Recursive method that chooses a single String according to multiple “color category” parameters
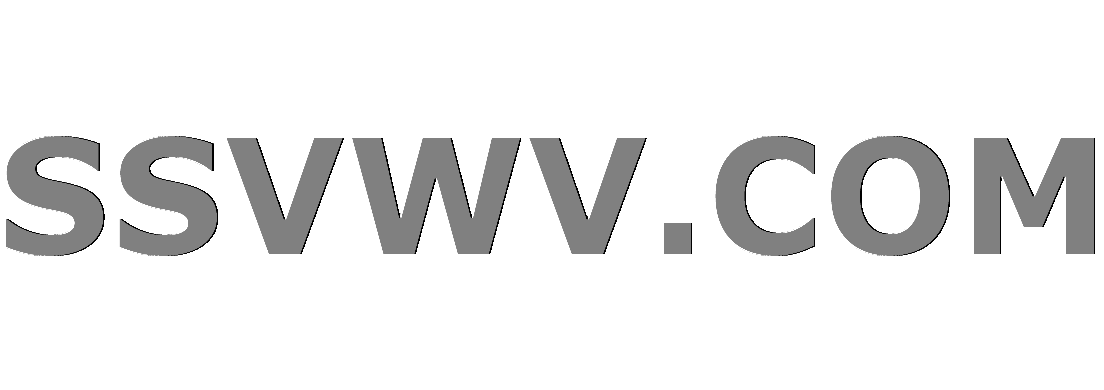
Multi tool use
I've created a recursive method to return a single, randomly-selected, String-value, that represents a specific color. It chooses this color from one, or several, "color categories", such as "blue", or "yellow".
Currently calling the method requires at least three arguments.
I feel like this method has too many parameters, and I would like to reduce them.
I know I could do this iteratively, which I am fine with, but that seems like it would mean I would have get rid of the switch-case and do a bunch of "else-if" statements, which I'd like to avoid.
Optimally, I'd like to be able to do the same thing with just the String... colorCategory
parameters.
public class MCVE {
private static String pupilColorPoss = {
"yellow", "chartreuse", "emerald", "cyan", "blue", "indigo",
"purple", "violet", "magenta", "scarlet", "red", "vermilion",
"orange", "golden", "brown", "chestnut", "auburn", "white",
"black"};
public static void main(String args) {
HashSet<String> colors = new HashSet<>();
String printValue = randomizePupilColor(colors, 2, "blue", "yellow", "green");
System.out.println(printValue);
}
private static String randomizePupilColor(HashSet<String> colors, int index,
String... colorCategory) {
// add all the colors from a certain category to the HashSet colors
switch (colorCategory[index]) {
case "yellow":
colors.add(pupilColorPoss[0]); // yellow
colors.add(pupilColorPoss[1]); // chartreuse
colors.add(pupilColorPoss[12]); // golden
colors.add(pupilColorPoss[13]); // orange
break;
case "green":
colors.add(pupilColorPoss[1]); // chartreuse
colors.add(pupilColorPoss[2]); // emerald
colors.add(pupilColorPoss[3]); // cyan
break;
case "blue":
colors.add(pupilColorPoss[3]); // cyan
colors.add(pupilColorPoss[4]); // blue
colors.add(pupilColorPoss[5]); // indigo
break;
case "purple":
colors.add(pupilColorPoss[5]); // indigo
colors.add(pupilColorPoss[6]); // purple
colors.add(pupilColorPoss[7]); // violet
colors.add(pupilColorPoss[8]); // magenta
break;
case "red":
colors.add(pupilColorPoss[8]); // magenta
colors.add(pupilColorPoss[9]); // scarlet
colors.add(pupilColorPoss[10]); // red
colors.add(pupilColorPoss[11]); // vermilion
colors.add(pupilColorPoss[16]); // auburn
break;
case "orange":
colors.add(pupilColorPoss[11]); // vermilion
colors.add(pupilColorPoss[12]); // orange
colors.add(pupilColorPoss[13]); // golden
break;
case "brown":
colors.add(pupilColorPoss[14]); // brown
colors.add(pupilColorPoss[15]); // chestnut
colors.add(pupilColorPoss[16]); // auburn
break;
case "black":
colors.add(pupilColorPoss[18]); // black
break;
case "white":
colors.add(pupilColorPoss[17]); // white
break;
default:
throw new IllegalArgumentException(
colorCategory[index] + " is not valid");
}
// recursion stop-case
if(index == 0) {
int stop = ThreadLocalRandom.current().nextInt(0, colors.size());
Iterator<String> iter = colors.iterator();
for(int i = 0; i < stop; i++)
iter.next();
return iter.next();
}
return randomizePupilColor(colors, index - 1, colorCategory);
}
}
java array recursion iteration static
add a comment |
I've created a recursive method to return a single, randomly-selected, String-value, that represents a specific color. It chooses this color from one, or several, "color categories", such as "blue", or "yellow".
Currently calling the method requires at least three arguments.
I feel like this method has too many parameters, and I would like to reduce them.
I know I could do this iteratively, which I am fine with, but that seems like it would mean I would have get rid of the switch-case and do a bunch of "else-if" statements, which I'd like to avoid.
Optimally, I'd like to be able to do the same thing with just the String... colorCategory
parameters.
public class MCVE {
private static String pupilColorPoss = {
"yellow", "chartreuse", "emerald", "cyan", "blue", "indigo",
"purple", "violet", "magenta", "scarlet", "red", "vermilion",
"orange", "golden", "brown", "chestnut", "auburn", "white",
"black"};
public static void main(String args) {
HashSet<String> colors = new HashSet<>();
String printValue = randomizePupilColor(colors, 2, "blue", "yellow", "green");
System.out.println(printValue);
}
private static String randomizePupilColor(HashSet<String> colors, int index,
String... colorCategory) {
// add all the colors from a certain category to the HashSet colors
switch (colorCategory[index]) {
case "yellow":
colors.add(pupilColorPoss[0]); // yellow
colors.add(pupilColorPoss[1]); // chartreuse
colors.add(pupilColorPoss[12]); // golden
colors.add(pupilColorPoss[13]); // orange
break;
case "green":
colors.add(pupilColorPoss[1]); // chartreuse
colors.add(pupilColorPoss[2]); // emerald
colors.add(pupilColorPoss[3]); // cyan
break;
case "blue":
colors.add(pupilColorPoss[3]); // cyan
colors.add(pupilColorPoss[4]); // blue
colors.add(pupilColorPoss[5]); // indigo
break;
case "purple":
colors.add(pupilColorPoss[5]); // indigo
colors.add(pupilColorPoss[6]); // purple
colors.add(pupilColorPoss[7]); // violet
colors.add(pupilColorPoss[8]); // magenta
break;
case "red":
colors.add(pupilColorPoss[8]); // magenta
colors.add(pupilColorPoss[9]); // scarlet
colors.add(pupilColorPoss[10]); // red
colors.add(pupilColorPoss[11]); // vermilion
colors.add(pupilColorPoss[16]); // auburn
break;
case "orange":
colors.add(pupilColorPoss[11]); // vermilion
colors.add(pupilColorPoss[12]); // orange
colors.add(pupilColorPoss[13]); // golden
break;
case "brown":
colors.add(pupilColorPoss[14]); // brown
colors.add(pupilColorPoss[15]); // chestnut
colors.add(pupilColorPoss[16]); // auburn
break;
case "black":
colors.add(pupilColorPoss[18]); // black
break;
case "white":
colors.add(pupilColorPoss[17]); // white
break;
default:
throw new IllegalArgumentException(
colorCategory[index] + " is not valid");
}
// recursion stop-case
if(index == 0) {
int stop = ThreadLocalRandom.current().nextInt(0, colors.size());
Iterator<String> iter = colors.iterator();
for(int i = 0; i < stop; i++)
iter.next();
return iter.next();
}
return randomizePupilColor(colors, index - 1, colorCategory);
}
}
java array recursion iteration static
add a comment |
I've created a recursive method to return a single, randomly-selected, String-value, that represents a specific color. It chooses this color from one, or several, "color categories", such as "blue", or "yellow".
Currently calling the method requires at least three arguments.
I feel like this method has too many parameters, and I would like to reduce them.
I know I could do this iteratively, which I am fine with, but that seems like it would mean I would have get rid of the switch-case and do a bunch of "else-if" statements, which I'd like to avoid.
Optimally, I'd like to be able to do the same thing with just the String... colorCategory
parameters.
public class MCVE {
private static String pupilColorPoss = {
"yellow", "chartreuse", "emerald", "cyan", "blue", "indigo",
"purple", "violet", "magenta", "scarlet", "red", "vermilion",
"orange", "golden", "brown", "chestnut", "auburn", "white",
"black"};
public static void main(String args) {
HashSet<String> colors = new HashSet<>();
String printValue = randomizePupilColor(colors, 2, "blue", "yellow", "green");
System.out.println(printValue);
}
private static String randomizePupilColor(HashSet<String> colors, int index,
String... colorCategory) {
// add all the colors from a certain category to the HashSet colors
switch (colorCategory[index]) {
case "yellow":
colors.add(pupilColorPoss[0]); // yellow
colors.add(pupilColorPoss[1]); // chartreuse
colors.add(pupilColorPoss[12]); // golden
colors.add(pupilColorPoss[13]); // orange
break;
case "green":
colors.add(pupilColorPoss[1]); // chartreuse
colors.add(pupilColorPoss[2]); // emerald
colors.add(pupilColorPoss[3]); // cyan
break;
case "blue":
colors.add(pupilColorPoss[3]); // cyan
colors.add(pupilColorPoss[4]); // blue
colors.add(pupilColorPoss[5]); // indigo
break;
case "purple":
colors.add(pupilColorPoss[5]); // indigo
colors.add(pupilColorPoss[6]); // purple
colors.add(pupilColorPoss[7]); // violet
colors.add(pupilColorPoss[8]); // magenta
break;
case "red":
colors.add(pupilColorPoss[8]); // magenta
colors.add(pupilColorPoss[9]); // scarlet
colors.add(pupilColorPoss[10]); // red
colors.add(pupilColorPoss[11]); // vermilion
colors.add(pupilColorPoss[16]); // auburn
break;
case "orange":
colors.add(pupilColorPoss[11]); // vermilion
colors.add(pupilColorPoss[12]); // orange
colors.add(pupilColorPoss[13]); // golden
break;
case "brown":
colors.add(pupilColorPoss[14]); // brown
colors.add(pupilColorPoss[15]); // chestnut
colors.add(pupilColorPoss[16]); // auburn
break;
case "black":
colors.add(pupilColorPoss[18]); // black
break;
case "white":
colors.add(pupilColorPoss[17]); // white
break;
default:
throw new IllegalArgumentException(
colorCategory[index] + " is not valid");
}
// recursion stop-case
if(index == 0) {
int stop = ThreadLocalRandom.current().nextInt(0, colors.size());
Iterator<String> iter = colors.iterator();
for(int i = 0; i < stop; i++)
iter.next();
return iter.next();
}
return randomizePupilColor(colors, index - 1, colorCategory);
}
}
java array recursion iteration static
I've created a recursive method to return a single, randomly-selected, String-value, that represents a specific color. It chooses this color from one, or several, "color categories", such as "blue", or "yellow".
Currently calling the method requires at least three arguments.
I feel like this method has too many parameters, and I would like to reduce them.
I know I could do this iteratively, which I am fine with, but that seems like it would mean I would have get rid of the switch-case and do a bunch of "else-if" statements, which I'd like to avoid.
Optimally, I'd like to be able to do the same thing with just the String... colorCategory
parameters.
public class MCVE {
private static String pupilColorPoss = {
"yellow", "chartreuse", "emerald", "cyan", "blue", "indigo",
"purple", "violet", "magenta", "scarlet", "red", "vermilion",
"orange", "golden", "brown", "chestnut", "auburn", "white",
"black"};
public static void main(String args) {
HashSet<String> colors = new HashSet<>();
String printValue = randomizePupilColor(colors, 2, "blue", "yellow", "green");
System.out.println(printValue);
}
private static String randomizePupilColor(HashSet<String> colors, int index,
String... colorCategory) {
// add all the colors from a certain category to the HashSet colors
switch (colorCategory[index]) {
case "yellow":
colors.add(pupilColorPoss[0]); // yellow
colors.add(pupilColorPoss[1]); // chartreuse
colors.add(pupilColorPoss[12]); // golden
colors.add(pupilColorPoss[13]); // orange
break;
case "green":
colors.add(pupilColorPoss[1]); // chartreuse
colors.add(pupilColorPoss[2]); // emerald
colors.add(pupilColorPoss[3]); // cyan
break;
case "blue":
colors.add(pupilColorPoss[3]); // cyan
colors.add(pupilColorPoss[4]); // blue
colors.add(pupilColorPoss[5]); // indigo
break;
case "purple":
colors.add(pupilColorPoss[5]); // indigo
colors.add(pupilColorPoss[6]); // purple
colors.add(pupilColorPoss[7]); // violet
colors.add(pupilColorPoss[8]); // magenta
break;
case "red":
colors.add(pupilColorPoss[8]); // magenta
colors.add(pupilColorPoss[9]); // scarlet
colors.add(pupilColorPoss[10]); // red
colors.add(pupilColorPoss[11]); // vermilion
colors.add(pupilColorPoss[16]); // auburn
break;
case "orange":
colors.add(pupilColorPoss[11]); // vermilion
colors.add(pupilColorPoss[12]); // orange
colors.add(pupilColorPoss[13]); // golden
break;
case "brown":
colors.add(pupilColorPoss[14]); // brown
colors.add(pupilColorPoss[15]); // chestnut
colors.add(pupilColorPoss[16]); // auburn
break;
case "black":
colors.add(pupilColorPoss[18]); // black
break;
case "white":
colors.add(pupilColorPoss[17]); // white
break;
default:
throw new IllegalArgumentException(
colorCategory[index] + " is not valid");
}
// recursion stop-case
if(index == 0) {
int stop = ThreadLocalRandom.current().nextInt(0, colors.size());
Iterator<String> iter = colors.iterator();
for(int i = 0; i < stop; i++)
iter.next();
return iter.next();
}
return randomizePupilColor(colors, index - 1, colorCategory);
}
}
java array recursion iteration static
java array recursion iteration static
asked 8 mins ago


LuminousNutria
1265
1265
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f210852%2frecursive-method-that-chooses-a-single-string-according-to-multiple-color-categ%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f210852%2frecursive-method-that-chooses-a-single-string-according-to-multiple-color-categ%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
n6knIEn,nrsybFfYml8R,0YhgDBe