Helper class for Fragments management
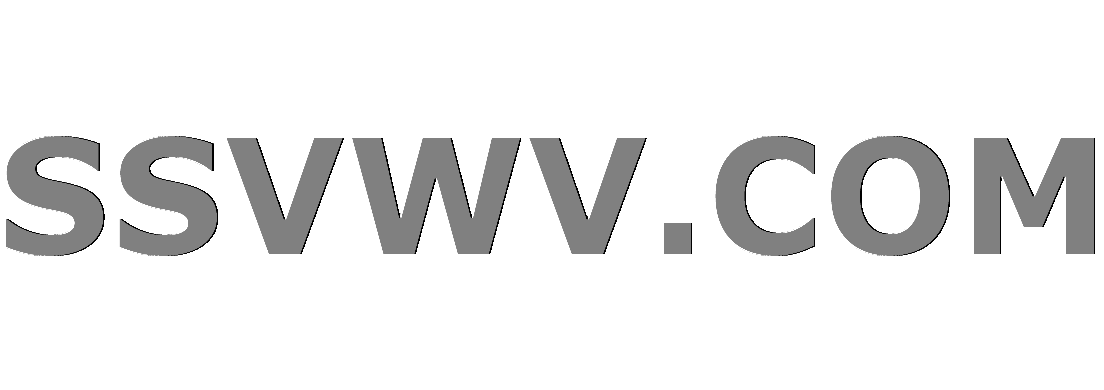
Multi tool use
up vote
2
down vote
favorite
A Helper class for Fragment Management.
The FragmentsManager class has methods to add and replace fragments.
The replace method checks if the given fragment is present in backstack or not and if the fragment is present it brings back the old fragment.
Need review for the following:
- Code optimized.
- Memory Efficiency
- Overall code review
- Can the replace method be improved in any way so as to avoid creation of additional fragments?
- I've tried to use popbackstack() but couldn't how to use it. Can you help with that?
Thanks
import android.app.Activity;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentTransaction;
import android.support.v7.app.AppCompatActivity;
class FragmentsManager {
private static final String TAG = "FragmentsManager";
private static FragmentTransaction transaction;
// Return Fragment Transaction
private static FragmentTransaction getTransaction(Activity activity){
// if (transaction == null) {
// return transaction;
// }
return getFragmentManager(activity).beginTransaction();
}
// Return Fragment Manager
private static FragmentManager getFragmentManager(Activity activity){
return ((AppCompatActivity)activity).getSupportFragmentManager();
}
/**
* Add Fragment to the given ID
* @param activity
* @param fragment
* @param id
* @param add_to_backstack
*/
static void addFragment(Activity activity, Fragment fragment, int id, boolean add_to_backstack){
transaction = getTransaction(activity);
transaction.add(id,fragment,fragment.getClass().getName());
if (add_to_backstack)
transaction.addToBackStack(fragment.getClass().getName());
transaction.commit();
}
static void replaceFragment(Activity activity, Fragment fragment, int id, boolean add_to_backstack) {
Fragment check_Fragment = getFragmentManager(activity).findFragmentByTag(fragment.getClass().getName());
if (check_Fragment == null) {
transaction = getTransaction(activity)
.replace(id,fragment,fragment.getClass().getName());
if (add_to_backstack)
transaction.addToBackStack(fragment.getClass().getName());
transaction.commit();
}
else{
transaction = getTransaction(activity);
transaction.replace(id,check_Fragment,check_Fragment.getClass().getName())
.addToBackStack(null)
.commit();
}
}
}
java android
New contributor
sdb1995 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
2
down vote
favorite
A Helper class for Fragment Management.
The FragmentsManager class has methods to add and replace fragments.
The replace method checks if the given fragment is present in backstack or not and if the fragment is present it brings back the old fragment.
Need review for the following:
- Code optimized.
- Memory Efficiency
- Overall code review
- Can the replace method be improved in any way so as to avoid creation of additional fragments?
- I've tried to use popbackstack() but couldn't how to use it. Can you help with that?
Thanks
import android.app.Activity;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentTransaction;
import android.support.v7.app.AppCompatActivity;
class FragmentsManager {
private static final String TAG = "FragmentsManager";
private static FragmentTransaction transaction;
// Return Fragment Transaction
private static FragmentTransaction getTransaction(Activity activity){
// if (transaction == null) {
// return transaction;
// }
return getFragmentManager(activity).beginTransaction();
}
// Return Fragment Manager
private static FragmentManager getFragmentManager(Activity activity){
return ((AppCompatActivity)activity).getSupportFragmentManager();
}
/**
* Add Fragment to the given ID
* @param activity
* @param fragment
* @param id
* @param add_to_backstack
*/
static void addFragment(Activity activity, Fragment fragment, int id, boolean add_to_backstack){
transaction = getTransaction(activity);
transaction.add(id,fragment,fragment.getClass().getName());
if (add_to_backstack)
transaction.addToBackStack(fragment.getClass().getName());
transaction.commit();
}
static void replaceFragment(Activity activity, Fragment fragment, int id, boolean add_to_backstack) {
Fragment check_Fragment = getFragmentManager(activity).findFragmentByTag(fragment.getClass().getName());
if (check_Fragment == null) {
transaction = getTransaction(activity)
.replace(id,fragment,fragment.getClass().getName());
if (add_to_backstack)
transaction.addToBackStack(fragment.getClass().getName());
transaction.commit();
}
else{
transaction = getTransaction(activity);
transaction.replace(id,check_Fragment,check_Fragment.getClass().getName())
.addToBackStack(null)
.commit();
}
}
}
java android
New contributor
sdb1995 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
A Helper class for Fragment Management.
The FragmentsManager class has methods to add and replace fragments.
The replace method checks if the given fragment is present in backstack or not and if the fragment is present it brings back the old fragment.
Need review for the following:
- Code optimized.
- Memory Efficiency
- Overall code review
- Can the replace method be improved in any way so as to avoid creation of additional fragments?
- I've tried to use popbackstack() but couldn't how to use it. Can you help with that?
Thanks
import android.app.Activity;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentTransaction;
import android.support.v7.app.AppCompatActivity;
class FragmentsManager {
private static final String TAG = "FragmentsManager";
private static FragmentTransaction transaction;
// Return Fragment Transaction
private static FragmentTransaction getTransaction(Activity activity){
// if (transaction == null) {
// return transaction;
// }
return getFragmentManager(activity).beginTransaction();
}
// Return Fragment Manager
private static FragmentManager getFragmentManager(Activity activity){
return ((AppCompatActivity)activity).getSupportFragmentManager();
}
/**
* Add Fragment to the given ID
* @param activity
* @param fragment
* @param id
* @param add_to_backstack
*/
static void addFragment(Activity activity, Fragment fragment, int id, boolean add_to_backstack){
transaction = getTransaction(activity);
transaction.add(id,fragment,fragment.getClass().getName());
if (add_to_backstack)
transaction.addToBackStack(fragment.getClass().getName());
transaction.commit();
}
static void replaceFragment(Activity activity, Fragment fragment, int id, boolean add_to_backstack) {
Fragment check_Fragment = getFragmentManager(activity).findFragmentByTag(fragment.getClass().getName());
if (check_Fragment == null) {
transaction = getTransaction(activity)
.replace(id,fragment,fragment.getClass().getName());
if (add_to_backstack)
transaction.addToBackStack(fragment.getClass().getName());
transaction.commit();
}
else{
transaction = getTransaction(activity);
transaction.replace(id,check_Fragment,check_Fragment.getClass().getName())
.addToBackStack(null)
.commit();
}
}
}
java android
New contributor
sdb1995 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
A Helper class for Fragment Management.
The FragmentsManager class has methods to add and replace fragments.
The replace method checks if the given fragment is present in backstack or not and if the fragment is present it brings back the old fragment.
Need review for the following:
- Code optimized.
- Memory Efficiency
- Overall code review
- Can the replace method be improved in any way so as to avoid creation of additional fragments?
- I've tried to use popbackstack() but couldn't how to use it. Can you help with that?
Thanks
import android.app.Activity;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentTransaction;
import android.support.v7.app.AppCompatActivity;
class FragmentsManager {
private static final String TAG = "FragmentsManager";
private static FragmentTransaction transaction;
// Return Fragment Transaction
private static FragmentTransaction getTransaction(Activity activity){
// if (transaction == null) {
// return transaction;
// }
return getFragmentManager(activity).beginTransaction();
}
// Return Fragment Manager
private static FragmentManager getFragmentManager(Activity activity){
return ((AppCompatActivity)activity).getSupportFragmentManager();
}
/**
* Add Fragment to the given ID
* @param activity
* @param fragment
* @param id
* @param add_to_backstack
*/
static void addFragment(Activity activity, Fragment fragment, int id, boolean add_to_backstack){
transaction = getTransaction(activity);
transaction.add(id,fragment,fragment.getClass().getName());
if (add_to_backstack)
transaction.addToBackStack(fragment.getClass().getName());
transaction.commit();
}
static void replaceFragment(Activity activity, Fragment fragment, int id, boolean add_to_backstack) {
Fragment check_Fragment = getFragmentManager(activity).findFragmentByTag(fragment.getClass().getName());
if (check_Fragment == null) {
transaction = getTransaction(activity)
.replace(id,fragment,fragment.getClass().getName());
if (add_to_backstack)
transaction.addToBackStack(fragment.getClass().getName());
transaction.commit();
}
else{
transaction = getTransaction(activity);
transaction.replace(id,check_Fragment,check_Fragment.getClass().getName())
.addToBackStack(null)
.commit();
}
}
}
java android
java android
New contributor
sdb1995 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
sdb1995 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 2 days ago


200_success
127k15148411
127k15148411
New contributor
sdb1995 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 2 days ago


sdb1995
112
112
New contributor
sdb1995 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
sdb1995 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
sdb1995 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
your code looks very good, there are only minor issues:
1) naming
since you don't create any instances of FragmentsManager
you should consider to rename it to FragmentsUtility
. An Utility class provides methods to help you with your code while a Manager is an instance that does the work for you.
boolean should beginn with prefix is (or rarely as an alternative has/can/should) (in Java it's convention to use camelCase)
so rename your boolean add_to_backstack
into boolean isAddedToBackstack
rather (btw. in java it's convention to use camelCase)
2) comments
remove commented code!
3) javadoc
you provide some javadoc on the addFragment
methode which is very crude - get things done and finish it!
the same applies to replaceFragment
.
Thank you for your review. I thought JavaDocs should be provided for all the methods defined for description purposes. So I added them. Guess I was wrong. Thanks again.
– sdb1995
21 hours ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
your code looks very good, there are only minor issues:
1) naming
since you don't create any instances of FragmentsManager
you should consider to rename it to FragmentsUtility
. An Utility class provides methods to help you with your code while a Manager is an instance that does the work for you.
boolean should beginn with prefix is (or rarely as an alternative has/can/should) (in Java it's convention to use camelCase)
so rename your boolean add_to_backstack
into boolean isAddedToBackstack
rather (btw. in java it's convention to use camelCase)
2) comments
remove commented code!
3) javadoc
you provide some javadoc on the addFragment
methode which is very crude - get things done and finish it!
the same applies to replaceFragment
.
Thank you for your review. I thought JavaDocs should be provided for all the methods defined for description purposes. So I added them. Guess I was wrong. Thanks again.
– sdb1995
21 hours ago
add a comment |
up vote
0
down vote
your code looks very good, there are only minor issues:
1) naming
since you don't create any instances of FragmentsManager
you should consider to rename it to FragmentsUtility
. An Utility class provides methods to help you with your code while a Manager is an instance that does the work for you.
boolean should beginn with prefix is (or rarely as an alternative has/can/should) (in Java it's convention to use camelCase)
so rename your boolean add_to_backstack
into boolean isAddedToBackstack
rather (btw. in java it's convention to use camelCase)
2) comments
remove commented code!
3) javadoc
you provide some javadoc on the addFragment
methode which is very crude - get things done and finish it!
the same applies to replaceFragment
.
Thank you for your review. I thought JavaDocs should be provided for all the methods defined for description purposes. So I added them. Guess I was wrong. Thanks again.
– sdb1995
21 hours ago
add a comment |
up vote
0
down vote
up vote
0
down vote
your code looks very good, there are only minor issues:
1) naming
since you don't create any instances of FragmentsManager
you should consider to rename it to FragmentsUtility
. An Utility class provides methods to help you with your code while a Manager is an instance that does the work for you.
boolean should beginn with prefix is (or rarely as an alternative has/can/should) (in Java it's convention to use camelCase)
so rename your boolean add_to_backstack
into boolean isAddedToBackstack
rather (btw. in java it's convention to use camelCase)
2) comments
remove commented code!
3) javadoc
you provide some javadoc on the addFragment
methode which is very crude - get things done and finish it!
the same applies to replaceFragment
.
your code looks very good, there are only minor issues:
1) naming
since you don't create any instances of FragmentsManager
you should consider to rename it to FragmentsUtility
. An Utility class provides methods to help you with your code while a Manager is an instance that does the work for you.
boolean should beginn with prefix is (or rarely as an alternative has/can/should) (in Java it's convention to use camelCase)
so rename your boolean add_to_backstack
into boolean isAddedToBackstack
rather (btw. in java it's convention to use camelCase)
2) comments
remove commented code!
3) javadoc
you provide some javadoc on the addFragment
methode which is very crude - get things done and finish it!
the same applies to replaceFragment
.
edited yesterday
answered yesterday


Martin Frank
547315
547315
Thank you for your review. I thought JavaDocs should be provided for all the methods defined for description purposes. So I added them. Guess I was wrong. Thanks again.
– sdb1995
21 hours ago
add a comment |
Thank you for your review. I thought JavaDocs should be provided for all the methods defined for description purposes. So I added them. Guess I was wrong. Thanks again.
– sdb1995
21 hours ago
Thank you for your review. I thought JavaDocs should be provided for all the methods defined for description purposes. So I added them. Guess I was wrong. Thanks again.
– sdb1995
21 hours ago
Thank you for your review. I thought JavaDocs should be provided for all the methods defined for description purposes. So I added them. Guess I was wrong. Thanks again.
– sdb1995
21 hours ago
add a comment |
sdb1995 is a new contributor. Be nice, and check out our Code of Conduct.
sdb1995 is a new contributor. Be nice, and check out our Code of Conduct.
sdb1995 is a new contributor. Be nice, and check out our Code of Conduct.
sdb1995 is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f208219%2fhelper-class-for-fragments-management%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IbGW6pQJlDZs91t6nNnSaPR1FGvHoKcKa aaHE5OzYAZUNNZOswTv1zLMC7wK0aDOpvMtKElLdr u5LnOTuVuWaFTBlsLn8M7j