Genetic algorithm fitness function for scheduling
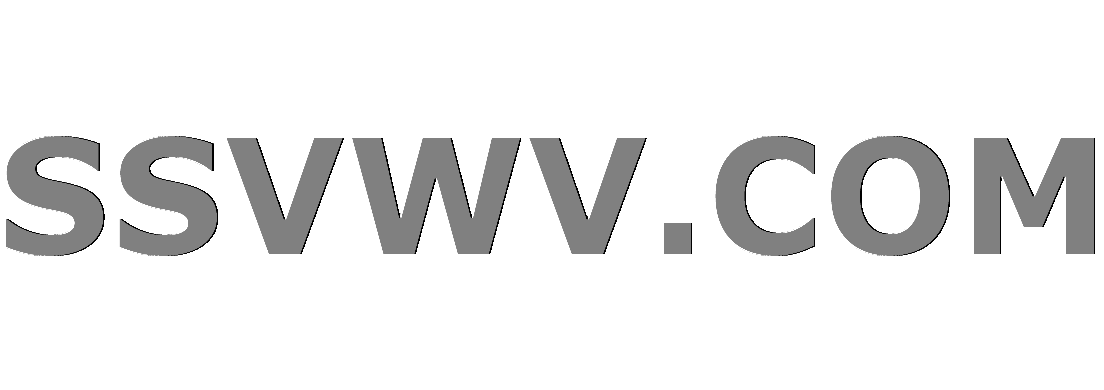
Multi tool use
up vote
2
down vote
favorite
For Job shop scheduling, I built a fitness function:
def makespan_timeCalculation(lot_size, RHS, setup_time, processing_time, sequence, mc_op):
machine_assigne_time =[[0 for i in range(mc_op[k])] for k in range(len(mc_op))]
max_c = [0 for i in range(len(lot_size))] # Equal number of job
completion_time = [0 for i in range(len(RHS))]
# Check all indices of RHS (Step 2 + 3 + 6)
for r in range(len(RHS)):
procTime = run_time(r, lot_size, RHS, sequence, processing_time) + setup_time[RHS[r][2]]
if lot_size[RHS[r][0]][RHS[r][1]] > 0: # Lot size not zero (Step 4)
if (machine_assigne_time[RHS[r][2]][RHS[r][3]] == 0 and RHS[r][2] == sequence[RHS[r][0]][0]):
# Step 5.1: First assignment to machine m and operation equal first operation in sequence
completion_time[r] = setup_time[RHS[r][2]] + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] == 0 and RHS[r][2] != sequence[RHS[r][0]][0]):
# Step 5.2: First assignment to machine m and operation over first operation in sequence
curent_sequence_index = sequence[RHS[r][0]].index(RHS[r][2]) # Calculate index sequence of current chromosome
prev_sequence = sequence[RHS[r][0]][curent_sequence_index-1] # Return previous sequence operation of current chromosome
prev_operation_comp_index = [i[0:3] for i in RHS].index([RHS[r][0], RHS[r][1], prev_sequence]) # Return previous chromosome of current job + sublot
completion_time[r] = max(setup_time[RHS[r][2]], completion_time[prev_operation_comp_index]) + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
#print(curent_sequence_index, RHS[r])
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] > 0 and RHS[r][2] == sequence[RHS[r][0]][0]):
# Step 5.3: First operation in sequence and machine m assignment > 1 (dung de xem xet khi co su khac nhau giua setup time ban dau, va setup time doi part)
prev_op_mc_RHS_index = max([i for i, al in enumerate(RHS[:r]) if RHS[r][2:] == al[2:]])
completion_time[r] = completion_time[prev_op_mc_RHS_index] + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] > 0 and RHS[r][2] != sequence[RHS[r][0]][0]):
# Step 5.4 Operation over first operation in sequence and machine m assignment > 1
curent_sequence_index = sequence[RHS[r][0]].index(RHS[r][2]) # Calculate index sequence of current chromosome
prev_sequence = sequence[RHS[r][0]][curent_sequence_index-1] # Return previous sequence operation of current chromosome
prev_operation_comp_index = [i[0:3] for i in RHS].index([RHS[r][0], RHS[r][1], prev_sequence]) # Return previous chromosome of current job + sublot
prev_op_mc_RHS_index = max([i for i, al in enumerate(RHS[:r]) if RHS[r][2:] == al[2:]])
completion_time[r] = max(completion_time[prev_operation_comp_index], completion_time[prev_op_mc_RHS_index]) + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
max_c[RHS[r][0]] = max(max_c[RHS[r][0]], completion_time[r])
return max(max_c)
The result is fine but speed is very slow. Could you help to have a look and advise on improvement?
python performance genetic-algorithm
bumped to the homepage by Community♦ 2 days ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
up vote
2
down vote
favorite
For Job shop scheduling, I built a fitness function:
def makespan_timeCalculation(lot_size, RHS, setup_time, processing_time, sequence, mc_op):
machine_assigne_time =[[0 for i in range(mc_op[k])] for k in range(len(mc_op))]
max_c = [0 for i in range(len(lot_size))] # Equal number of job
completion_time = [0 for i in range(len(RHS))]
# Check all indices of RHS (Step 2 + 3 + 6)
for r in range(len(RHS)):
procTime = run_time(r, lot_size, RHS, sequence, processing_time) + setup_time[RHS[r][2]]
if lot_size[RHS[r][0]][RHS[r][1]] > 0: # Lot size not zero (Step 4)
if (machine_assigne_time[RHS[r][2]][RHS[r][3]] == 0 and RHS[r][2] == sequence[RHS[r][0]][0]):
# Step 5.1: First assignment to machine m and operation equal first operation in sequence
completion_time[r] = setup_time[RHS[r][2]] + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] == 0 and RHS[r][2] != sequence[RHS[r][0]][0]):
# Step 5.2: First assignment to machine m and operation over first operation in sequence
curent_sequence_index = sequence[RHS[r][0]].index(RHS[r][2]) # Calculate index sequence of current chromosome
prev_sequence = sequence[RHS[r][0]][curent_sequence_index-1] # Return previous sequence operation of current chromosome
prev_operation_comp_index = [i[0:3] for i in RHS].index([RHS[r][0], RHS[r][1], prev_sequence]) # Return previous chromosome of current job + sublot
completion_time[r] = max(setup_time[RHS[r][2]], completion_time[prev_operation_comp_index]) + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
#print(curent_sequence_index, RHS[r])
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] > 0 and RHS[r][2] == sequence[RHS[r][0]][0]):
# Step 5.3: First operation in sequence and machine m assignment > 1 (dung de xem xet khi co su khac nhau giua setup time ban dau, va setup time doi part)
prev_op_mc_RHS_index = max([i for i, al in enumerate(RHS[:r]) if RHS[r][2:] == al[2:]])
completion_time[r] = completion_time[prev_op_mc_RHS_index] + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] > 0 and RHS[r][2] != sequence[RHS[r][0]][0]):
# Step 5.4 Operation over first operation in sequence and machine m assignment > 1
curent_sequence_index = sequence[RHS[r][0]].index(RHS[r][2]) # Calculate index sequence of current chromosome
prev_sequence = sequence[RHS[r][0]][curent_sequence_index-1] # Return previous sequence operation of current chromosome
prev_operation_comp_index = [i[0:3] for i in RHS].index([RHS[r][0], RHS[r][1], prev_sequence]) # Return previous chromosome of current job + sublot
prev_op_mc_RHS_index = max([i for i, al in enumerate(RHS[:r]) if RHS[r][2:] == al[2:]])
completion_time[r] = max(completion_time[prev_operation_comp_index], completion_time[prev_op_mc_RHS_index]) + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
max_c[RHS[r][0]] = max(max_c[RHS[r][0]], completion_time[r])
return max(max_c)
The result is fine but speed is very slow. Could you help to have a look and advise on improvement?
python performance genetic-algorithm
bumped to the homepage by Community♦ 2 days ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
Welcome to Code Review! The current question title, which states your concerns about the code, is too general to be useful here. Please edit to the site standard, which is for the title to simply state the task accomplished by the code. Please see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles. Also, you don't need to mention the language - that information is in the tags.
– Toby Speight
Aug 22 at 9:20
1
Could you add some example input? Specifically, it is unclear what exactlyRHS
,lot_size
, etc look like (are they nested dictionaries/lists?).
– Graipher
Aug 22 at 9:40
Please don't use paste.ofcode.org for code. The snippets expire after a week, which means the link would be useless. Add the code directly into the question
– Zoe
Sep 22 at 9:06
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
For Job shop scheduling, I built a fitness function:
def makespan_timeCalculation(lot_size, RHS, setup_time, processing_time, sequence, mc_op):
machine_assigne_time =[[0 for i in range(mc_op[k])] for k in range(len(mc_op))]
max_c = [0 for i in range(len(lot_size))] # Equal number of job
completion_time = [0 for i in range(len(RHS))]
# Check all indices of RHS (Step 2 + 3 + 6)
for r in range(len(RHS)):
procTime = run_time(r, lot_size, RHS, sequence, processing_time) + setup_time[RHS[r][2]]
if lot_size[RHS[r][0]][RHS[r][1]] > 0: # Lot size not zero (Step 4)
if (machine_assigne_time[RHS[r][2]][RHS[r][3]] == 0 and RHS[r][2] == sequence[RHS[r][0]][0]):
# Step 5.1: First assignment to machine m and operation equal first operation in sequence
completion_time[r] = setup_time[RHS[r][2]] + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] == 0 and RHS[r][2] != sequence[RHS[r][0]][0]):
# Step 5.2: First assignment to machine m and operation over first operation in sequence
curent_sequence_index = sequence[RHS[r][0]].index(RHS[r][2]) # Calculate index sequence of current chromosome
prev_sequence = sequence[RHS[r][0]][curent_sequence_index-1] # Return previous sequence operation of current chromosome
prev_operation_comp_index = [i[0:3] for i in RHS].index([RHS[r][0], RHS[r][1], prev_sequence]) # Return previous chromosome of current job + sublot
completion_time[r] = max(setup_time[RHS[r][2]], completion_time[prev_operation_comp_index]) + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
#print(curent_sequence_index, RHS[r])
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] > 0 and RHS[r][2] == sequence[RHS[r][0]][0]):
# Step 5.3: First operation in sequence and machine m assignment > 1 (dung de xem xet khi co su khac nhau giua setup time ban dau, va setup time doi part)
prev_op_mc_RHS_index = max([i for i, al in enumerate(RHS[:r]) if RHS[r][2:] == al[2:]])
completion_time[r] = completion_time[prev_op_mc_RHS_index] + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] > 0 and RHS[r][2] != sequence[RHS[r][0]][0]):
# Step 5.4 Operation over first operation in sequence and machine m assignment > 1
curent_sequence_index = sequence[RHS[r][0]].index(RHS[r][2]) # Calculate index sequence of current chromosome
prev_sequence = sequence[RHS[r][0]][curent_sequence_index-1] # Return previous sequence operation of current chromosome
prev_operation_comp_index = [i[0:3] for i in RHS].index([RHS[r][0], RHS[r][1], prev_sequence]) # Return previous chromosome of current job + sublot
prev_op_mc_RHS_index = max([i for i, al in enumerate(RHS[:r]) if RHS[r][2:] == al[2:]])
completion_time[r] = max(completion_time[prev_operation_comp_index], completion_time[prev_op_mc_RHS_index]) + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
max_c[RHS[r][0]] = max(max_c[RHS[r][0]], completion_time[r])
return max(max_c)
The result is fine but speed is very slow. Could you help to have a look and advise on improvement?
python performance genetic-algorithm
For Job shop scheduling, I built a fitness function:
def makespan_timeCalculation(lot_size, RHS, setup_time, processing_time, sequence, mc_op):
machine_assigne_time =[[0 for i in range(mc_op[k])] for k in range(len(mc_op))]
max_c = [0 for i in range(len(lot_size))] # Equal number of job
completion_time = [0 for i in range(len(RHS))]
# Check all indices of RHS (Step 2 + 3 + 6)
for r in range(len(RHS)):
procTime = run_time(r, lot_size, RHS, sequence, processing_time) + setup_time[RHS[r][2]]
if lot_size[RHS[r][0]][RHS[r][1]] > 0: # Lot size not zero (Step 4)
if (machine_assigne_time[RHS[r][2]][RHS[r][3]] == 0 and RHS[r][2] == sequence[RHS[r][0]][0]):
# Step 5.1: First assignment to machine m and operation equal first operation in sequence
completion_time[r] = setup_time[RHS[r][2]] + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] == 0 and RHS[r][2] != sequence[RHS[r][0]][0]):
# Step 5.2: First assignment to machine m and operation over first operation in sequence
curent_sequence_index = sequence[RHS[r][0]].index(RHS[r][2]) # Calculate index sequence of current chromosome
prev_sequence = sequence[RHS[r][0]][curent_sequence_index-1] # Return previous sequence operation of current chromosome
prev_operation_comp_index = [i[0:3] for i in RHS].index([RHS[r][0], RHS[r][1], prev_sequence]) # Return previous chromosome of current job + sublot
completion_time[r] = max(setup_time[RHS[r][2]], completion_time[prev_operation_comp_index]) + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
#print(curent_sequence_index, RHS[r])
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] > 0 and RHS[r][2] == sequence[RHS[r][0]][0]):
# Step 5.3: First operation in sequence and machine m assignment > 1 (dung de xem xet khi co su khac nhau giua setup time ban dau, va setup time doi part)
prev_op_mc_RHS_index = max([i for i, al in enumerate(RHS[:r]) if RHS[r][2:] == al[2:]])
completion_time[r] = completion_time[prev_op_mc_RHS_index] + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
elif (machine_assigne_time[RHS[r][2]][RHS[r][3]] > 0 and RHS[r][2] != sequence[RHS[r][0]][0]):
# Step 5.4 Operation over first operation in sequence and machine m assignment > 1
curent_sequence_index = sequence[RHS[r][0]].index(RHS[r][2]) # Calculate index sequence of current chromosome
prev_sequence = sequence[RHS[r][0]][curent_sequence_index-1] # Return previous sequence operation of current chromosome
prev_operation_comp_index = [i[0:3] for i in RHS].index([RHS[r][0], RHS[r][1], prev_sequence]) # Return previous chromosome of current job + sublot
prev_op_mc_RHS_index = max([i for i, al in enumerate(RHS[:r]) if RHS[r][2:] == al[2:]])
completion_time[r] = max(completion_time[prev_operation_comp_index], completion_time[prev_op_mc_RHS_index]) + procTime
machine_assigne_time[RHS[r][2]][RHS[r][3]] +=1
max_c[RHS[r][0]] = max(max_c[RHS[r][0]], completion_time[r])
return max(max_c)
The result is fine but speed is very slow. Could you help to have a look and advise on improvement?
python performance genetic-algorithm
python performance genetic-algorithm
edited Sep 22 at 13:20


Zoe
21719
21719
asked Aug 22 at 7:15


Khái Duy
113
113
bumped to the homepage by Community♦ 2 days ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
bumped to the homepage by Community♦ 2 days ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
Welcome to Code Review! The current question title, which states your concerns about the code, is too general to be useful here. Please edit to the site standard, which is for the title to simply state the task accomplished by the code. Please see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles. Also, you don't need to mention the language - that information is in the tags.
– Toby Speight
Aug 22 at 9:20
1
Could you add some example input? Specifically, it is unclear what exactlyRHS
,lot_size
, etc look like (are they nested dictionaries/lists?).
– Graipher
Aug 22 at 9:40
Please don't use paste.ofcode.org for code. The snippets expire after a week, which means the link would be useless. Add the code directly into the question
– Zoe
Sep 22 at 9:06
add a comment |
Welcome to Code Review! The current question title, which states your concerns about the code, is too general to be useful here. Please edit to the site standard, which is for the title to simply state the task accomplished by the code. Please see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles. Also, you don't need to mention the language - that information is in the tags.
– Toby Speight
Aug 22 at 9:20
1
Could you add some example input? Specifically, it is unclear what exactlyRHS
,lot_size
, etc look like (are they nested dictionaries/lists?).
– Graipher
Aug 22 at 9:40
Please don't use paste.ofcode.org for code. The snippets expire after a week, which means the link would be useless. Add the code directly into the question
– Zoe
Sep 22 at 9:06
Welcome to Code Review! The current question title, which states your concerns about the code, is too general to be useful here. Please edit to the site standard, which is for the title to simply state the task accomplished by the code. Please see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles. Also, you don't need to mention the language - that information is in the tags.
– Toby Speight
Aug 22 at 9:20
Welcome to Code Review! The current question title, which states your concerns about the code, is too general to be useful here. Please edit to the site standard, which is for the title to simply state the task accomplished by the code. Please see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles. Also, you don't need to mention the language - that information is in the tags.
– Toby Speight
Aug 22 at 9:20
1
1
Could you add some example input? Specifically, it is unclear what exactly
RHS
, lot_size
, etc look like (are they nested dictionaries/lists?).– Graipher
Aug 22 at 9:40
Could you add some example input? Specifically, it is unclear what exactly
RHS
, lot_size
, etc look like (are they nested dictionaries/lists?).– Graipher
Aug 22 at 9:40
Please don't use paste.ofcode.org for code. The snippets expire after a week, which means the link would be useless. Add the code directly into the question
– Zoe
Sep 22 at 9:06
Please don't use paste.ofcode.org for code. The snippets expire after a week, which means the link would be useless. Add the code directly into the question
– Zoe
Sep 22 at 9:06
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
I think the biggest boost to readability will come from giving things proper names. You currently have a lot of RHS[r][i]
going on, with i = 0, 1, 2, 3
. For someone who does not know what these things are (including possibly you in a few months), this makes the code basically unreadable.
I would start by making the iteration look like this:
for r, (a, b, c, d) in enumerate(RHS):
...
Where a, b, c, d
are actual sensible names (like name
, length
, or whatever is actually at those positions).
Next, your setup code can be simplified using the fact that e.g. [0] * 3 == [0, 0, 0]
(but don't do it with nested loops, see below):
machine_assigne_time = [[0] * op] for op in mc_op]
max_c = [0] * len(lot_size) # Equal number of job
completion_time = [0] * len(RHS)
And finally, without being able to run your code, the most obvious case for the slow down are your repeated calls to list.index
. This is very slow ($mathcal{O}(n)$ in the worst case). I would try to find a more efficient data structure for this (dictionaries?).
Hi Graipher, kindly check my data structure per parameter as edited above. Could you advise the way how to re-structure it?
– Khái Duy
Aug 23 at 2:39
Kindly check link I have problem when running many times in row 142, 144. Can you help to advise also?
– Khái Duy
Aug 23 at 2:52
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
I think the biggest boost to readability will come from giving things proper names. You currently have a lot of RHS[r][i]
going on, with i = 0, 1, 2, 3
. For someone who does not know what these things are (including possibly you in a few months), this makes the code basically unreadable.
I would start by making the iteration look like this:
for r, (a, b, c, d) in enumerate(RHS):
...
Where a, b, c, d
are actual sensible names (like name
, length
, or whatever is actually at those positions).
Next, your setup code can be simplified using the fact that e.g. [0] * 3 == [0, 0, 0]
(but don't do it with nested loops, see below):
machine_assigne_time = [[0] * op] for op in mc_op]
max_c = [0] * len(lot_size) # Equal number of job
completion_time = [0] * len(RHS)
And finally, without being able to run your code, the most obvious case for the slow down are your repeated calls to list.index
. This is very slow ($mathcal{O}(n)$ in the worst case). I would try to find a more efficient data structure for this (dictionaries?).
Hi Graipher, kindly check my data structure per parameter as edited above. Could you advise the way how to re-structure it?
– Khái Duy
Aug 23 at 2:39
Kindly check link I have problem when running many times in row 142, 144. Can you help to advise also?
– Khái Duy
Aug 23 at 2:52
add a comment |
up vote
1
down vote
I think the biggest boost to readability will come from giving things proper names. You currently have a lot of RHS[r][i]
going on, with i = 0, 1, 2, 3
. For someone who does not know what these things are (including possibly you in a few months), this makes the code basically unreadable.
I would start by making the iteration look like this:
for r, (a, b, c, d) in enumerate(RHS):
...
Where a, b, c, d
are actual sensible names (like name
, length
, or whatever is actually at those positions).
Next, your setup code can be simplified using the fact that e.g. [0] * 3 == [0, 0, 0]
(but don't do it with nested loops, see below):
machine_assigne_time = [[0] * op] for op in mc_op]
max_c = [0] * len(lot_size) # Equal number of job
completion_time = [0] * len(RHS)
And finally, without being able to run your code, the most obvious case for the slow down are your repeated calls to list.index
. This is very slow ($mathcal{O}(n)$ in the worst case). I would try to find a more efficient data structure for this (dictionaries?).
Hi Graipher, kindly check my data structure per parameter as edited above. Could you advise the way how to re-structure it?
– Khái Duy
Aug 23 at 2:39
Kindly check link I have problem when running many times in row 142, 144. Can you help to advise also?
– Khái Duy
Aug 23 at 2:52
add a comment |
up vote
1
down vote
up vote
1
down vote
I think the biggest boost to readability will come from giving things proper names. You currently have a lot of RHS[r][i]
going on, with i = 0, 1, 2, 3
. For someone who does not know what these things are (including possibly you in a few months), this makes the code basically unreadable.
I would start by making the iteration look like this:
for r, (a, b, c, d) in enumerate(RHS):
...
Where a, b, c, d
are actual sensible names (like name
, length
, or whatever is actually at those positions).
Next, your setup code can be simplified using the fact that e.g. [0] * 3 == [0, 0, 0]
(but don't do it with nested loops, see below):
machine_assigne_time = [[0] * op] for op in mc_op]
max_c = [0] * len(lot_size) # Equal number of job
completion_time = [0] * len(RHS)
And finally, without being able to run your code, the most obvious case for the slow down are your repeated calls to list.index
. This is very slow ($mathcal{O}(n)$ in the worst case). I would try to find a more efficient data structure for this (dictionaries?).
I think the biggest boost to readability will come from giving things proper names. You currently have a lot of RHS[r][i]
going on, with i = 0, 1, 2, 3
. For someone who does not know what these things are (including possibly you in a few months), this makes the code basically unreadable.
I would start by making the iteration look like this:
for r, (a, b, c, d) in enumerate(RHS):
...
Where a, b, c, d
are actual sensible names (like name
, length
, or whatever is actually at those positions).
Next, your setup code can be simplified using the fact that e.g. [0] * 3 == [0, 0, 0]
(but don't do it with nested loops, see below):
machine_assigne_time = [[0] * op] for op in mc_op]
max_c = [0] * len(lot_size) # Equal number of job
completion_time = [0] * len(RHS)
And finally, without being able to run your code, the most obvious case for the slow down are your repeated calls to list.index
. This is very slow ($mathcal{O}(n)$ in the worst case). I would try to find a more efficient data structure for this (dictionaries?).
answered Aug 22 at 9:48
Graipher
22.2k53284
22.2k53284
Hi Graipher, kindly check my data structure per parameter as edited above. Could you advise the way how to re-structure it?
– Khái Duy
Aug 23 at 2:39
Kindly check link I have problem when running many times in row 142, 144. Can you help to advise also?
– Khái Duy
Aug 23 at 2:52
add a comment |
Hi Graipher, kindly check my data structure per parameter as edited above. Could you advise the way how to re-structure it?
– Khái Duy
Aug 23 at 2:39
Kindly check link I have problem when running many times in row 142, 144. Can you help to advise also?
– Khái Duy
Aug 23 at 2:52
Hi Graipher, kindly check my data structure per parameter as edited above. Could you advise the way how to re-structure it?
– Khái Duy
Aug 23 at 2:39
Hi Graipher, kindly check my data structure per parameter as edited above. Could you advise the way how to re-structure it?
– Khái Duy
Aug 23 at 2:39
Kindly check link I have problem when running many times in row 142, 144. Can you help to advise also?
– Khái Duy
Aug 23 at 2:52
Kindly check link I have problem when running many times in row 142, 144. Can you help to advise also?
– Khái Duy
Aug 23 at 2:52
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f202190%2fgenetic-algorithm-fitness-function-for-scheduling%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HiJu8FfHm0R3ks SJBdK2DOK6Nrt2,HBU,8KQH866o ZI4,0T9jME8FoB4s1ySrk55xBl6
Welcome to Code Review! The current question title, which states your concerns about the code, is too general to be useful here. Please edit to the site standard, which is for the title to simply state the task accomplished by the code. Please see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles. Also, you don't need to mention the language - that information is in the tags.
– Toby Speight
Aug 22 at 9:20
1
Could you add some example input? Specifically, it is unclear what exactly
RHS
,lot_size
, etc look like (are they nested dictionaries/lists?).– Graipher
Aug 22 at 9:40
Please don't use paste.ofcode.org for code. The snippets expire after a week, which means the link would be useless. Add the code directly into the question
– Zoe
Sep 22 at 9:06