
}
}
else
{
Console.WriteLine(
"A connection string has not been defined in the system environment variables. " +
"Add a environment variable named 'storageconnectionstring' with your storage " +
"connection string as a value.");
}
}
}
The second class:
public class TncStorage
{
public static async Task UploadDocToBlobStorage(float version, string filename)
{
CloudStorageAccount storageAccount = null;
CloudBlobContainer cloudBlobContainer = null;
string storageConnectionString = "";
if (CloudStorageAccount.TryParse(storageConnectionString, out storageAccount))
{
try
{
CloudBlobClient cloudBlobClient = storageAccount.CreateCloudBlobClient();
cloudBlobContainer = cloudBlobClient.GetContainerReference("tncdata");
await cloudBlobContainer.CreateIfNotExistsAsync();
BlobContainerPermissions permissions = new BlobContainerPermissions
{
PublicAccess = BlobContainerPublicAccessType.Blob
};
await cloudBlobContainer.SetPermissionsAsync(permissions);
var blobUrl = "";
string file = Guid.NewGuid().ToString() + Path.GetExtension(filename);
CloudBlockBlob cloudBlockBlob = cloudBlobContainer.GetBlockBlobReference(file);
try
{
await cloudBlockBlob.UploadFromFileAsync(filename);
}
catch (Exception ex)
{
}
blobUrl = cloudBlockBlob.Uri.AbsoluteUri;
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
await table.CreateIfNotExistsAsync();
var v = "v" + version;
VersionURL content = new VersionURL("TnC", v);
content.ETag = "*";
content.URL = blobUrl;
var query = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
TableContinuationToken continuationToken = null;
do
{
var result = await table.ExecuteQuerySegmentedAsync(query, continuationToken);
continuationToken = result.ContinuationToken;
if (result.Count() != 0)
{
foreach (VersionURL entity in result)
{
if (entity.RowKey == v)
{
try
{
var dec = version + .1;
content.RowKey = "v" + dec;
TableOperation updateOperation = TableOperation.Insert(content);
await table.ExecuteAsync(updateOperation);
}
catch (Exception ex)
{
}
}
}
}
else
{
TableOperation insertOperation = TableOperation.Insert(content);
await table.ExecuteAsync(insertOperation);
}
} while (continuationToken != null);
}
catch (StorageException ex)
{
Console.WriteLine("Error returned from the service: {0}", ex.Message);
}
finally
{
}
}
else
{
Console.WriteLine(
"A connection string has not been defined in the system environment variables. " +
"Add a environment variable named 'storageconnectionstring' with your storage " +
"connection string as a value.");
}
}
public static string GetURL(float version)
{
CloudStorageAccount storageAccount = null;
string storageConnectionString = "";
storageAccount = CloudStorageAccount.Parse(storageConnectionString);
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
var v = "v" + version;
var tableQuery = new TableQuery<VersionURL>();
tableQuery = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
var entities = table.ExecuteQuerySegmentedAsync(tableQuery, null);
var results = entities.Result;
var s = "";
foreach (var file in results.Where(x => x.RowKey == v))
{
//if (file.RowKey == v)
//{
return s = file.URL;
//}
//else
// return null;
}
return null;
}
How can I simplify the first and the second class?
c# asp.net-web-api azure
New contributor
T x is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
I wrote this code, but is not very practical. I'm still new in this area of coding. Basically, I'm storing an image into a blob container, and I'm saving the URL in a table. I'm doing the same thing for a text file. I would like to use dependency injection for azure connection part to make my code more practical.
Here's my Logo Controller:
[Route("api/manage/logo")]
[ApiController]
public class ManageLogoController : ControllerBase
{
[HttpPost("{version}")]
public async Task<IActionResult> Post(IFormFile image, float version)
{
if (image.Length >= 1048576)
{
return BadRequest("Uploaded image may not exceed 1Mb, please upload a smaller image.");
}
var allowedExtensions = new {
".png", ".jpg", "jpeg" };
string fileExt = Path.GetExtension(image.FileName);
if (allowedExtensions.Contains(fileExt))
{
try
{
await LogoStorage.UploadFileToBlobStorage(version, image.FileName);
return Ok(new
{
lenght = image.Length,
name = image.FileName
});
}
catch (Exception ex)
{
return BadRequest();
}
}
TnC Controller
[Route("api/manage/tnc")]
[ApiController]
public class ManageTermCondController : ControllerBase
{
[HttpPost("{version}")]
public async Task<IActionResult> Post(IFormFile doc, float version)
{
var allowedExtension = ".txt";
string fileExt = Path.GetExtension(doc.FileName);
if (allowedExtension.Contains(fileExt))
{
try
{
await TncStorage.UploadDocToBlobStorage(version, doc.FileName);
return Ok(new
{
lenght = doc.Length,
name = doc.FileName
});
}
catch (Exception ex)
{
return BadRequest();
}
}
else
{
return BadRequest("Only .txt files are allowed!");
}
}
[HttpGet]
public string Geti(float version)
{
var x = TncStorage.GetURL(version);
if (x == null)
{
return "There's no such record";
}
else return TncStorage.GetURL(version);
}
}
I made a new class library named .Service for the following part:
public class VersionURL : TableEntity
{
public VersionURL(string type, string version)
{
PartitionKey = type;
RowKey = version;
}
public VersionURL() { }
public string URL { get; set; }
public string ETag { get; set; }
}
The first class:
public static async Task UploadFileToBlobStorage(float version, string filename)
{
CloudStorageAccount storageAccount = null;
CloudBlobContainer cloudBlobContainer = null;
string storageConnectionString = "";
if (CloudStorageAccount.TryParse(storageConnectionString, out storageAccount))
{
try
{
CloudBlobClient cloudBlobClient = storageAccount.CreateCloudBlobClient();
cloudBlobContainer = cloudBlobClient.GetContainerReference("logodata");
await cloudBlobContainer.CreateIfNotExistsAsync();
BlobContainerPermissions permissions = new BlobContainerPermissions
{
PublicAccess = BlobContainerPublicAccessType.Blob
};
await cloudBlobContainer.SetPermissionsAsync(permissions);
var blobUrl = "";
string file = Guid.NewGuid().ToString() + Path.GetExtension(filename);
CloudBlockBlob cloudBlockBlob = cloudBlobContainer.GetBlockBlobReference(file);
try
{
await cloudBlockBlob.UploadFromFileAsync(filename);
}
catch (Exception ex)
{
}
blobUrl = cloudBlockBlob.Uri.AbsoluteUri;
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
await table.CreateIfNotExistsAsync();
var v = "v" + version;
VersionURL content = new VersionURL("Logo", v);
content.ETag = "*";
content.URL = blobUrl;
var query = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
TableContinuationToken continuationToken = null;
do
{
var result = await table.ExecuteQuerySegmentedAsync(query, continuationToken);
continuationToken = result.ContinuationToken;
if (result.Count() != 0)
{
foreach (VersionURL entity in result)
{
if (entity.RowKey == v)
{
try
{
TableOperation updateOperation = TableOperation.Merge(content);
await table.ExecuteAsync(updateOperation);
}
catch (Exception ex)
{
}
}
}
}
else
{
TableOperation insertOperation = TableOperation.Insert(content);
await table.ExecuteAsync(insertOperation);
}
} while (continuationToken != null);
}
catch (StorageException ex)
{
Console.WriteLine("Error returned from the service: {0}", ex.Message);
}
finally
{
}
}
else
{
Console.WriteLine(
"A connection string has not been defined in the system environment variables. " +
"Add a environment variable named 'storageconnectionstring' with your storage " +
"connection string as a value.");
}
}
}
The second class:
public class TncStorage
{
public static async Task UploadDocToBlobStorage(float version, string filename)
{
CloudStorageAccount storageAccount = null;
CloudBlobContainer cloudBlobContainer = null;
string storageConnectionString = "";
if (CloudStorageAccount.TryParse(storageConnectionString, out storageAccount))
{
try
{
CloudBlobClient cloudBlobClient = storageAccount.CreateCloudBlobClient();
cloudBlobContainer = cloudBlobClient.GetContainerReference("tncdata");
await cloudBlobContainer.CreateIfNotExistsAsync();
BlobContainerPermissions permissions = new BlobContainerPermissions
{
PublicAccess = BlobContainerPublicAccessType.Blob
};
await cloudBlobContainer.SetPermissionsAsync(permissions);
var blobUrl = "";
string file = Guid.NewGuid().ToString() + Path.GetExtension(filename);
CloudBlockBlob cloudBlockBlob = cloudBlobContainer.GetBlockBlobReference(file);
try
{
await cloudBlockBlob.UploadFromFileAsync(filename);
}
catch (Exception ex)
{
}
blobUrl = cloudBlockBlob.Uri.AbsoluteUri;
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
await table.CreateIfNotExistsAsync();
var v = "v" + version;
VersionURL content = new VersionURL("TnC", v);
content.ETag = "*";
content.URL = blobUrl;
var query = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
TableContinuationToken continuationToken = null;
do
{
var result = await table.ExecuteQuerySegmentedAsync(query, continuationToken);
continuationToken = result.ContinuationToken;
if (result.Count() != 0)
{
foreach (VersionURL entity in result)
{
if (entity.RowKey == v)
{
try
{
var dec = version + .1;
content.RowKey = "v" + dec;
TableOperation updateOperation = TableOperation.Insert(content);
await table.ExecuteAsync(updateOperation);
}
catch (Exception ex)
{
}
}
}
}
else
{
TableOperation insertOperation = TableOperation.Insert(content);
await table.ExecuteAsync(insertOperation);
}
} while (continuationToken != null);
}
catch (StorageException ex)
{
Console.WriteLine("Error returned from the service: {0}", ex.Message);
}
finally
{
}
}
else
{
Console.WriteLine(
"A connection string has not been defined in the system environment variables. " +
"Add a environment variable named 'storageconnectionstring' with your storage " +
"connection string as a value.");
}
}
public static string GetURL(float version)
{
CloudStorageAccount storageAccount = null;
string storageConnectionString = "";
storageAccount = CloudStorageAccount.Parse(storageConnectionString);
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
var v = "v" + version;
var tableQuery = new TableQuery<VersionURL>();
tableQuery = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
var entities = table.ExecuteQuerySegmentedAsync(tableQuery, null);
var results = entities.Result;
var s = "";
foreach (var file in results.Where(x => x.RowKey == v))
{
//if (file.RowKey == v)
//{
return s = file.URL;
//}
//else
// return null;
}
return null;
}
How can I simplify the first and the second class?
c# asp.net-web-api azure
New contributor
T x is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
5
The request for using dependency-injection is off-topic. We don't implement new features. You also need to better describe your code. The information at the top is very general. Please add more details about what each part is doing and if necessary also write something about how and why.
– t3chb0t
9 hours ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I wrote this code, but is not very practical. I'm still new in this area of coding. Basically, I'm storing an image into a blob container, and I'm saving the URL in a table. I'm doing the same thing for a text file. I would like to use dependency injection for azure connection part to make my code more practical.
Here's my Logo Controller:
[Route("api/manage/logo")]
[ApiController]
public class ManageLogoController : ControllerBase
{
[HttpPost("{version}")]
public async Task<IActionResult> Post(IFormFile image, float version)
{
if (image.Length >= 1048576)
{
return BadRequest("Uploaded image may not exceed 1Mb, please upload a smaller image.");
}
var allowedExtensions = new {
".png", ".jpg", "jpeg" };
string fileExt = Path.GetExtension(image.FileName);
if (allowedExtensions.Contains(fileExt))
{
try
{
await LogoStorage.UploadFileToBlobStorage(version, image.FileName);
return Ok(new
{
lenght = image.Length,
name = image.FileName
});
}
catch (Exception ex)
{
return BadRequest();
}
}
TnC Controller
[Route("api/manage/tnc")]
[ApiController]
public class ManageTermCondController : ControllerBase
{
[HttpPost("{version}")]
public async Task<IActionResult> Post(IFormFile doc, float version)
{
var allowedExtension = ".txt";
string fileExt = Path.GetExtension(doc.FileName);
if (allowedExtension.Contains(fileExt))
{
try
{
await TncStorage.UploadDocToBlobStorage(version, doc.FileName);
return Ok(new
{
lenght = doc.Length,
name = doc.FileName
});
}
catch (Exception ex)
{
return BadRequest();
}
}
else
{
return BadRequest("Only .txt files are allowed!");
}
}
[HttpGet]
public string Geti(float version)
{
var x = TncStorage.GetURL(version);
if (x == null)
{
return "There's no such record";
}
else return TncStorage.GetURL(version);
}
}
I made a new class library named .Service for the following part:
public class VersionURL : TableEntity
{
public VersionURL(string type, string version)
{
PartitionKey = type;
RowKey = version;
}
public VersionURL() { }
public string URL { get; set; }
public string ETag { get; set; }
}
The first class:
public static async Task UploadFileToBlobStorage(float version, string filename)
{
CloudStorageAccount storageAccount = null;
CloudBlobContainer cloudBlobContainer = null;
string storageConnectionString = "";
if (CloudStorageAccount.TryParse(storageConnectionString, out storageAccount))
{
try
{
CloudBlobClient cloudBlobClient = storageAccount.CreateCloudBlobClient();
cloudBlobContainer = cloudBlobClient.GetContainerReference("logodata");
await cloudBlobContainer.CreateIfNotExistsAsync();
BlobContainerPermissions permissions = new BlobContainerPermissions
{
PublicAccess = BlobContainerPublicAccessType.Blob
};
await cloudBlobContainer.SetPermissionsAsync(permissions);
var blobUrl = "";
string file = Guid.NewGuid().ToString() + Path.GetExtension(filename);
CloudBlockBlob cloudBlockBlob = cloudBlobContainer.GetBlockBlobReference(file);
try
{
await cloudBlockBlob.UploadFromFileAsync(filename);
}
catch (Exception ex)
{
}
blobUrl = cloudBlockBlob.Uri.AbsoluteUri;
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
await table.CreateIfNotExistsAsync();
var v = "v" + version;
VersionURL content = new VersionURL("Logo", v);
content.ETag = "*";
content.URL = blobUrl;
var query = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
TableContinuationToken continuationToken = null;
do
{
var result = await table.ExecuteQuerySegmentedAsync(query, continuationToken);
continuationToken = result.ContinuationToken;
if (result.Count() != 0)
{
foreach (VersionURL entity in result)
{
if (entity.RowKey == v)
{
try
{
TableOperation updateOperation = TableOperation.Merge(content);
await table.ExecuteAsync(updateOperation);
}
catch (Exception ex)
{
}
}
}
}
else
{
TableOperation insertOperation = TableOperation.Insert(content);
await table.ExecuteAsync(insertOperation);
}
} while (continuationToken != null);
}
catch (StorageException ex)
{
Console.WriteLine("Error returned from the service: {0}", ex.Message);
}
finally
{
}
}
else
{
Console.WriteLine(
"A connection string has not been defined in the system environment variables. " +
"Add a environment variable named 'storageconnectionstring' with your storage " +
"connection string as a value.");
}
}
}
The second class:
public class TncStorage
{
public static async Task UploadDocToBlobStorage(float version, string filename)
{
CloudStorageAccount storageAccount = null;
CloudBlobContainer cloudBlobContainer = null;
string storageConnectionString = "";
if (CloudStorageAccount.TryParse(storageConnectionString, out storageAccount))
{
try
{
CloudBlobClient cloudBlobClient = storageAccount.CreateCloudBlobClient();
cloudBlobContainer = cloudBlobClient.GetContainerReference("tncdata");
await cloudBlobContainer.CreateIfNotExistsAsync();
BlobContainerPermissions permissions = new BlobContainerPermissions
{
PublicAccess = BlobContainerPublicAccessType.Blob
};
await cloudBlobContainer.SetPermissionsAsync(permissions);
var blobUrl = "";
string file = Guid.NewGuid().ToString() + Path.GetExtension(filename);
CloudBlockBlob cloudBlockBlob = cloudBlobContainer.GetBlockBlobReference(file);
try
{
await cloudBlockBlob.UploadFromFileAsync(filename);
}
catch (Exception ex)
{
}
blobUrl = cloudBlockBlob.Uri.AbsoluteUri;
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
await table.CreateIfNotExistsAsync();
var v = "v" + version;
VersionURL content = new VersionURL("TnC", v);
content.ETag = "*";
content.URL = blobUrl;
var query = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
TableContinuationToken continuationToken = null;
do
{
var result = await table.ExecuteQuerySegmentedAsync(query, continuationToken);
continuationToken = result.ContinuationToken;
if (result.Count() != 0)
{
foreach (VersionURL entity in result)
{
if (entity.RowKey == v)
{
try
{
var dec = version + .1;
content.RowKey = "v" + dec;
TableOperation updateOperation = TableOperation.Insert(content);
await table.ExecuteAsync(updateOperation);
}
catch (Exception ex)
{
}
}
}
}
else
{
TableOperation insertOperation = TableOperation.Insert(content);
await table.ExecuteAsync(insertOperation);
}
} while (continuationToken != null);
}
catch (StorageException ex)
{
Console.WriteLine("Error returned from the service: {0}", ex.Message);
}
finally
{
}
}
else
{
Console.WriteLine(
"A connection string has not been defined in the system environment variables. " +
"Add a environment variable named 'storageconnectionstring' with your storage " +
"connection string as a value.");
}
}
public static string GetURL(float version)
{
CloudStorageAccount storageAccount = null;
string storageConnectionString = "";
storageAccount = CloudStorageAccount.Parse(storageConnectionString);
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
var v = "v" + version;
var tableQuery = new TableQuery<VersionURL>();
tableQuery = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
var entities = table.ExecuteQuerySegmentedAsync(tableQuery, null);
var results = entities.Result;
var s = "";
foreach (var file in results.Where(x => x.RowKey == v))
{
//if (file.RowKey == v)
//{
return s = file.URL;
//}
//else
// return null;
}
return null;
}
How can I simplify the first and the second class?
c# asp.net-web-api azure
New contributor
T x is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I wrote this code, but is not very practical. I'm still new in this area of coding. Basically, I'm storing an image into a blob container, and I'm saving the URL in a table. I'm doing the same thing for a text file. I would like to use dependency injection for azure connection part to make my code more practical.
Here's my Logo Controller:
[Route("api/manage/logo")]
[ApiController]
public class ManageLogoController : ControllerBase
{
[HttpPost("{version}")]
public async Task<IActionResult> Post(IFormFile image, float version)
{
if (image.Length >= 1048576)
{
return BadRequest("Uploaded image may not exceed 1Mb, please upload a smaller image.");
}
var allowedExtensions = new {
".png", ".jpg", "jpeg" };
string fileExt = Path.GetExtension(image.FileName);
if (allowedExtensions.Contains(fileExt))
{
try
{
await LogoStorage.UploadFileToBlobStorage(version, image.FileName);
return Ok(new
{
lenght = image.Length,
name = image.FileName
});
}
catch (Exception ex)
{
return BadRequest();
}
}
TnC Controller
[Route("api/manage/tnc")]
[ApiController]
public class ManageTermCondController : ControllerBase
{
[HttpPost("{version}")]
public async Task<IActionResult> Post(IFormFile doc, float version)
{
var allowedExtension = ".txt";
string fileExt = Path.GetExtension(doc.FileName);
if (allowedExtension.Contains(fileExt))
{
try
{
await TncStorage.UploadDocToBlobStorage(version, doc.FileName);
return Ok(new
{
lenght = doc.Length,
name = doc.FileName
});
}
catch (Exception ex)
{
return BadRequest();
}
}
else
{
return BadRequest("Only .txt files are allowed!");
}
}
[HttpGet]
public string Geti(float version)
{
var x = TncStorage.GetURL(version);
if (x == null)
{
return "There's no such record";
}
else return TncStorage.GetURL(version);
}
}
I made a new class library named .Service for the following part:
public class VersionURL : TableEntity
{
public VersionURL(string type, string version)
{
PartitionKey = type;
RowKey = version;
}
public VersionURL() { }
public string URL { get; set; }
public string ETag { get; set; }
}
The first class:
public static async Task UploadFileToBlobStorage(float version, string filename)
{
CloudStorageAccount storageAccount = null;
CloudBlobContainer cloudBlobContainer = null;
string storageConnectionString = "";
if (CloudStorageAccount.TryParse(storageConnectionString, out storageAccount))
{
try
{
CloudBlobClient cloudBlobClient = storageAccount.CreateCloudBlobClient();
cloudBlobContainer = cloudBlobClient.GetContainerReference("logodata");
await cloudBlobContainer.CreateIfNotExistsAsync();
BlobContainerPermissions permissions = new BlobContainerPermissions
{
PublicAccess = BlobContainerPublicAccessType.Blob
};
await cloudBlobContainer.SetPermissionsAsync(permissions);
var blobUrl = "";
string file = Guid.NewGuid().ToString() + Path.GetExtension(filename);
CloudBlockBlob cloudBlockBlob = cloudBlobContainer.GetBlockBlobReference(file);
try
{
await cloudBlockBlob.UploadFromFileAsync(filename);
}
catch (Exception ex)
{
}
blobUrl = cloudBlockBlob.Uri.AbsoluteUri;
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
await table.CreateIfNotExistsAsync();
var v = "v" + version;
VersionURL content = new VersionURL("Logo", v);
content.ETag = "*";
content.URL = blobUrl;
var query = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
TableContinuationToken continuationToken = null;
do
{
var result = await table.ExecuteQuerySegmentedAsync(query, continuationToken);
continuationToken = result.ContinuationToken;
if (result.Count() != 0)
{
foreach (VersionURL entity in result)
{
if (entity.RowKey == v)
{
try
{
TableOperation updateOperation = TableOperation.Merge(content);
await table.ExecuteAsync(updateOperation);
}
catch (Exception ex)
{
}
}
}
}
else
{
TableOperation insertOperation = TableOperation.Insert(content);
await table.ExecuteAsync(insertOperation);
}
} while (continuationToken != null);
}
catch (StorageException ex)
{
Console.WriteLine("Error returned from the service: {0}", ex.Message);
}
finally
{
}
}
else
{
Console.WriteLine(
"A connection string has not been defined in the system environment variables. " +
"Add a environment variable named 'storageconnectionstring' with your storage " +
"connection string as a value.");
}
}
}
The second class:
public class TncStorage
{
public static async Task UploadDocToBlobStorage(float version, string filename)
{
CloudStorageAccount storageAccount = null;
CloudBlobContainer cloudBlobContainer = null;
string storageConnectionString = "";
if (CloudStorageAccount.TryParse(storageConnectionString, out storageAccount))
{
try
{
CloudBlobClient cloudBlobClient = storageAccount.CreateCloudBlobClient();
cloudBlobContainer = cloudBlobClient.GetContainerReference("tncdata");
await cloudBlobContainer.CreateIfNotExistsAsync();
BlobContainerPermissions permissions = new BlobContainerPermissions
{
PublicAccess = BlobContainerPublicAccessType.Blob
};
await cloudBlobContainer.SetPermissionsAsync(permissions);
var blobUrl = "";
string file = Guid.NewGuid().ToString() + Path.GetExtension(filename);
CloudBlockBlob cloudBlockBlob = cloudBlobContainer.GetBlockBlobReference(file);
try
{
await cloudBlockBlob.UploadFromFileAsync(filename);
}
catch (Exception ex)
{
}
blobUrl = cloudBlockBlob.Uri.AbsoluteUri;
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
await table.CreateIfNotExistsAsync();
var v = "v" + version;
VersionURL content = new VersionURL("TnC", v);
content.ETag = "*";
content.URL = blobUrl;
var query = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
TableContinuationToken continuationToken = null;
do
{
var result = await table.ExecuteQuerySegmentedAsync(query, continuationToken);
continuationToken = result.ContinuationToken;
if (result.Count() != 0)
{
foreach (VersionURL entity in result)
{
if (entity.RowKey == v)
{
try
{
var dec = version + .1;
content.RowKey = "v" + dec;
TableOperation updateOperation = TableOperation.Insert(content);
await table.ExecuteAsync(updateOperation);
}
catch (Exception ex)
{
}
}
}
}
else
{
TableOperation insertOperation = TableOperation.Insert(content);
await table.ExecuteAsync(insertOperation);
}
} while (continuationToken != null);
}
catch (StorageException ex)
{
Console.WriteLine("Error returned from the service: {0}", ex.Message);
}
finally
{
}
}
else
{
Console.WriteLine(
"A connection string has not been defined in the system environment variables. " +
"Add a environment variable named 'storageconnectionstring' with your storage " +
"connection string as a value.");
}
}
public static string GetURL(float version)
{
CloudStorageAccount storageAccount = null;
string storageConnectionString = "";
storageAccount = CloudStorageAccount.Parse(storageConnectionString);
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
CloudTable table = tableClient.GetTableReference("CommonURL");
var v = "v" + version;
var tableQuery = new TableQuery<VersionURL>();
tableQuery = new TableQuery<VersionURL>().Where(TableQuery.GenerateFilterCondition("RowKey", QueryComparisons.Equal, v));
var entities = table.ExecuteQuerySegmentedAsync(tableQuery, null);
var results = entities.Result;
var s = "";
foreach (var file in results.Where(x => x.RowKey == v))
{
//if (file.RowKey == v)
//{
return s = file.URL;
//}
//else
// return null;
}
return null;
}
How can I simplify the first and the second class?
c# asp.net-web-api azure
c# asp.net-web-api azure
New contributor
T x is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
T x is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 14 mins ago


Jamal♦
30.2k11115226
30.2k11115226
New contributor
T x is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 16 hours ago


T x
11
11
New contributor
T x is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
T x is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
T x is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
5
The request for using dependency-injection is off-topic. We don't implement new features. You also need to better describe your code. The information at the top is very general. Please add more details about what each part is doing and if necessary also write something about how and why.
– t3chb0t
9 hours ago
add a comment |
5
The request for using dependency-injection is off-topic. We don't implement new features. You also need to better describe your code. The information at the top is very general. Please add more details about what each part is doing and if necessary also write something about how and why.
– t3chb0t
9 hours ago
5
5
The request for using dependency-injection is off-topic. We don't implement new features. You also need to better describe your code. The information at the top is very general. Please add more details about what each part is doing and if necessary also write something about how and why.
– t3chb0t
9 hours ago
The request for using dependency-injection is off-topic. We don't implement new features. You also need to better describe your code. The information at the top is very general. Please add more details about what each part is doing and if necessary also write something about how and why.
– t3chb0t
9 hours ago
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
T x is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f209900%2fazure-blob-and-table-storage%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
T x is a new contributor. Be nice, and check out our Code of Conduct.
T x is a new contributor. Be nice, and check out our Code of Conduct.
T x is a new contributor. Be nice, and check out our Code of Conduct.
T x is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f209900%2fazure-blob-and-table-storage%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yDDz vVhj,75BwCgFQYFEOjclBrfSrECHqJ LMekoiDGVT,m,X
5
The request for using dependency-injection is off-topic. We don't implement new features. You also need to better describe your code. The information at the top is very general. Please add more details about what each part is doing and if necessary also write something about how and why.
– t3chb0t
9 hours ago