Award Budget Cuts implementation in Java
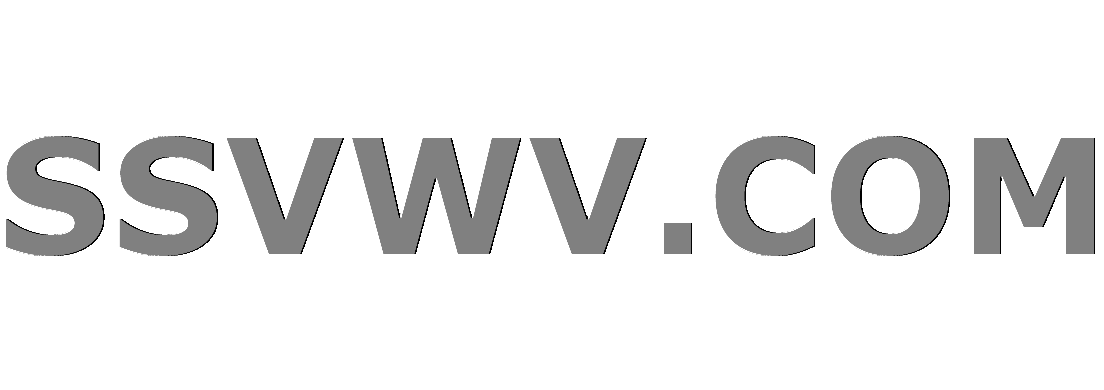
Multi tool use
up vote
1
down vote
favorite
PRAMP Award Budget Cuts
The awards committee of your alma mater (i.e. your college/university) asked for your assistance with a budget allocation problem they’re facing. Originally, the committee planned to give N research grants this year. However, due to spending cutbacks, the budget was reduced to newBudget dollars and now they need to reallocate the grants. The committee made a decision that they’d like to impact as few grant recipients as possible by applying a maximum cap on all grants. Every grant initially planned to be higher than cap will now be exactly cap dollars. Grants less or equal to cap, obviously, won’t be impacted.
Given an array grantsArray of the original grants and the reduced budget newBudget, write a function
findGrantsCap
that finds in the most efficient manner a cap such that the least number of recipients is impacted and that the new budget constraint is met (i.e. sum of the N reallocated grants equals to newBudget).
Example:
input: grantsArray = [2, 100, 50, 120, 1000], newBudget = 190
output: 47 # and given this cap the new grants array would be
# [2, 47, 47, 47, 47]. Notice that the sum of the
# new grants is indeed 190
My approach:
import java.util.Arrays;
class Solution {
static double findGrantsCap(double grantsArray, double newBudget) {
// your code goes here
int len = grantsArray.length;
Arrays.sort(grantsArray);
//Calculate the newBudget
double modBudget = newBudget;
double cap;
double sum = 0;
double avg;
for (double elem: grantsArray)
sum += elem;
avg = sum/len;
if( avg == (int)newBudget)
return (newBudget)/len;
else
{
Arrays.sort(grantsArray);
modBudget -= grantsArray[0];
cap = modBudget/(len - 1);
for( int i = 1; i < len-1; i++ )
{
System.out.println(cap);
if( (int)cap > grantsArray[i] )
{
modBudget -= grantsArray[i];
cap = modBudget/(len - i - 1);
}
}
}
return cap;
}
public static void main(String args) {
double grantsArray = {2,100,50,120,167};
double newBudget = 400;
System.out.println(findGrantsCap(grantsArray,newBudget));
}
}
I have the following questions with regards to the above code:
Is there a better way to attempt this question?
Is my solution satisfying all the test cases and why is it failing if any?
How can I further improve the algorithm, time or space wise?
Have I made any gross violations to the Java Coding conventions?
Reference
java beginner interview-questions complexity
add a comment |
up vote
1
down vote
favorite
PRAMP Award Budget Cuts
The awards committee of your alma mater (i.e. your college/university) asked for your assistance with a budget allocation problem they’re facing. Originally, the committee planned to give N research grants this year. However, due to spending cutbacks, the budget was reduced to newBudget dollars and now they need to reallocate the grants. The committee made a decision that they’d like to impact as few grant recipients as possible by applying a maximum cap on all grants. Every grant initially planned to be higher than cap will now be exactly cap dollars. Grants less or equal to cap, obviously, won’t be impacted.
Given an array grantsArray of the original grants and the reduced budget newBudget, write a function
findGrantsCap
that finds in the most efficient manner a cap such that the least number of recipients is impacted and that the new budget constraint is met (i.e. sum of the N reallocated grants equals to newBudget).
Example:
input: grantsArray = [2, 100, 50, 120, 1000], newBudget = 190
output: 47 # and given this cap the new grants array would be
# [2, 47, 47, 47, 47]. Notice that the sum of the
# new grants is indeed 190
My approach:
import java.util.Arrays;
class Solution {
static double findGrantsCap(double grantsArray, double newBudget) {
// your code goes here
int len = grantsArray.length;
Arrays.sort(grantsArray);
//Calculate the newBudget
double modBudget = newBudget;
double cap;
double sum = 0;
double avg;
for (double elem: grantsArray)
sum += elem;
avg = sum/len;
if( avg == (int)newBudget)
return (newBudget)/len;
else
{
Arrays.sort(grantsArray);
modBudget -= grantsArray[0];
cap = modBudget/(len - 1);
for( int i = 1; i < len-1; i++ )
{
System.out.println(cap);
if( (int)cap > grantsArray[i] )
{
modBudget -= grantsArray[i];
cap = modBudget/(len - i - 1);
}
}
}
return cap;
}
public static void main(String args) {
double grantsArray = {2,100,50,120,167};
double newBudget = 400;
System.out.println(findGrantsCap(grantsArray,newBudget));
}
}
I have the following questions with regards to the above code:
Is there a better way to attempt this question?
Is my solution satisfying all the test cases and why is it failing if any?
How can I further improve the algorithm, time or space wise?
Have I made any gross violations to the Java Coding conventions?
Reference
java beginner interview-questions complexity
"Is my solution satisfying all the test cases and why is it failing if any": have you ran tests yourself?
– Koekje
May 12 at 20:52
@Koekje, Yes, I have run some tests given on the website and my algorithm passes most of them. I am unsure as in how I can solve for the remaining cases. My sincere apologies if this is defying the rules of this web forum.
– Anirudh Thatipelli
May 13 at 4:55
It is written that you should have code which works, to the best of your knowledge. Which you know it does not. So it would seem it does not completely conform. I have no idea how good/bad it is in this case, or what to do :D
– Koekje
May 13 at 23:41
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
PRAMP Award Budget Cuts
The awards committee of your alma mater (i.e. your college/university) asked for your assistance with a budget allocation problem they’re facing. Originally, the committee planned to give N research grants this year. However, due to spending cutbacks, the budget was reduced to newBudget dollars and now they need to reallocate the grants. The committee made a decision that they’d like to impact as few grant recipients as possible by applying a maximum cap on all grants. Every grant initially planned to be higher than cap will now be exactly cap dollars. Grants less or equal to cap, obviously, won’t be impacted.
Given an array grantsArray of the original grants and the reduced budget newBudget, write a function
findGrantsCap
that finds in the most efficient manner a cap such that the least number of recipients is impacted and that the new budget constraint is met (i.e. sum of the N reallocated grants equals to newBudget).
Example:
input: grantsArray = [2, 100, 50, 120, 1000], newBudget = 190
output: 47 # and given this cap the new grants array would be
# [2, 47, 47, 47, 47]. Notice that the sum of the
# new grants is indeed 190
My approach:
import java.util.Arrays;
class Solution {
static double findGrantsCap(double grantsArray, double newBudget) {
// your code goes here
int len = grantsArray.length;
Arrays.sort(grantsArray);
//Calculate the newBudget
double modBudget = newBudget;
double cap;
double sum = 0;
double avg;
for (double elem: grantsArray)
sum += elem;
avg = sum/len;
if( avg == (int)newBudget)
return (newBudget)/len;
else
{
Arrays.sort(grantsArray);
modBudget -= grantsArray[0];
cap = modBudget/(len - 1);
for( int i = 1; i < len-1; i++ )
{
System.out.println(cap);
if( (int)cap > grantsArray[i] )
{
modBudget -= grantsArray[i];
cap = modBudget/(len - i - 1);
}
}
}
return cap;
}
public static void main(String args) {
double grantsArray = {2,100,50,120,167};
double newBudget = 400;
System.out.println(findGrantsCap(grantsArray,newBudget));
}
}
I have the following questions with regards to the above code:
Is there a better way to attempt this question?
Is my solution satisfying all the test cases and why is it failing if any?
How can I further improve the algorithm, time or space wise?
Have I made any gross violations to the Java Coding conventions?
Reference
java beginner interview-questions complexity
PRAMP Award Budget Cuts
The awards committee of your alma mater (i.e. your college/university) asked for your assistance with a budget allocation problem they’re facing. Originally, the committee planned to give N research grants this year. However, due to spending cutbacks, the budget was reduced to newBudget dollars and now they need to reallocate the grants. The committee made a decision that they’d like to impact as few grant recipients as possible by applying a maximum cap on all grants. Every grant initially planned to be higher than cap will now be exactly cap dollars. Grants less or equal to cap, obviously, won’t be impacted.
Given an array grantsArray of the original grants and the reduced budget newBudget, write a function
findGrantsCap
that finds in the most efficient manner a cap such that the least number of recipients is impacted and that the new budget constraint is met (i.e. sum of the N reallocated grants equals to newBudget).
Example:
input: grantsArray = [2, 100, 50, 120, 1000], newBudget = 190
output: 47 # and given this cap the new grants array would be
# [2, 47, 47, 47, 47]. Notice that the sum of the
# new grants is indeed 190
My approach:
import java.util.Arrays;
class Solution {
static double findGrantsCap(double grantsArray, double newBudget) {
// your code goes here
int len = grantsArray.length;
Arrays.sort(grantsArray);
//Calculate the newBudget
double modBudget = newBudget;
double cap;
double sum = 0;
double avg;
for (double elem: grantsArray)
sum += elem;
avg = sum/len;
if( avg == (int)newBudget)
return (newBudget)/len;
else
{
Arrays.sort(grantsArray);
modBudget -= grantsArray[0];
cap = modBudget/(len - 1);
for( int i = 1; i < len-1; i++ )
{
System.out.println(cap);
if( (int)cap > grantsArray[i] )
{
modBudget -= grantsArray[i];
cap = modBudget/(len - i - 1);
}
}
}
return cap;
}
public static void main(String args) {
double grantsArray = {2,100,50,120,167};
double newBudget = 400;
System.out.println(findGrantsCap(grantsArray,newBudget));
}
}
I have the following questions with regards to the above code:
Is there a better way to attempt this question?
Is my solution satisfying all the test cases and why is it failing if any?
How can I further improve the algorithm, time or space wise?
Have I made any gross violations to the Java Coding conventions?
Reference
java beginner interview-questions complexity
java beginner interview-questions complexity
edited Aug 30 at 19:04


Sᴀᴍ Onᴇᴌᴀ
8,12861751
8,12861751
asked May 12 at 19:04


Anirudh Thatipelli
271213
271213
"Is my solution satisfying all the test cases and why is it failing if any": have you ran tests yourself?
– Koekje
May 12 at 20:52
@Koekje, Yes, I have run some tests given on the website and my algorithm passes most of them. I am unsure as in how I can solve for the remaining cases. My sincere apologies if this is defying the rules of this web forum.
– Anirudh Thatipelli
May 13 at 4:55
It is written that you should have code which works, to the best of your knowledge. Which you know it does not. So it would seem it does not completely conform. I have no idea how good/bad it is in this case, or what to do :D
– Koekje
May 13 at 23:41
add a comment |
"Is my solution satisfying all the test cases and why is it failing if any": have you ran tests yourself?
– Koekje
May 12 at 20:52
@Koekje, Yes, I have run some tests given on the website and my algorithm passes most of them. I am unsure as in how I can solve for the remaining cases. My sincere apologies if this is defying the rules of this web forum.
– Anirudh Thatipelli
May 13 at 4:55
It is written that you should have code which works, to the best of your knowledge. Which you know it does not. So it would seem it does not completely conform. I have no idea how good/bad it is in this case, or what to do :D
– Koekje
May 13 at 23:41
"Is my solution satisfying all the test cases and why is it failing if any": have you ran tests yourself?
– Koekje
May 12 at 20:52
"Is my solution satisfying all the test cases and why is it failing if any": have you ran tests yourself?
– Koekje
May 12 at 20:52
@Koekje, Yes, I have run some tests given on the website and my algorithm passes most of them. I am unsure as in how I can solve for the remaining cases. My sincere apologies if this is defying the rules of this web forum.
– Anirudh Thatipelli
May 13 at 4:55
@Koekje, Yes, I have run some tests given on the website and my algorithm passes most of them. I am unsure as in how I can solve for the remaining cases. My sincere apologies if this is defying the rules of this web forum.
– Anirudh Thatipelli
May 13 at 4:55
It is written that you should have code which works, to the best of your knowledge. Which you know it does not. So it would seem it does not completely conform. I have no idea how good/bad it is in this case, or what to do :D
– Koekje
May 13 at 23:41
It is written that you should have code which works, to the best of your knowledge. Which you know it does not. So it would seem it does not completely conform. I have no idea how good/bad it is in this case, or what to do :D
– Koekje
May 13 at 23:41
add a comment |
4 Answers
4
active
oldest
votes
up vote
2
down vote
I believe that the problem in the first place is not well defined (or we are trying to solve a different problem). And I mean...
The problem states the following:
write a function findGrantsCap that finds in the most efficient manner a cap such that the least number of recipients is impacted and that the new budget constraint is met
So it focuses on the recipients impacted and not on the amounts.
e.g
Input: grantsArray = [2, 100, 50, 120, 1000], newBudget = 190
If I pick for cap the 100 value the array becomes like this
Input: grantsArray = [2, 100, 50, 1, 37], newBudget = 190
Now only 2 recipients affected (of course the sum of the array elements meets the newBudget constraint), but it's totally unfair.
1
"If I pich" --- was that supposed to be "If I pitch"?
– Sᴀᴍ Onᴇᴌᴀ
Aug 30 at 18:56
Just "pick" :)
– E. Bekas
Aug 31 at 8:29
I also found this question confusing, but your solution doesn't work because of this clause: "Every grant initially planned to be higher than cap will now be exactly cap dollars."
– mcfroob
Nov 20 at 12:00
add a comment |
up vote
1
down vote
1) Code from https://repl.it/@sixxta/Award-Budget-Cuts suggests you could sort array in descending order and keep decreasing maximum single grant until there is no overspending. I don't know if you version, starting with smallest value, works correctly.
2) for cases:
{14,15,16,17,18,19}, 97
{14,15,16,17,18,19}, 98
{14,15,16,17,18,19}, 99
your code returns:
17.33
17.66
18.5
While the proper answer is:
17
18
19
3) the algorithm is optimal in terms of time and space, unless the is some way to remove sorting that I am not aware of.
4) you are looking for exact solution, using floating point variables isn't a good idea
if( avg == (int)newBudget)
return (newBudget)/len;
This check doesn't make any sense. You probably wanted to compare with newBudget/len
but even then you would return 2
for {1,2,3},6
when right answer is 3
.
Thanks for the advice. @user158037. I will keep this important floating point issue in mind.
– Anirudh Thatipelli
May 19 at 16:06
add a comment |
up vote
1
down vote
There is a better approach for that problem.
First start by sorting the array, next traverse the array, for every element that is lower than the cap
(your cap
starts as the avg
of budget/array.length
) reduce it from your current funds and recalculate the cap (i.e. now the cap
equals to budget/(array.length - (i+1))
).
Once you got to the end of the array or to the first element that is higher than your current cap
you just return the last cap you have in hand.
So all in all this is your solution:
static double findGrantsCap(double arr, double newBudget) {
// your code goes here
Arrays.sort(arr); // O(N*LogN)
int n = arr.length;
double cap = newBudget/n;
for(int i = 0; i < n; i++) { // O(N)
if(arr[i] < cap) {
newBudget -= arr[i];
cap = (newBudget/(n-(1+i)));
}else {
arr[i] = cap;
}
}
return cap;
}
Adding all the numbers on the array will be the newBudget since for every lower number we subtract it from the total and everything above just gets capped (essentially gets to be the average from what we got left with divided with the total elements we got left with - adding those will be the remains of the budget we got up to them).
add a comment |
up vote
0
down vote
This is my solution with complexity: time $O(N*log(N))$, space $O(1)$.
static double findGrantsCap(double grantsArray, double newBudget) {
if (grantsArray == null ||
grantsArray.length == 0 ||
(grantsArray.length == 1 && grantsArray[0] <= newBudget)) {
return newBudget;
}
Arrays.sort(grantsArray);
double cap = newBudget / grantsArray.length;
double allocated = 0.0;
for (int i=0; i<grantsArray.length; i++) {
double currentRequestValue = grantsArray[i];
if (currentRequestValue <= cap) {
allocated += currentRequestValue;
int divisor = (grantsArray.length - i - 1);
// If last index in the array is below the cap divisor will be "0"
// It means that all the items in the carousel can be allocated as they are
cap = divisor != 0 ? (newBudget - allocated) / divisor : currentRequestValue;
}
}
return cap;
}
New contributor
user72708 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f194272%2faward-budget-cuts-implementation-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
I believe that the problem in the first place is not well defined (or we are trying to solve a different problem). And I mean...
The problem states the following:
write a function findGrantsCap that finds in the most efficient manner a cap such that the least number of recipients is impacted and that the new budget constraint is met
So it focuses on the recipients impacted and not on the amounts.
e.g
Input: grantsArray = [2, 100, 50, 120, 1000], newBudget = 190
If I pick for cap the 100 value the array becomes like this
Input: grantsArray = [2, 100, 50, 1, 37], newBudget = 190
Now only 2 recipients affected (of course the sum of the array elements meets the newBudget constraint), but it's totally unfair.
1
"If I pich" --- was that supposed to be "If I pitch"?
– Sᴀᴍ Onᴇᴌᴀ
Aug 30 at 18:56
Just "pick" :)
– E. Bekas
Aug 31 at 8:29
I also found this question confusing, but your solution doesn't work because of this clause: "Every grant initially planned to be higher than cap will now be exactly cap dollars."
– mcfroob
Nov 20 at 12:00
add a comment |
up vote
2
down vote
I believe that the problem in the first place is not well defined (or we are trying to solve a different problem). And I mean...
The problem states the following:
write a function findGrantsCap that finds in the most efficient manner a cap such that the least number of recipients is impacted and that the new budget constraint is met
So it focuses on the recipients impacted and not on the amounts.
e.g
Input: grantsArray = [2, 100, 50, 120, 1000], newBudget = 190
If I pick for cap the 100 value the array becomes like this
Input: grantsArray = [2, 100, 50, 1, 37], newBudget = 190
Now only 2 recipients affected (of course the sum of the array elements meets the newBudget constraint), but it's totally unfair.
1
"If I pich" --- was that supposed to be "If I pitch"?
– Sᴀᴍ Onᴇᴌᴀ
Aug 30 at 18:56
Just "pick" :)
– E. Bekas
Aug 31 at 8:29
I also found this question confusing, but your solution doesn't work because of this clause: "Every grant initially planned to be higher than cap will now be exactly cap dollars."
– mcfroob
Nov 20 at 12:00
add a comment |
up vote
2
down vote
up vote
2
down vote
I believe that the problem in the first place is not well defined (or we are trying to solve a different problem). And I mean...
The problem states the following:
write a function findGrantsCap that finds in the most efficient manner a cap such that the least number of recipients is impacted and that the new budget constraint is met
So it focuses on the recipients impacted and not on the amounts.
e.g
Input: grantsArray = [2, 100, 50, 120, 1000], newBudget = 190
If I pick for cap the 100 value the array becomes like this
Input: grantsArray = [2, 100, 50, 1, 37], newBudget = 190
Now only 2 recipients affected (of course the sum of the array elements meets the newBudget constraint), but it's totally unfair.
I believe that the problem in the first place is not well defined (or we are trying to solve a different problem). And I mean...
The problem states the following:
write a function findGrantsCap that finds in the most efficient manner a cap such that the least number of recipients is impacted and that the new budget constraint is met
So it focuses on the recipients impacted and not on the amounts.
e.g
Input: grantsArray = [2, 100, 50, 120, 1000], newBudget = 190
If I pick for cap the 100 value the array becomes like this
Input: grantsArray = [2, 100, 50, 1, 37], newBudget = 190
Now only 2 recipients affected (of course the sum of the array elements meets the newBudget constraint), but it's totally unfair.
edited Aug 31 at 8:28
answered Aug 30 at 17:55
E. Bekas
213
213
1
"If I pich" --- was that supposed to be "If I pitch"?
– Sᴀᴍ Onᴇᴌᴀ
Aug 30 at 18:56
Just "pick" :)
– E. Bekas
Aug 31 at 8:29
I also found this question confusing, but your solution doesn't work because of this clause: "Every grant initially planned to be higher than cap will now be exactly cap dollars."
– mcfroob
Nov 20 at 12:00
add a comment |
1
"If I pich" --- was that supposed to be "If I pitch"?
– Sᴀᴍ Onᴇᴌᴀ
Aug 30 at 18:56
Just "pick" :)
– E. Bekas
Aug 31 at 8:29
I also found this question confusing, but your solution doesn't work because of this clause: "Every grant initially planned to be higher than cap will now be exactly cap dollars."
– mcfroob
Nov 20 at 12:00
1
1
"If I pich" --- was that supposed to be "If I pitch"?
– Sᴀᴍ Onᴇᴌᴀ
Aug 30 at 18:56
"If I pich" --- was that supposed to be "If I pitch"?
– Sᴀᴍ Onᴇᴌᴀ
Aug 30 at 18:56
Just "pick" :)
– E. Bekas
Aug 31 at 8:29
Just "pick" :)
– E. Bekas
Aug 31 at 8:29
I also found this question confusing, but your solution doesn't work because of this clause: "Every grant initially planned to be higher than cap will now be exactly cap dollars."
– mcfroob
Nov 20 at 12:00
I also found this question confusing, but your solution doesn't work because of this clause: "Every grant initially planned to be higher than cap will now be exactly cap dollars."
– mcfroob
Nov 20 at 12:00
add a comment |
up vote
1
down vote
1) Code from https://repl.it/@sixxta/Award-Budget-Cuts suggests you could sort array in descending order and keep decreasing maximum single grant until there is no overspending. I don't know if you version, starting with smallest value, works correctly.
2) for cases:
{14,15,16,17,18,19}, 97
{14,15,16,17,18,19}, 98
{14,15,16,17,18,19}, 99
your code returns:
17.33
17.66
18.5
While the proper answer is:
17
18
19
3) the algorithm is optimal in terms of time and space, unless the is some way to remove sorting that I am not aware of.
4) you are looking for exact solution, using floating point variables isn't a good idea
if( avg == (int)newBudget)
return (newBudget)/len;
This check doesn't make any sense. You probably wanted to compare with newBudget/len
but even then you would return 2
for {1,2,3},6
when right answer is 3
.
Thanks for the advice. @user158037. I will keep this important floating point issue in mind.
– Anirudh Thatipelli
May 19 at 16:06
add a comment |
up vote
1
down vote
1) Code from https://repl.it/@sixxta/Award-Budget-Cuts suggests you could sort array in descending order and keep decreasing maximum single grant until there is no overspending. I don't know if you version, starting with smallest value, works correctly.
2) for cases:
{14,15,16,17,18,19}, 97
{14,15,16,17,18,19}, 98
{14,15,16,17,18,19}, 99
your code returns:
17.33
17.66
18.5
While the proper answer is:
17
18
19
3) the algorithm is optimal in terms of time and space, unless the is some way to remove sorting that I am not aware of.
4) you are looking for exact solution, using floating point variables isn't a good idea
if( avg == (int)newBudget)
return (newBudget)/len;
This check doesn't make any sense. You probably wanted to compare with newBudget/len
but even then you would return 2
for {1,2,3},6
when right answer is 3
.
Thanks for the advice. @user158037. I will keep this important floating point issue in mind.
– Anirudh Thatipelli
May 19 at 16:06
add a comment |
up vote
1
down vote
up vote
1
down vote
1) Code from https://repl.it/@sixxta/Award-Budget-Cuts suggests you could sort array in descending order and keep decreasing maximum single grant until there is no overspending. I don't know if you version, starting with smallest value, works correctly.
2) for cases:
{14,15,16,17,18,19}, 97
{14,15,16,17,18,19}, 98
{14,15,16,17,18,19}, 99
your code returns:
17.33
17.66
18.5
While the proper answer is:
17
18
19
3) the algorithm is optimal in terms of time and space, unless the is some way to remove sorting that I am not aware of.
4) you are looking for exact solution, using floating point variables isn't a good idea
if( avg == (int)newBudget)
return (newBudget)/len;
This check doesn't make any sense. You probably wanted to compare with newBudget/len
but even then you would return 2
for {1,2,3},6
when right answer is 3
.
1) Code from https://repl.it/@sixxta/Award-Budget-Cuts suggests you could sort array in descending order and keep decreasing maximum single grant until there is no overspending. I don't know if you version, starting with smallest value, works correctly.
2) for cases:
{14,15,16,17,18,19}, 97
{14,15,16,17,18,19}, 98
{14,15,16,17,18,19}, 99
your code returns:
17.33
17.66
18.5
While the proper answer is:
17
18
19
3) the algorithm is optimal in terms of time and space, unless the is some way to remove sorting that I am not aware of.
4) you are looking for exact solution, using floating point variables isn't a good idea
if( avg == (int)newBudget)
return (newBudget)/len;
This check doesn't make any sense. You probably wanted to compare with newBudget/len
but even then you would return 2
for {1,2,3},6
when right answer is 3
.
answered May 16 at 10:41
user158037
54637
54637
Thanks for the advice. @user158037. I will keep this important floating point issue in mind.
– Anirudh Thatipelli
May 19 at 16:06
add a comment |
Thanks for the advice. @user158037. I will keep this important floating point issue in mind.
– Anirudh Thatipelli
May 19 at 16:06
Thanks for the advice. @user158037. I will keep this important floating point issue in mind.
– Anirudh Thatipelli
May 19 at 16:06
Thanks for the advice. @user158037. I will keep this important floating point issue in mind.
– Anirudh Thatipelli
May 19 at 16:06
add a comment |
up vote
1
down vote
There is a better approach for that problem.
First start by sorting the array, next traverse the array, for every element that is lower than the cap
(your cap
starts as the avg
of budget/array.length
) reduce it from your current funds and recalculate the cap (i.e. now the cap
equals to budget/(array.length - (i+1))
).
Once you got to the end of the array or to the first element that is higher than your current cap
you just return the last cap you have in hand.
So all in all this is your solution:
static double findGrantsCap(double arr, double newBudget) {
// your code goes here
Arrays.sort(arr); // O(N*LogN)
int n = arr.length;
double cap = newBudget/n;
for(int i = 0; i < n; i++) { // O(N)
if(arr[i] < cap) {
newBudget -= arr[i];
cap = (newBudget/(n-(1+i)));
}else {
arr[i] = cap;
}
}
return cap;
}
Adding all the numbers on the array will be the newBudget since for every lower number we subtract it from the total and everything above just gets capped (essentially gets to be the average from what we got left with divided with the total elements we got left with - adding those will be the remains of the budget we got up to them).
add a comment |
up vote
1
down vote
There is a better approach for that problem.
First start by sorting the array, next traverse the array, for every element that is lower than the cap
(your cap
starts as the avg
of budget/array.length
) reduce it from your current funds and recalculate the cap (i.e. now the cap
equals to budget/(array.length - (i+1))
).
Once you got to the end of the array or to the first element that is higher than your current cap
you just return the last cap you have in hand.
So all in all this is your solution:
static double findGrantsCap(double arr, double newBudget) {
// your code goes here
Arrays.sort(arr); // O(N*LogN)
int n = arr.length;
double cap = newBudget/n;
for(int i = 0; i < n; i++) { // O(N)
if(arr[i] < cap) {
newBudget -= arr[i];
cap = (newBudget/(n-(1+i)));
}else {
arr[i] = cap;
}
}
return cap;
}
Adding all the numbers on the array will be the newBudget since for every lower number we subtract it from the total and everything above just gets capped (essentially gets to be the average from what we got left with divided with the total elements we got left with - adding those will be the remains of the budget we got up to them).
add a comment |
up vote
1
down vote
up vote
1
down vote
There is a better approach for that problem.
First start by sorting the array, next traverse the array, for every element that is lower than the cap
(your cap
starts as the avg
of budget/array.length
) reduce it from your current funds and recalculate the cap (i.e. now the cap
equals to budget/(array.length - (i+1))
).
Once you got to the end of the array or to the first element that is higher than your current cap
you just return the last cap you have in hand.
So all in all this is your solution:
static double findGrantsCap(double arr, double newBudget) {
// your code goes here
Arrays.sort(arr); // O(N*LogN)
int n = arr.length;
double cap = newBudget/n;
for(int i = 0; i < n; i++) { // O(N)
if(arr[i] < cap) {
newBudget -= arr[i];
cap = (newBudget/(n-(1+i)));
}else {
arr[i] = cap;
}
}
return cap;
}
Adding all the numbers on the array will be the newBudget since for every lower number we subtract it from the total and everything above just gets capped (essentially gets to be the average from what we got left with divided with the total elements we got left with - adding those will be the remains of the budget we got up to them).
There is a better approach for that problem.
First start by sorting the array, next traverse the array, for every element that is lower than the cap
(your cap
starts as the avg
of budget/array.length
) reduce it from your current funds and recalculate the cap (i.e. now the cap
equals to budget/(array.length - (i+1))
).
Once you got to the end of the array or to the first element that is higher than your current cap
you just return the last cap you have in hand.
So all in all this is your solution:
static double findGrantsCap(double arr, double newBudget) {
// your code goes here
Arrays.sort(arr); // O(N*LogN)
int n = arr.length;
double cap = newBudget/n;
for(int i = 0; i < n; i++) { // O(N)
if(arr[i] < cap) {
newBudget -= arr[i];
cap = (newBudget/(n-(1+i)));
}else {
arr[i] = cap;
}
}
return cap;
}
Adding all the numbers on the array will be the newBudget since for every lower number we subtract it from the total and everything above just gets capped (essentially gets to be the average from what we got left with divided with the total elements we got left with - adding those will be the remains of the budget we got up to them).
edited Jul 23 at 17:25


t3chb0t
33.8k746110
33.8k746110
answered Jul 23 at 17:16


crazyPixel
1286
1286
add a comment |
add a comment |
up vote
0
down vote
This is my solution with complexity: time $O(N*log(N))$, space $O(1)$.
static double findGrantsCap(double grantsArray, double newBudget) {
if (grantsArray == null ||
grantsArray.length == 0 ||
(grantsArray.length == 1 && grantsArray[0] <= newBudget)) {
return newBudget;
}
Arrays.sort(grantsArray);
double cap = newBudget / grantsArray.length;
double allocated = 0.0;
for (int i=0; i<grantsArray.length; i++) {
double currentRequestValue = grantsArray[i];
if (currentRequestValue <= cap) {
allocated += currentRequestValue;
int divisor = (grantsArray.length - i - 1);
// If last index in the array is below the cap divisor will be "0"
// It means that all the items in the carousel can be allocated as they are
cap = divisor != 0 ? (newBudget - allocated) / divisor : currentRequestValue;
}
}
return cap;
}
New contributor
user72708 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
This is my solution with complexity: time $O(N*log(N))$, space $O(1)$.
static double findGrantsCap(double grantsArray, double newBudget) {
if (grantsArray == null ||
grantsArray.length == 0 ||
(grantsArray.length == 1 && grantsArray[0] <= newBudget)) {
return newBudget;
}
Arrays.sort(grantsArray);
double cap = newBudget / grantsArray.length;
double allocated = 0.0;
for (int i=0; i<grantsArray.length; i++) {
double currentRequestValue = grantsArray[i];
if (currentRequestValue <= cap) {
allocated += currentRequestValue;
int divisor = (grantsArray.length - i - 1);
// If last index in the array is below the cap divisor will be "0"
// It means that all the items in the carousel can be allocated as they are
cap = divisor != 0 ? (newBudget - allocated) / divisor : currentRequestValue;
}
}
return cap;
}
New contributor
user72708 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
up vote
0
down vote
This is my solution with complexity: time $O(N*log(N))$, space $O(1)$.
static double findGrantsCap(double grantsArray, double newBudget) {
if (grantsArray == null ||
grantsArray.length == 0 ||
(grantsArray.length == 1 && grantsArray[0] <= newBudget)) {
return newBudget;
}
Arrays.sort(grantsArray);
double cap = newBudget / grantsArray.length;
double allocated = 0.0;
for (int i=0; i<grantsArray.length; i++) {
double currentRequestValue = grantsArray[i];
if (currentRequestValue <= cap) {
allocated += currentRequestValue;
int divisor = (grantsArray.length - i - 1);
// If last index in the array is below the cap divisor will be "0"
// It means that all the items in the carousel can be allocated as they are
cap = divisor != 0 ? (newBudget - allocated) / divisor : currentRequestValue;
}
}
return cap;
}
New contributor
user72708 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
This is my solution with complexity: time $O(N*log(N))$, space $O(1)$.
static double findGrantsCap(double grantsArray, double newBudget) {
if (grantsArray == null ||
grantsArray.length == 0 ||
(grantsArray.length == 1 && grantsArray[0] <= newBudget)) {
return newBudget;
}
Arrays.sort(grantsArray);
double cap = newBudget / grantsArray.length;
double allocated = 0.0;
for (int i=0; i<grantsArray.length; i++) {
double currentRequestValue = grantsArray[i];
if (currentRequestValue <= cap) {
allocated += currentRequestValue;
int divisor = (grantsArray.length - i - 1);
// If last index in the array is below the cap divisor will be "0"
// It means that all the items in the carousel can be allocated as they are
cap = divisor != 0 ? (newBudget - allocated) / divisor : currentRequestValue;
}
}
return cap;
}
New contributor
user72708 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 14 hours ago


alecxe
14.5k53277
14.5k53277
New contributor
user72708 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 15 hours ago
user72708
1011
1011
New contributor
user72708 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
user72708 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
user72708 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f194272%2faward-budget-cuts-implementation-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sz3zlhv5MLDk M4kG66GLCEMQ5SfUlu8XxrYQkZB28sSIjJM9,z9MWbF1k vd
"Is my solution satisfying all the test cases and why is it failing if any": have you ran tests yourself?
– Koekje
May 12 at 20:52
@Koekje, Yes, I have run some tests given on the website and my algorithm passes most of them. I am unsure as in how I can solve for the remaining cases. My sincere apologies if this is defying the rules of this web forum.
– Anirudh Thatipelli
May 13 at 4:55
It is written that you should have code which works, to the best of your knowledge. Which you know it does not. So it would seem it does not completely conform. I have no idea how good/bad it is in this case, or what to do :D
– Koekje
May 13 at 23:41